Build a bot
Getting started
Basics and builder
Fields in Landbot - Getting Started
Builder's keyboard shortcuts and mechanics! 🏗
Builder Interface
How to "debug" (troubleshoot) your bot's flow to spot possible errors (for non coders)
Starting Point
How to disable a bot
Bricks
Bot's Settings
Bot General Settings
Second Visit Settings
Custom System Messages
Hidden Fields (Get params / UTMs from url and use it as variables)
Landbot native SEO & Tracking tools
Typing Emulation (Message Delay)
Build it for me!
Getting started - build a bot
Messages, Questions and Logic & Technical blocks
Messages
Media Block
Media block
How to display images with a variable URL source
How to embed a .gif file inside a message
Different ways to embed Videos in Landbot
Display video and hide button to continue until video has ended
Send a Message block - Simple Message
Goodbye block
Question blocks
Date Block
How to avoid visitors selecting specific days of the week
Question: Date block
How to calculate the number of days between two selected dates
Email Block
Form Block
Scale Block
Buttons block
How to set up Multiple Choice questions
Multi-Question block
Question: Address block
Question: Autocomplete block
Question: File block
Ask for a name
Question: Number block
Question: Phone block
Question: Picture Choice block
Question: Rating block
Question: Text block
Question: URL block
Question: Yes/No block
Logic & Technical blocks
Code Blocks
Dynamic Data
Dynamic Data (Arrays)
Get the array's index of the user selection and extract information from array
Formulas
How to Perform Basic Calculations
Get started with the Formulas block
Formulas Blocks Dashboard
Formulas - Regex
Formulas - Date
Formulas - String
Formulas - Logical
Formulas - Math
Formulas - Object
Formulas - Comparison
Formulas - Array
Persistent Menu
Trigger Automation
Webhook
Webhook Block Dashboard
Webhook block (External API REST)
How to TEST your Http Request (Webhook block)
Landbot System Variables
Set Variable
Any of the above Output
Global Keywords 🌍
Keyword Jump
Lead Scoring block
Jump To block
AB Test
Conditions block
Conditions block II (with Dates, Usage and Agents variables)
Close Chat block
How to ask a question based on a variable not being set (empty URL params)
Business Hours block
Custom Goals
Note block
Share & Embed
Redirect Users
How to open a new URL in another tab (window)
How to redirect visitors to a URL (web only)
Conditional Welcome (Non-embedded bots)
How to add a Click-to-Call/Email/WhatsApp button
Redirect User Based on Language Input (DeepL)
Generate a URL that has variables from user answers
Popup on Exit Intent
Share
Customized Embed Actions
How to redirect user to another url in your site with Livechat open to continue conversation
How to Detect Visitors Browser
Customize and embed your WhatsApp Widget
Modifying Embed Size
Conditional Welcome: Direct the Flow of Your Bot Based on External Parameters
Detect if bot was opened
Customized Behavior in Mobile Browsers
Load script and display bot on click button
Launch Bot On Exit Intent
Display Bot During Business Hours Only (Livechat & Popup)
Open / Close a Web bot (embedded)
Launching a bot depending on browser language
How to pass WordPress logged in user data to Landbot
Set the flow depending on the url path (for embedded landbots)
How to launch a Landbot by clicking a button
Open LiveChat bot as soon as page loads
Detect if a visitor is on Mobile/Tablet or Desktop
Embed
Embed the bot into your Website
Embed Landbot in an iframe
Landbot in Wix
Landbot in your web with Google Tag Manager
Landbot in Webflow
Embed in Sharetribe
Landbot in Shopify
Embedding Landbot in Carrd
Landbot in Wordpress
Landbot in Squarespace
Customizing the Proactive Message
Design section (web bots)
Verification & Security
Account Settings and Billing
Billing
Account Settings
Account Settings
Account Homepage
Dashboard
Common reasons for not receiving account activation email
Privacy and Security
Landbot's Privacy & Security Policies
Compliance with the GDPR - General Data Protection Regulation
Landbot's Abuse Policy - How to Report an Abusive Use of Landbot
Teammates
Agent Status and Log out
Roles & Permissions for Teammates
Teammates - Add extra agents (seats) to your Account
Notifications section
Startup Discounts
NGOs and Educational Organizations Discount
Human Takeover & Inbox
Integrations with Landbot
Native Integrations
Airtable
Airtable integration block
Get data filtered from Airtable with a Brick- Shop example
20 different ways to GET and filter data from Airtable
How to add/update different field types in Airtable (POST, PATCH & PUT)
How to Create, Update, Retrieve and Delete records in Airtable (POST, PATCH, GET & DELETE)
Get more than 100 items from Airtable
Insert Multiple Records to Airtable with a Loop
How to Get an Airtable Token
Advanced filters formulas Airtable block
Airtable usecase: Create an event registration bot with limited availability
Update Multiple Records in Airtable Using a Loop
Reservation bot with Airtable
Calendly
Dialogflow
Dialogflow & Landbot course
Dialogflow & Landbot intro: What is NLP, Dialogflow and what can you do with it?
Dialogflow & Landbot lesson 1: Create your first agent and intent in Dialogflow
Dialogflow & Landbot lesson 2: Get the JSON Key
Dialogflow & Landbot lesson 3: Setting up of Dialogflow in Landbot
Dialogflow & Landbot lesson 4: Training phrases and responses for a FAQ
Dialogflow & Landbot lesson 5: Entities and Landbot variables
Dialogflow & Landbot lesson 6: Redirect user depending on Dialogflow response parameters (intent, entities and more)
Learn more about Dialogflow - Courses and communities
Integrations > Dialogflow Block
How to extract parameters from Dialogflow response with Formulas
Dialogflow Integration Dashboard
Dialogflow in Unsupported Languages (& Multilingual)
Dialogflow - How to get JSON Key
Google Sheets
Google Sheets (Insert, Update and Get data)
How to use Google Sheets to create a simple verification system for returning visitors
How to Upload Document & store link
How to give unique Coupon Codes (with Google Spreadsheets)
Google Sheets Integration Dashboard
How to insert a new row with data and formulas in Google Sheets
How to use Google Spreadsheet as a Content Management System for your bot
Hubspot
MailChimp
Salesforce
Segment
SendGrid
Slack
Stripe
Zapier
Zapier block
Zapier Integration Dashboard
How to insert a row to Google Spreadsheet by Zapier
How to generate a document with PDFMonkey by Zapier
Send WhatsApp Message Templates from Zapier
How to send an email with Gmail by Zapier
Get Opt-ins (Subscribers) from Facebook Leads using Zapier
How to extract data from an external source with Zapier and use it in Landbot
Zapier trigger
How to complete a digital signature flow by Zapier
Make a survey with Landbot and display the results in a Notion table using Zapier
Zendesk
Non-Native Integrations
ActiveCampaign
Google Calendar
Google Fonts
Google Maps
Embed Google Maps
Google Maps API Key for Address block
Extract Data With Google Maps Geocoding API
Calculate Distances With Google Maps API
Google Meet
IFTTT
Integrately
Intercom
Make
Connecting MySQL with Make.com (formerly Integromat)
How to upload a file to Google Drive using Make.com (formerly Integromat)
Send WhatsApp Message Template from Make (ex Integromat)
Make Integration With Trigger Automation Block
How to send an email through Sendinblue by Make.com (formerly Integromat)
Get Opt-ins (Subscribers) from Facebook Leads using Make (ex Integromat)
How to extract data from an external source with Make.com and use it in Landbot
OCR
Pabbly
Paragon
Pipedream
PDF Monkey
Store Locator Widgets
Xano
Zendesk
WhatsApp Channel
Getting started!
WhatsApp Testing
Build a WhatsApp Bot - Best Practices and User guide
Build a WhatsApp Bot - Best Practices for Developers
Types of Content and Media you can use in WhatsApp 🖼
1. WhatsApp Article Directory
2. WhatsApp Integration FAQ's
Adding & Managing your WhatsApp Channel
Facebook Business Verification - Best Practices 🇬🇧
WhatsApp Number Deletion (WA Channel management)
Adding a WhatsApp number to your account
WhatsApp’s Messaging Policy: New Accepted Industry verticals
Meta processes guide: FBM verification, Official Business Account (OBA) requests, Appeals
Additional Number integration: Limitations and Requirements (Number integration)
Existing WhatsApp Number Migration
Key Insights for Migrating to WhatsApp Business API Cloud
How to's, Compatibility & Workarounds
WhatsApp bots - Feature Compatibility Guide
WhatsApp - How to direct a user through a different bot flow on their second visit
WhatsApp - Get user out of error message loop
Getting Subscribers: Opt-in, Contacts
How to get Opt-ins (Subscribers) for your WhatsApp 🚀
WhatsApp Quality - Best Practices
Opt-In block for WhatsApp 🚀
Opt-in Check Block
WhatsApp Channel Settings
Parent Bot/Linked Bot - Add a main bot to your WhatsApp number
WhatsApp Channel Panel (Settings)
Growth Tools for WhatsApp
Duplicating bots
Messaging and contacting your users
WhatsApp Campaigns 💌
WhatsApp's Message Templates
Audience block
WhatsApp Marketing Playbook: Best Practices for Leadgen
Audiences
WhatsApp for Devs
How to calculate the number of days between two selected dates (WhatsApp)
Creating a Loop in WhatsApp
Recognise the users input when sending a Message Template with buttons
Trigger Event if User Abandons Chat
Calculate Distances in WhatsApp
Send Automated Message Templates based on Dates
Trigger Event if User Abandons Chat Using AWS
reply from Slack: How to create an integration to allow agents reply WhatsApp users from Slack (with Node JS)
Set Up a Delay Timer in Bot
Notify Teammates of Chat via WhatsApp
Native blocks for WhatsApp
Other Channels - Messenger and APIChat
Facebook Messenger
The Facebook Messenger Ultimate Guide
Types of content you can use in Messenger bots 🖼
How to Preview a Messenger bot
Facebook Ad connected to Messenger Bot
API Chat (for Developers)
AI in Landbot
Non-native AI Features
Create a JSON format response from OpenAI in WhatsApp
Connect OpenAI Assistant with Landbot
AI in WhatsApp
How to build a FAQ chatbot with GPT-3
GPT-4 in Landbot
OpenAI
Prompt Engineering for GPT-3
Build a Customer Service Bot with ChatGPT and Extract Information
Native AI Features
Metrics and Data Management
Metrics Section
How to export the data from your bots
Export data: How to open a CSV file
Bot's Analyze Section
For Developers & Designers
JavaScript and CSS
CSS and Design Customizations
Design Customizations
Advanced (Custom CSS & Custom JS)
Components CSS Library Index
Background Class CSS
Identify Blocks CSS
Buttons Class CSS
Header Class CSS
Media Class CSS
Message Bubble Class CSS
Miscellaneous Classes CSS
CSS Customization Examples: "Back to School" Theme
Get started guide for CSS Design in Landbot
CSS Customization Examples: Call To Action: WhatsApp
CSS Examples: Lead Gen
CSS Customization Examples: "Translucid"
CSS Customization Examples: "Minimalist" Theme
Dynamic Data CSS
Form Block CSS
CSS for Typewriter Effect
CSS Customization Examples: Carrd Embed Beginner
Dynamically Change a Bot's Background
Proactive Message Customizations with Javascript and CSS
Landbot v3 - Web CSS - RTL
Multi Questions CSS
CSS Customization Examples: Video Bubble
Dynamically Change Any Style
CSS Customization Examples: CV Template
Change Landbot custom CSS dynamically from parent page onload
Widget/Bubble Customizations with Javascript and CSS
JavaScript
How to change Avatar dynamically (Landbot v3)
Javascript in WhatsApp
Javascript in Landbot v3
Different ways to format numbers with JS
How to display an HTML Table and a List in Landbot v3 web
Trigger a Global Keyword with JS (web v3)
Create Dynamic Shopping Cart with JS and CSS
Add a Chart (with Chart JS library) in your Landbot
Different ways to format numbers with JS (WhatsApp)
Pop up modal to embed third party elements
Landbot API
Send WhatsApp Messages with Landbot API
How to "send" a user to a specific point in the flow with Javascript and with the API
APIs
Create Contacts (Opt-ins) using Landbot API
MessageHooks - Landbot Webhooks
Resume flow based on external process with Landbot API (Request, Set, Go)
Tracking
Google Analytics - Track Events (Not embedded)
Google Analytics - Track Events (Embedded)
Meta Pixel - Track Events (only Embedded)
How Track Google Analytics Events in Landbot with Google Tag Manager (GTM)
Google Adwords - How to track Google Adwords in Landbot
Set a timer to get the time spent during the flow
Workarounds and How To's
Workflows
How to build an event registration Landbot (to be used in one screen by many attendees) (web only)
How to let user select a time of booking (with a minimum 45 minutes notice)
Send Files Hosted in Landbot to Your Google Drive with Make
Two-Step Email Verification
Skip the Welcome Message
Fixing Web Bot Loading Issues for iOS Devices in Meta Campaigns with Disclaimers
Progress Bar Workaround
How to set up questions with a countdown
Creating a Simple Cart in WhatsApp
More Topics
Table of Contents
- All Categories
-
- Pop up modal to embed third party elements copy
Pop up modal to embed third party elements copy
Updated
by Pau Sanchez
Displaying html and scripts in Landbot sometimes can be limited by the size of the messages, and it's more useful to display 3rd party elements on top of the messages for better visualisation. Here we will show how to do it with a pop up modal:
In Landbot 3
Only 1 modal
- Here is how is going to be the builder flow:
- A welcome message;
- An Ask A Question block, with 1 button and the code that will trigger the modal pop up. The button will be used to continue the flow once the modal is closed
- A last message, just for the example
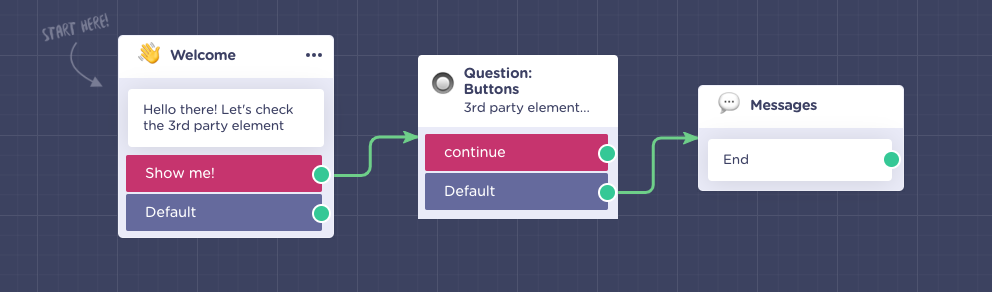
The Ask A Question block, is going to be used, to trigger an internal function once the user arrives to this point
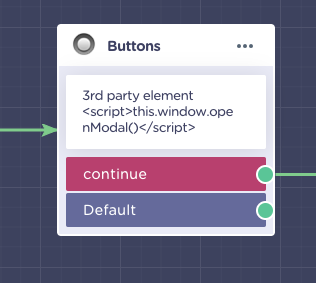
3rd party element <script>this.window.openModal()</script>
- Design / Advanced / Add CSS & ADD JS Section:
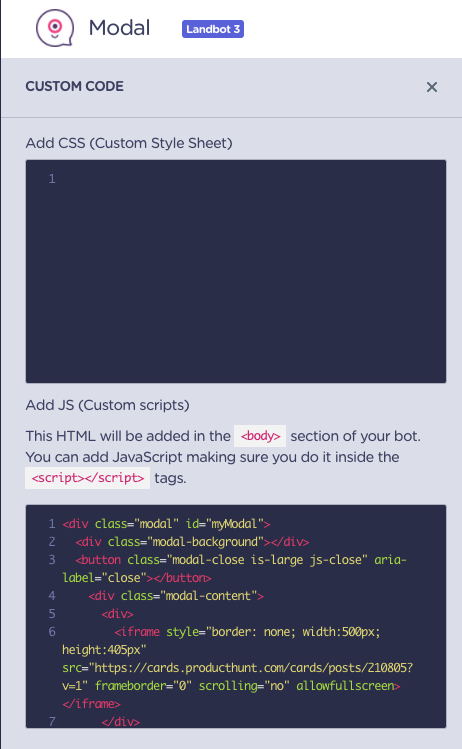
In the ADD JS section paste the code below:
<div class="modal" id="myModal">
<div class="modal-background"></div>
<button class="modal-close is-large js-close" aria-label="close"></button>
<div class="modal-content">
<div>
<iframe style="border: none; width:500px; height:405px" src="https://cards.producthunt.com/cards/posts/210805?v=1" frameborder="0" scrolling="no" allowfullscreen></iframe>
</div>
</div>
</div>
<script>
var _landbot = this;
this.onLoad(function() {
// Be careful with the function scope. `this` won't be available anymore as the widget instance
var modal = _landbot.document.getElementById('myModal');
// Open modal function
_landbot.window.openModal = function openModal() {
modal.classList.add('is-active');
}
// Close modal function
_landbot.window.closeModal = function closeModal() {
modal.classList.remove('is-active');
}
// Close event handler
_landbot.document.querySelectorAll('.js-close').forEach(function(button) {
button.addEventListener('click', function(event) {
_landbot.window.closeModal();
});
});
});
</script>
Here you can see an example:
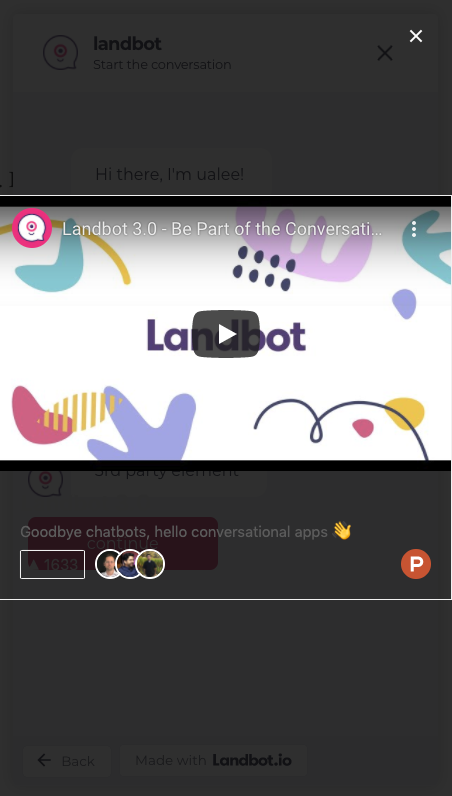
- To display your iframe, just need to replace this line:
<iframe style="border: none; width:500px; height:405px" src="https://cards.producthunt.com/cards/posts/210805?v=1" frameborder="0" scrolling="no" allowfullscreen></iframe>
Multiple modals different iframe urls
In case we want to display more than one modal but with different content, we should adapt the code accordingly.
In the builder we will add the following blocks to trigger the scripts:
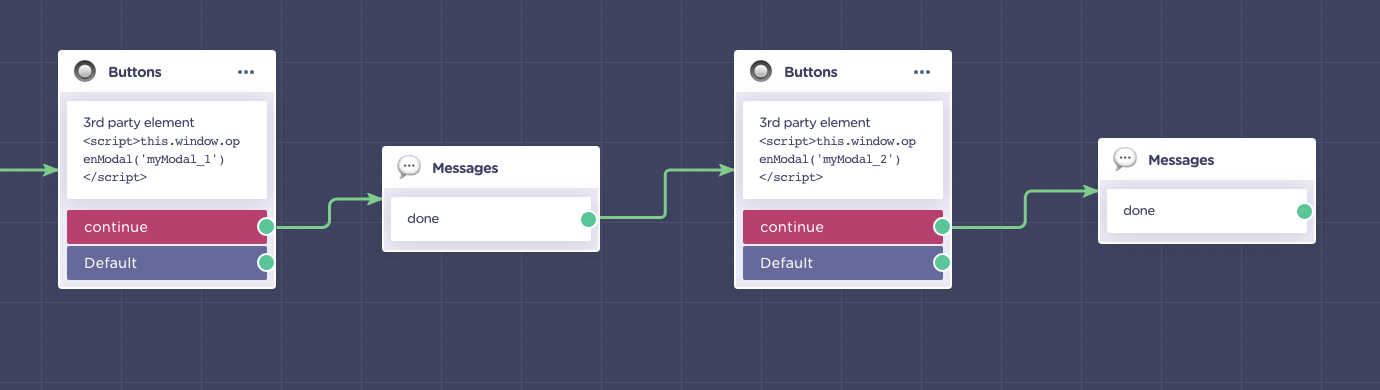
As you can notice we are using the same function, but with a different parameter, in the first case is "myModal_1" and in the second one is "myModal_2"
Then, using such name, we will add different names to the divs that contain our specific iframes:
<div class="modal" id="myModal_1">
<div class="modal-background"></div>
<button class="modal-close is-large js-close" aria-label="close"></button>
<div class="modal-content">
<div>
<iframe style="border: none; width:500px; height:405px" src="https://cards.producthunt.com/cards/posts/210805?v=1" frameborder="0" scrolling="no" allowfullscreen></iframe>
</div>
</div>
</div>
<div class="modal" id="myModal_2">
<div class="modal-background"></div>
<button class="modal-close is-large js-close" aria-label="close"></button>
<div class="modal-content">
<div>
<iframe width="100%" height="166" scrolling="no" frameborder="no" src="https://w.soundcloud.com/player/?url=https%3A//api.soundcloud.com/tracks/256153253&color=%23ff5500&auto_play=false&hide_related=false&show_comments=true&show_user=true&show_reposts=false&show_teaser=true"></iframe>
</div>
</div>
</div>
<script>
var _landbot = this;
this.onLoad(function() {
var modal;
// Open modal function
_landbot.window.openModal = function openModal(modalId) {
modal = _landbot.document.getElementById(modalId);
modal.classList.add('is-active');
}
// Close modal function
_landbot.window.closeModal = function closeModal() {
modal.classList.remove('is-active');
}
// Close event handler
_landbot.document.querySelectorAll('.js-close').forEach(function(button) {
button.addEventListener('click', function(event) {
_landbot.window.closeModal();
});
});
});
</script>
Modal with dynamic urls
In case we want to display more than one modal but with different content, and at the same time with urls that change every time, here is how:
In the builder we will add the following blocks to trigger the scripts:
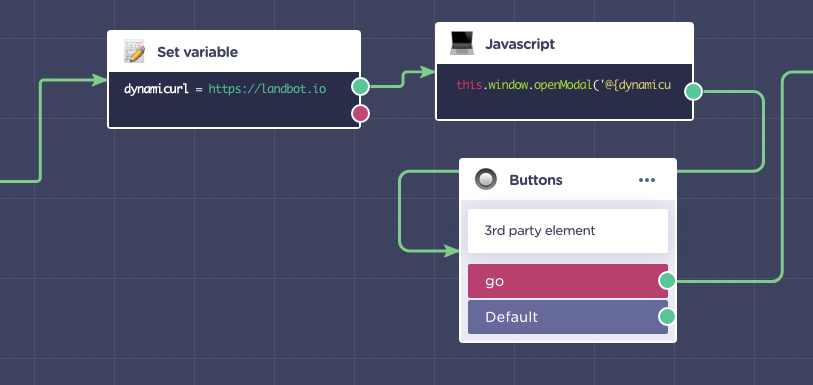
We use a code block to trigger the modal display:
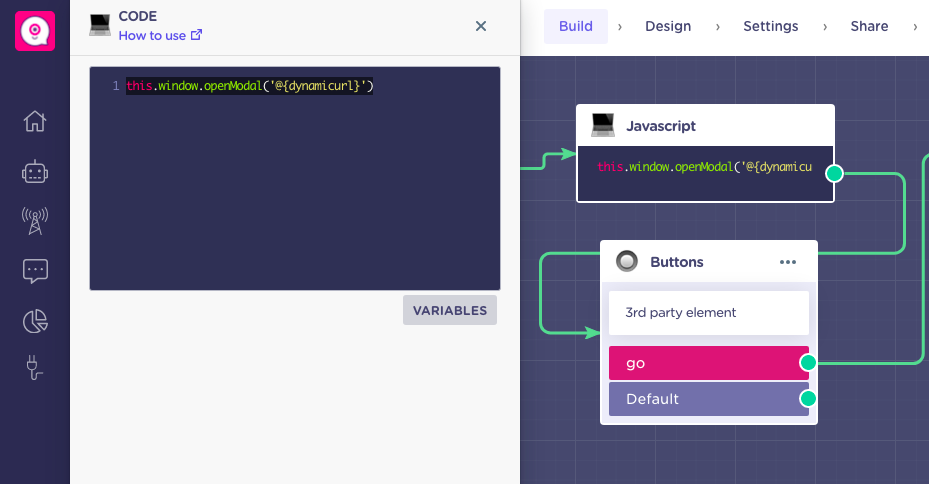
With this code:
this.window.openModal('@{dynamicurl}')
In this case @dynamicurl is a string variable, that will contain in it's value the url that will be displayed in the iframe
Now in the Design > Custom Code > Add JS section we will add the following code:
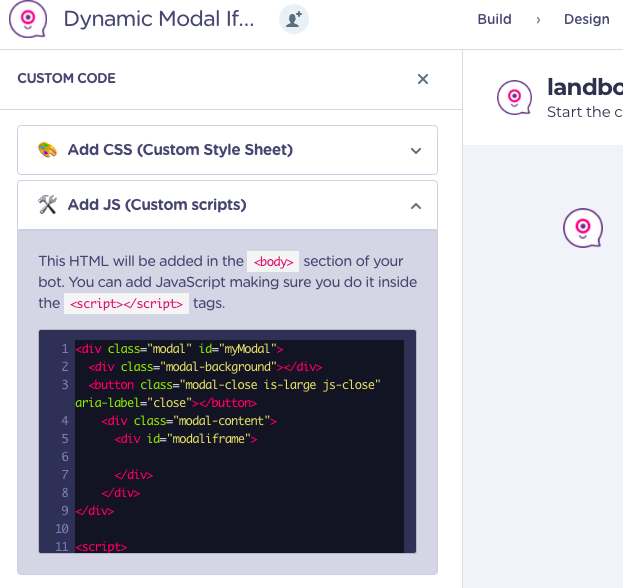
<div class="modal" id="myModal">
<div class="modal-background"></div>
<button class="modal-close is-large js-close" aria-label="close"></button>
<div class="modal-content">
<div id="modaliframe">
</div>
</div>
</div>
<script>
var _landbot = this;
this.onLoad(function() {
// Be careful with the function scope. `this` won't be available anymore as the widget instance
var modal = _landbot.document.getElementById('myModal');
// Open modal function
_landbot.window.openModal = function openModal(url) {
var modaliframe = _landbot.document.getElementById('modaliframe');
var ifrm = document.createElement("iframe");
ifrm.setAttribute('src', url);
ifrm.style.border = "none";
ifrm.style.width = "500px";
ifrm.style.height = "405px";
modaliframe.appendChild(ifrm);
modal.classList.add('is-active');
}
// Close modal function
_landbot.window.closeModal = function closeModal() {
var modaliframe = _landbot.document.getElementById('modaliframe');
modaliframe.innerHTML = "";
modal.classList.remove('is-active');
}
// Close event handler
_landbot.document.querySelectorAll('.js-close').forEach(function(button) {
button.addEventListener('click', function(event) {
_landbot.window.closeModal();
});
});
});
</script>
In Landbot 2
- Here is how is going to be the builder flow:
- A welcome message;
- An Ask A Question block, with 1 button and the code that will trigger the modal pop up. The button will be used to continue the flow once the modal is closed
- A last message, just for the example
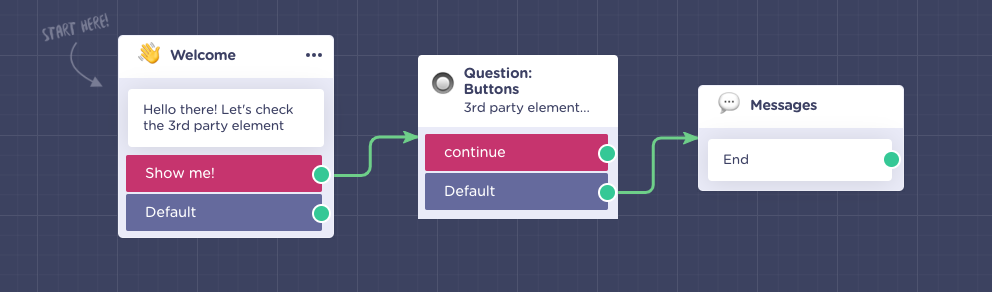
The Ask A Question block, is going to be used, to trigger an internal function once the user arrives to this point
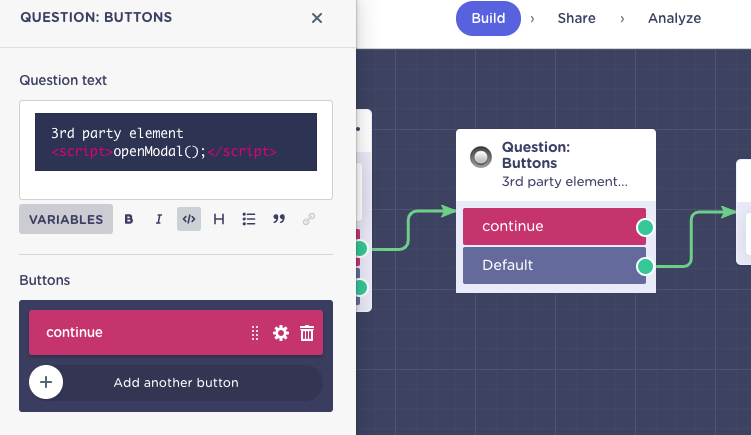
3rd party element <script>openModal();</script>
- Design / Advanced / Add CSS & ADD JS Section:
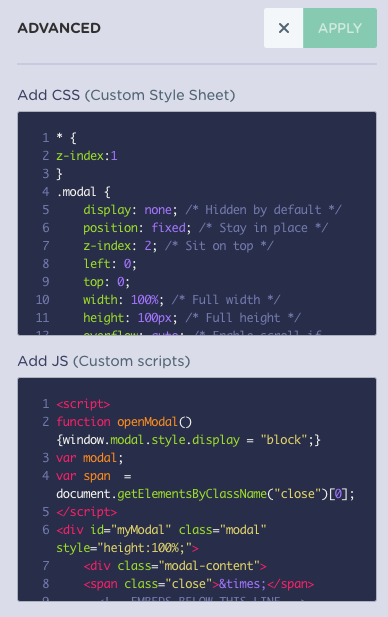
In the ADD CSS section paste the code below:
* {
z-index:1
}
.modal {
display: none; /* Hidden by default */
position: fixed; /* Stay in place */
z-index: 2; /* Sit on top */
left: 0;
top: 0;
width: 100%; /* Full width */
height: 100px; /* Full height */
overflow: auto; /* Enable scroll if needed */
background-color: rgb(0,0,0); /* Fallback color */
background-color: rgba(0,0,0,0.4); /* Black w/ opacity */
}
/* Modal Content/Box */
.modal-content {
background-color: #fefefe;
margin: 15% auto; /* 15% from the top and centered */
padding: 0px;
border: 1px solid #888;
width: 95%; /* Could be more or less, depending on screen size */
}
/* The Close Button */
.close {
color: #aaa;
float: right;
font-size: 28px;
font-weight: bold;
}
.close:hover,
.close:focus {
color: black;
text-decoration: none;
cursor: pointer;
}
In the ADD JS section paste the code below:
<script>
function openModal() {window.modal.style.display = "block";}
var modal;
var span = document.getElementsByClassName("close")[0];
</script>
<div id="myModal" class="modal" style="height:100%;">
<div class="modal-content">
<span class="close">×</span>
<div style="width:100%;height:0;padding-bottom:56%;position:relative;"><iframe src="https://giphy.com/embed/Rd6sn03ncIklmprvy6" width="100%" height="100%" style="position:absolute" frameBorder="0" class="giphy-embed" allowFullScreen></iframe></div><p><a href="https://giphy.com/gifs/mostexpensivest-viceland-most-expensivest-Rd6sn03ncIklmprvy6">via GIPHY</a></p>
</div>
</div>
<script>
var modal = document.getElementById('myModal');
var myIframe = document.getElementsByClassName('myIframe')
var span = document.getElementsByClassName("close")[0];
window.span.onclick = function() {
modal.style.display = "none";
}
window.onclick = function(event) {
if (event.target == modal) {
modal.style.display = "none";
}
}
</script>