Create your bot
Basics - Essentials to build a bot
Variables in Landbot - Getting Started
Landbot System Variables
Builder's keyboard shortcuts and mechanics! π
Builder Interface
Supported Browsers
Guidelines π 5 best practices for chatbot design in Landbot π€
How to "debug" (troubleshoot) your bot's flow to spot possible errors (for non coders)
Build a bot
Blocks and flow operations
AB Test block (randomize flow)
Buttons block
Buttons block
Reply Buttons block (WhatsApp)
Any of the above Output
How to open a new URL in another tab (window)
How to set up Multiple Choice questions
Code Block (Javascript)
Conditions block
Conditions block
Conditions block II (with Dates, Usage and Agents variables)
How to ask a question based on a variable not being set (empty URL params)
Flow operations
Global Keywords π
Keyword Jump
Keyword Options π Assign keywords to buttons (WhatsApp and Facebook)
Lead Scoring block
Persistent Menu
Jump To block
Formulas block
Get started with the Formulas block
Formulas Blocks Dashboard
Formulas - Regex
Formulas - Date
Formulas - String
Formulas - Logical
Formulas - Math
Formulas - Object
Formulas - Comparison
Formulas - Array
Goals block
Goodbye block
Media Block
Multi-Question block (Create a Form)
Note block
Question blocks
Question: Address block
Question: Autocomplete block
Question: Date block
Question: Email block
Question: File block
Question: Name block
Question: Number block
Question: Phone block
Question: Picture Choice block
Question: Rating block
Question: Scale block
Question: Text block
Question: URL block
Question: Yes/No block
Send a Message block
Send an Email block
Set Variable block
Starting Point
Business Hours block
List Buttons Block (WhatsApp)
Collect Intent block
Bricks
Bot Settings
Bot General Settings
Second Visit Settings
Custom System Messages
Hidden Fields (Get params / UTMs from url and use it as variables)
Landbot native SEO & Tracking tools
Typing Emulation (Message Delay)
Landbot in Wix
Chats - Livechat and Human Takeover
Design (bots for Web)
Share & Embed
Account Settings and Billing
Billing
Account Settings
Account Settings
Account Homepage
Dashboard
Roles & Permissions for Teammates
Agent Status and Log out
Common reasons for not receiving account activation email
Privacy and Security
Landbot's Privacy & Security Policies
Compliance with the GDPR - Β General Data Protection Regulation
Landbot's Abuse Policy - How to Report an Abusive Use of Landbot
Teammates - Add extra agents (seats) to your Account
Notifications section
Integrations with Landbot
ActiveCampaign
Airtable
Airtable integration block
Get data filtered from Airtable with a Brick- Shop example
20 different ways to GET and filter data from Airtable
How to add/update different field types in Airtable (POST, PATCH & PUT)
How to Create, Update, Retrieve and Delete records in Airtable (POST, PATCH, GET & DELETE)
Get more than 100 items from Airtable
Insert Multiple Records to Airtable with a Loop
How to Get an Airtable Token
Advanced filters formulas Airtable block
Update Multiple Records in Airtable Using a Loop
Calendly
Carrd
DeepL
Dialogflow
Dialogflow & Landbot course
Dialogflow & Landbot intro: What is NLP, Dialogflow and what can you do with it?
Dialogflow & Landbot lesson 1: Create your first agent and intent in Dialogflow
Dialogflow & Landbot lesson 2: Get the JSON Key
Dialogflow & Landbot lesson 3: Setting up of Dialogflow in Landbot
Dialogflow & Landbot lesson 4: Training phrases and responses for a FAQ
Dialogflow & Landbot lesson 5: Entities and Landbot variables
Dialogflow & Landbot lesson 6: Redirect user depending on Dialogflow response parameters (intent, entities and more)
Learn more about Dialogflow - Courses and communities
Integrations > Dialogflow Block
How to extract parameters from Dialogflow response with Formulas
Dialogflow Integration Dashboard
Dialogflow in Unsupported Languages (& Multilingual)
Dialogflow - How to get JSON Key
External API REST
Dynamic Data (Arrays)
Webhook Block Dashboard
Webhook block (External API REST)
How to TEST your Http Request (Webhook block)
Facebook Pixel
Google Analytics
Google Adwords
Google Maps
Google Maps API Key for Address block
Extract Data With Google Maps Geocoding API
Calculate Distances With Google Maps API
Google Sheets
Google Sheets (Insert, Update and Get data)
How to use Google Sheets to create a simple verification system for returning visitors
How to Upload Document & store link
How to give unique Coupon Codes (with Google Spreadsheets)
Google Sheets Integration Dashboard
How to insert a new row with data and formulas in Google Sheets
How to use Google Spreadsheet as a Content Management System for your bot
Google Tag Manager
How Track Google Analytics Events in Landbot with Google Tag Manager (GTM)
Landbot in your web with Google Tag Manager
HubSpot
IFTTT
Integromat
Connecting MySQL with Make.com (formerly Integromat)
How to upload a file to Google Drive using Make.com (formerly Integromat)
Send WhatsApp Message Template from Make (ex Integromat)
Make.com (formerly Integromat) trigger
How to send an email through Sendinblue by Make.com (formerly Integromat)
Get Opt-ins (Subscribers) from Facebook Leads using Make (ex Integromat)
How to extract data from an external source with Make.com and use it in Landbot
Integrately
Intercom
Vonage
Mailchimp
OCR
OpenAI
Create a JSON format response from OpenAI in WhatsApp
Connect OpenAI Assistant with Landbot
How to build a FAQ chatbot with GPT-3
GPT-4 in Landbot
Fine-Tune GPT3 with Postman
OpenAI
Prompt Engineering for GPT-3
Build a Customer Service Bot with ChatGPT and Extract Information
Pabbly
Paragon
PDF Monkey
Pipedream
Salesforce
Segment
Sendgrid
Slack
Stripe
Squarespace
Sharetribe
Shopify
Store Locator Widgets
How to display the near location of your store in WhatsApp (with Store Locator Widgets)
How to display the near location of your store for Web bots (with Store Locator Widgets)
Webflow
Wordpress
Xano
Zendesk
Zapier
Zapier block
Zapier Integration Dashboard
How to insert a row to Google Spreadsheet by Zapier
How to generate a document with PDFMonkey by Zapier
Send WhatsApp Message Templates from Zapier
How to send an email with Gmail by Zapier
Get Opt-ins (Subscribers) from Facebook Leads using Zapier
How to extract data from an external source with Zapier and use it in Landbot
Zapier trigger
How to complete a digital signature flow by Zapier
Make a survey with Landbot and display the results in a Notion table using Zapier
Trigger Automation block
WhatsApp Channel
Getting started with the WhatsApp Integration
WhatsApp Testing
Facebook Business Verification - Best Practices π¬π§
Adding a WhatsApp number to your account
WhatsApp Channel Settings
Growth Tools for WhatsApp
Opt-in, Templates and Campaigns
Parent Bot/Linked Bot - Add a main bot to your WhatsApp number
How to get Opt-ins (Subscribers) for your WhatsApp π
WhatsApp Campaigns π
WhatsApp's Message Templates
Send WhatsApp Messages with Landbot API
Audience block
Recognise the users input when sending a Message Template with buttons
Audiences
Create Opt-ins (Subscribers) using Landbot API
Opt-In block for WhatsApp π
Opt-in Check Block
WhatsApp Bot Creation - Best Practices and compatibility guide
Build a WhatsApp Bot - Best Practices and User guide
Build a WhatsApp Bot - Best Practices for Developers
WhatsApp bots - Feature Compatibility Guide
Types of Content and Media you can use in WhatsApp πΌ
WhatsApp - How to direct a user through a different bot flow on their second visit
WhatsApp - Get user out of error message loop
Javascript in WhatsApp
How to calculate the number of days between two selected dates (WhatsApp)
Creating a Loop in WhatsApp
AI in WhatsApp
Send Automated Message Templates based on Dates
Different ways to format numbers with JS (WhatsApp)
Notify Teammates of Chat via WhatsApp
Creating a Simple Cart in WhatsApp
1. WhatsApp Article Directory
2. WhatsApp Integration FAQ's
WhatsApp Number Deletion (WA Channel management)
Customize and embed your WhatsApp Widget
Meta processes guide: FBM verification, Official Business Account (OBA) requests, Appeals
Additional Number integration: Limitations and Requirements (Number integration)
Send a Message Template from the Builder
Existing WhatsApp Number Migration (Migration)
Other Channels - Messenger and APIChat
Facebook Messenger
The Facebook Messenger Ultimate Guide
Types of content you can use in Messenger bots πΌ
How to Preview a Messenger bot
Facebook Ad connected to Messenger Bot
API Chat (for Developers)
How to create an API chat bot and set up the Hook Url
Building a Slack APP Bot with Landbot APIChat and Node JS
Build a Telegram Bot with Landbot APIChat and Node JS
Duplicating bots
AI in Landbot
Metrics and Data Management
Metrics Section
How to export the data from your bots
Export data: How to open a CSV file
Bot's Analyze Section
For Developers & Designers
CSS and Design Customizations
Dynamic Customizations
How to change Avatar dynamically (Landbot v3)
Dynamically Change a Bot's Background
Create a Checkbox in the Form Block
Dynamically Change Any Style
Embed Customizations
How to "send" a user to a specific point in the flow with Javascript and with the API
Modifying Embed Size
Load script and display bot on click button
How to launch a Landbot by clicking a button
Open LiveChat bot as soon as page loads (3 ways)
Change Landbot custom CSS dynamically from parent page onload
CSS Customization Examples
CSS Customization Examples: "Back to School" Theme
CSS Customization Examples: Call To Action: WhatsApp
CSS Customization Examples: "Translucid"
CSS Customization Examples: "Minimalist" Theme
CSS Customization Examples: Carrd Embed Beginner
Make Persistent Menu Appear During the Flow
CSS Customization Examples: Video Bubble
CSS Customization Examples: CV Template
Design Customizations
How to use Google Fonts in Landbot
CSS for Typewriter Effect
Proactive Message Customizations with Javascript and CSS
Landbot v3 - Web CSS - RTL
Hide time stamp (under avatar)
How to remove Landbot branding
HTML Template for Emails
Widget/Bubble Customizations with Javascript and CSS
Detect if a visitor is on Mobile/Tablet or Desktop
Customized display in the bot
How to display an HTML Table and a List in Landbot v3 web
Progress Bar Workaround
Add a Chart (with Chart JS library) in your Landbot
How to embed a .gif file inside a message
Different ways to embed Videos in Landbot
Pop up modal to embed third party elements
Display video and hide button to continue until video has ended
Components CSS Library
Components CSS Library Index
Background Class CSS
Identify Blocks CSS
Buttons Class CSS
Header Class CSS
Media Class CSS
Message Bubble Class CSS
Miscellaneous Classes CSS
Recommended Image Sizes
Dynamic Data CSS
Form Block CSS
Multi Questions CSS
Get started guide for CSS Design in Landbot
Landbot APIs
APIs
Create an SMS bot with ClickSend and API Chatbot
reply from Slack: How to create an integration to allow agents reply WhatsApp users from Slack (with Node JS)
MessageHooks - Landbot Webhooks
Javascript and SDK
Workarounds and How To's
Building Tips & How to's
How to build an event registration Landbot (to be used in one screen by many attendees) (web only)
How to redirect user to another url in your site with Livechat open to continue conversation
How to let user select a time of booking (with a minimum 45 minutes notice)
Get the array's index of the user selection and extract information from array
How to redirect visitors to a URL (web only)
Embed Google Maps
How to add a Click-to-Call/Email/WhatsApp button
How to disable a bot
Airtable usecase: Create an event registration bot with limited availability
How to calculate the number of days between two selected dates
Reservation bot with Airtable
Set a timer to get the time spent during the flow
Workarounds & Codes
How to Detect Visitors Browser
Conditional Welcome (Non-embedded bots)
Conditional Welcome: Direct the Flow of Your Bot Based on External Parameters
Two-Step Email Verification
Detect if bot was opened
Different ways to format numbers with JS
Trigger Event if User Abandons Chat
Calculate Distances in WhatsApp
Customized Behavior in Mobile Browsers
Trigger Event if User Abandons Chat Using AWS
Launch Bot On Exit Intent
Display Bot During Business Hours Only (Livechat & Popup)
Delete Customer Data in Flow
Cookie consent banner (full page / full page embed)
Add Captcha Verification (Non-Embedded Bots)
Launching a bot depending on browser language
Trigger a Global Keyword with JS (web v3)
Create a Scale out of Buttons - Workaround
How to set up questions with a countdown
How to pass WordPress logged in user data to Landbot
Create Dynamic Shopping Cart with JS and CSS
Set the flow depending on the url path (for embedded landbots)
Generate a URL that has variables from user answers
Resume flow based on external process with Landbot API (Request, Set, Go)
Creating Masks for User Input (2 examples)
Popup on Exit Intent
Open / Close a Web bot (embedded)
More Topics
- All Categories
- Workarounds and How To's
- Workarounds & Codes
- Creating Masks for User Input (2 examples)
Creating Masks for User Input (2 examples)
Updated
by Cesar Banchio
In this article we are going to provide 2 examples on applying masks to user's input, when we want them formatted a certain way.
Date Format
Add a code block before the Text Block with the following snippet:
const wrapper = () => {
var input = this.window.document.querySelector(".InputText input");
input.maxLength=10;
var dateInputMask = (elm) => {
elm.addEventListener('keypress', function(e) {
if(e.keyCode < 47 || e.keyCode > 57) {
e.preventDefault();
}
var len = elm.value.length;
// If we're at a particular place, let the user type the slash
// i.e., 12/12/1212
if(len !== 1 || len !== 3) {
if(e.keyCode == 47) {
e.preventDefault();
}
}
// If they don't add the slash, do it for them...
if(len === 2) {
elm.value += '/';
}
// If they don't add the slash, do it for them...
if(len === 5) {
elm.value += '/';
}
});
};
dateInputMask(input);
}
setTimeout(wrapper, 1500)
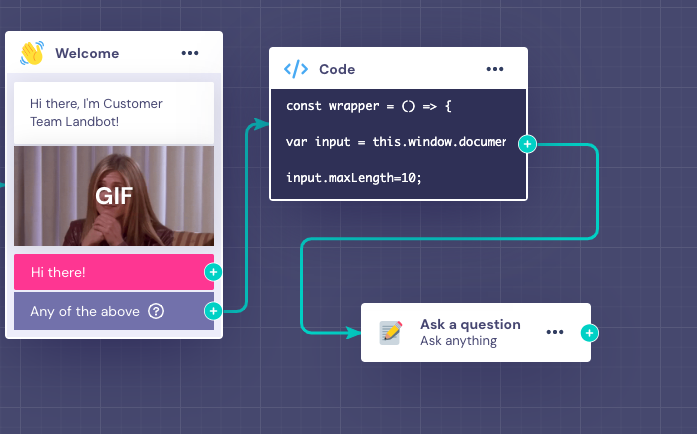
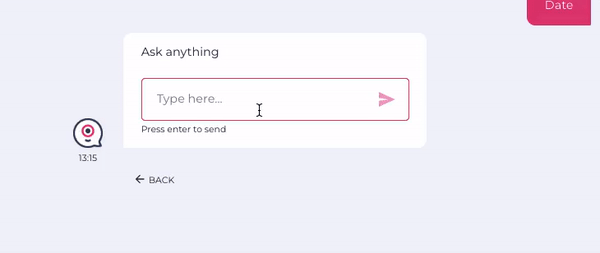
Number Format
Add a code block before the Text Block with the following snippet:
String.prototype.reverse = function(){
return this.split('').reverse().join('');
};
const handler = function(evento) {
let num = evento.target;
var tecla = (!evento) ? window.event.keyCode : evento.which;
var valor = num.value.replace(/[^\d]+/gi,'').reverse();
var resultado = "";
var mascara = "##.###.###,#".reverse();
for (var x=0, y=0; x<mascara.length && y<valor.length;) {
if (mascara.charAt(x) != '#') {
resultado += mascara.charAt(x);
x++;
} else {
resultado += valor.charAt(y);
y++;
x++;
}
}
num.value = resultado.reverse();
}
const selection = () => {
const inp = _landbot.document.querySelector(".InputText");
inp.addEventListener("keypress", handler);
}
setTimeout(selection, 1000);
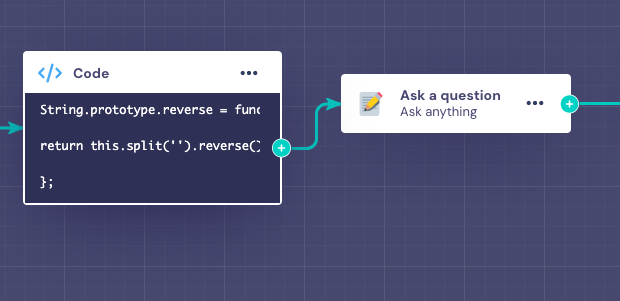
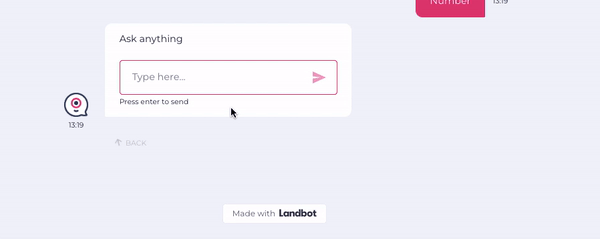