Build a bot
Getting started
Basics and builder
Managing Data in Your Chatbot: A Guide to Using Fields
Builder's keyboard shortcuts and mechanics! 🏗
Builder Interface
How to "debug" (troubleshoot) your bot's flow to spot possible errors (for non coders)
Starting Point
How to disable a bot
Bricks
Bot's Settings
Bot General Settings
Second Visit Settings
Custom System Messages
Hidden Fields (Get params / UTMs from url and use it as variables)
Landbot native SEO & Tracking tools
Typing Emulation (Message Delay)
Build it for me!
Getting started - build a bot
Messages, Questions and Logic & Technical blocks
Messages
Media Block
Media block
How to display images with a variable URL source
How to embed a .gif file inside a message
Different ways to embed Videos in Landbot
Display video and hide button to continue until video has ended
Goodbye block
Send a Message block - Simple Message
Question blocks
Date Block
Scale Block
Buttons block
Ask for a Name block
Ask for an Email block
Ask a Question block
Question: Phone block
Forms block
How to set up Multiple Choice questions
Question: Address block
Question: Autocomplete block
Question: File block
Question: Number block
Question: Picture Choice block
Question: Rating block
Question: URL block
Question: Yes/No block
Logic & Technical blocks
Code Blocks
Dynamic Data
Dynamic Data (Arrays)
Get the array's index of the user selection and extract information from array
Formulas
How to Perform Basic Calculations
Get started with the Formulas block
Formulas Blocks Dashboard
Formulas - Regex
Formulas - Date
Formulas - String
Formulas - Logical
Formulas - Math
Formulas - Object
Formulas - Comparison
Formulas - Array
Persistent Menu
Trigger Automation
Webhook
Webhook Block Dashboard
Webhook Block: Simplify API Integrations in Landbot
How to TEST your Http Request (Webhook block)
Landbot System Fields
Set a Field block
Any of the above Output
Global Keywords 🌍
Keyword Jump
Lead Scoring block
Jump To block
AB Test
Conditions block
Conditions block II (with Dates, Usage and Agents variables)
Close Chat block
How to ask a question based on a variable not being set (empty URL params)
Business Hours block
Custom Goals
Note block
Share & Embed
Redirect Users
How to open a new URL in another tab (window)
How to redirect visitors to a URL (web only)
Conditional Welcome (Non-embedded bots)
How to add a Click-to-Call/Email/WhatsApp button
Redirect User Based on Language Input (DeepL)
Generate a URL that has variables from user answers
Popup on Exit Intent
Share
Customized Embed Actions
How to redirect user to another url in your site with Livechat open to continue conversation
How to Detect Visitors Browser
Customize and embed your WhatsApp Widget
Modifying Embed Size
Conditional Welcome: Direct the Flow of Your Bot Based on External Parameters
Detect if bot was opened
Customized Behavior in Mobile Browsers
Load script and display bot on click button
Launch Bot On Exit Intent
Display Bot During Business Hours Only (Livechat & Popup)
Open / Close a Web bot (embedded)
Launching a bot depending on browser language
How to pass WordPress logged in user data to Landbot
Set the flow depending on the url path (for embedded landbots)
How to launch a Landbot by clicking a button
Open LiveChat bot as soon as page loads
Detect if a visitor is on Mobile/Tablet or Desktop
Embed
Embed the bot into your Website
Embed Landbot in an iframe
Landbot in Wix
Landbot in your web with Google Tag Manager
Landbot in Webflow
Embed in Sharetribe
Landbot in Shopify
Embedding Landbot in Carrd
Landbot in Wordpress
Landbot in Squarespace
Customizing the Proactive Message
Design section (web bots)
Verification & Security
Account Settings and Billing
Billing
Account Settings
Account Settings
Account Homepage
Dashboard
Common reasons for not receiving account activation email
Privacy and Security
Landbot's Privacy & Security Policies
Compliance with the GDPR - General Data Protection Regulation
Landbot's Abuse Policy - How to Report an Abusive Use of Landbot
Delete Customer Data in Flow
Teammates
Agent Status and Log out
Roles & Permissions for Teammates
Manage Landbot Teammates - Add and Customize Agents
Notifications section
Startup Discounts
NGOs and Educational Organizations Discount
AI in Landbot
Landbot AI Agent
Custom AI Integrations
Create a JSON format response from OpenAI in WhatsApp
Connect OpenAI Assistant with Landbot
AI in WhatsApp
How to build a FAQ chatbot with GPT-3
GPT-4 in Landbot
OpenAI
Prompt Engineering for GPT-3
Build a Customer Service Bot with ChatGPT and Extract Information
Google Gemini in Landbot
Build a Chatbot with DeepSeek
How to Build a Hybrid AI Bot
Integrations with Landbot
Native Integrations
Airtable
Airtable integration block
Get data filtered from Airtable with a Brick- Shop example
20 different ways to GET and filter data from Airtable
How to add/update different field types in Airtable (POST, PATCH & PUT)
How to Create, Update, Retrieve and Delete records in Airtable (POST, PATCH, GET & DELETE)
Get more than 100 items from Airtable
Insert Multiple Records to Airtable with a Loop
How to Get an Airtable Token
Advanced filters formulas Airtable block
Airtable usecase: Create an event registration bot with limited availability
Update Multiple Records in Airtable Using a Loop
Reservation bot with Airtable
Calendly
Dialogflow
Dialogflow & Landbot course
Dialogflow & Landbot intro: What is NLP, Dialogflow and what can you do with it?
Dialogflow & Landbot lesson 1: Create your first agent and intent in Dialogflow
Dialogflow & Landbot lesson 2: Get the JSON Key
Dialogflow & Landbot lesson 3: Setting up of Dialogflow in Landbot
Dialogflow & Landbot lesson 4: Training phrases and responses for a FAQ
Dialogflow & Landbot lesson 5: Entities and Landbot variables
Dialogflow & Landbot lesson 6: Redirect user depending on Dialogflow response parameters (intent, entities and more)
Learn more about Dialogflow - Courses and communities
Integrations > Dialogflow Block
How to extract parameters from Dialogflow response with Formulas
Dialogflow Integration Dashboard
Dialogflow in Unsupported Languages (& Multilingual)
Dialogflow - How to get JSON Key
Google Sheets
Google Sheets (Insert, Update and Get data)
How to use Google Sheets to create a simple verification system for returning visitors
How to Upload Document & store link
How to give unique Coupon Codes (with Google Spreadsheets)
Google Sheets Integration Dashboard
How to insert a new row with data and formulas in Google Sheets
How to use Google Spreadsheet as a Content Management System for your bot
Hubspot
MailChimp
Salesforce
Segment
SendGrid
Send an Email
Sendgrid Integration Dashboard
How to create a custom SendGrid email - (Custom "from" email)
Slack
Stripe
Zapier
How to Configure the Landbot and Zapier Integration Using the Zapier Block
Zapier Integration Dashboard
How to insert a row to Google Spreadsheet by Zapier
How to generate a document with PDFMonkey by Zapier
Send WhatsApp Templates from Zapier
How to send an email with Gmail by Zapier
Get Opt-ins (Contacts) from Facebook Leads using Zapier
How to extract data from an external source with Zapier and use it in Landbot
Zapier trigger
How to complete a digital signature flow by Zapier
Make a survey with Landbot and display the results in a Notion table using Zapier
Custom Integrations
ActiveCampaign
Google Calendar
Google Fonts
Google Maps
Embed Google Maps
Google Maps API Key for Address block
Extract Data With Google Maps Geocoding API
Calculate Distances With Google Maps API
Google Meet
IFTTT
Integrately
Intercom
Make
Connecting MySQL with Make.com (formerly Integromat)
Send WhatsApp Message Template from Make
Make Integration With Trigger Automation Block
How to send an email through Sendinblue by Make.com (formerly Integromat)
Get Opt-ins (Contacts) from Facebook Leads using Make
How to extract data from an external source with Make.com and use it in Landbot
OCR
Pabbly
Paragon
Pipedream
PDF Monkey
Store Locator Widgets
Xano
Zendesk
WhatsApp Channel
Getting started!
WhatsApp Testing
Build a WhatsApp Bot - Best Practices and User guide
Build a WhatsApp Bot - Best Practices for Developers
Types of Content and Media you can use in WhatsApp 🖼
1. WhatsApp Article Directory
2. WhatsApp Integration FAQ's
Adding & Managing your WhatsApp Channel
Facebook Business Verification - Best Practices 🇬🇧
WhatsApp Number Deletion (WA Channel management)
Adding a WhatsApp number to your account
WhatsApp’s Messaging Policy: New Accepted Industry verticals
Meta processes guide: FBM verification, Official Business Account (OBA) requests, Appeals
Additional Number integration: Limitations and Requirements (Number integration)
Existing WhatsApp Number Migration
Key Insights for Migrating to WhatsApp Business API Cloud
How to's, Compatibility & Workarounds
WhatsApp bots - Feature Compatibility Guide
WhatsApp - How to direct a user through a different bot flow on their second visit
WhatsApp - Get user out of error message loop
Getting Subscribers: Opt-in, Contacts
How to get Opt-ins (Contacts) for your WhatsApp 🚀
WhatsApp Quality - Best Practices
Opt-In block for WhatsApp 🚀
Opt-in Check Block
WhatsApp Channel Settings
Parent Bot/Linked Bot - Add a main bot to your WhatsApp number
WhatsApp Channel Panel (Settings)
Growth Tools for WhatsApp
Duplicating bots
Messaging and contacting your users
WhatsApp Campaigns 💌
WhatsApp's Message Templates
Audience block
WhatsApp Marketing Playbook: Best Practices for Leadgen
WhatsApp Error Logs: Troubleshooting guide
Audiences
WhatsApp for Devs
How to calculate the number of days between two selected dates (WhatsApp)
Creating a Loop in WhatsApp
Recognise the users input when sending a Message Template with buttons
Trigger Event if User Abandons Chat
Calculate Distances in WhatsApp
Send Automated Message Templates based on Dates
Trigger Event if User Abandons Chat Using AWS
reply from Slack: How to create an integration to allow agents reply WhatsApp users from Slack (with Node JS)
Set Up a Delay Timer in Bot
Notify Teammates of Chat via WhatsApp
Native blocks for WhatsApp
Other Channels - Messenger and APIChat
Facebook Messenger
The Facebook Messenger Ultimate Guide
Types of content you can use in Messenger bots 🖼
How to Preview a Messenger bot
API Chat (for Developers)
Human Takeover & Inbox
Metrics and Data Management
Metrics Section
How to export the data from your bots
Export data: How to open a CSV file
Bot's Analyze Section
For Developers & Designers
JavaScript and CSS
CSS and Design Customizations
Design Customizations
Advanced (Custom CSS & Custom JS)
Components CSS Library Index
Background Class CSS
Identify Blocks CSS
Buttons Class CSS
Header Class CSS
Media Class CSS
Message Bubble Class CSS
Miscellaneous Classes CSS
CSS Customization Examples: "Back to School" Theme
Get started guide for CSS Design in Landbot
CSS Customization Examples: Call To Action: WhatsApp
CSS Examples: Lead Gen
CSS Customization Examples: "Translucid"
CSS Customization Examples: "Minimalist" Theme
Dynamic Data CSS
Form Block CSS
CSS for Typewriter Effect
CSS Customization Examples: Carrd Embed Beginner
Dynamically Change a Bot's Background
Proactive Message Customizations with Javascript and CSS
Landbot v3 - Web CSS - RTL
CSS Customization Examples: Video Bubble
Dynamically Change Any Style
CSS Customization Examples: CV Template
Change Landbot custom CSS dynamically from parent page onload
Widget/Bubble Customizations with Javascript and CSS
JavaScript
How to change Avatar dynamically
Javascript in WhatsApp
Landbot JavaScript Integration
Different ways to format numbers with JS
How to display an HTML Table and a List in Landbot v3 web
Trigger a Global Keyword with JS (web v3)
Create Dynamic Shopping Cart with JS and CSS
Add a Chart (with Chart JS library) in your Landbot
Different ways to format numbers with JS (WhatsApp)
Pop up modal to embed third party elements
Landbot API
Send WhatsApp Messages with Landbot API
How to "send" a user to a specific point in the flow with Javascript and with the API
APIs
Get Opt-ins (Contacts) using Landbot API
MessageHooks - Landbot Webhooks
Resume flow based on external process with Landbot API (Request, Set, Go)
Tracking
Google Analytics - Track Events (Not embedded)
Google Analytics - Track Events (Embedded)
Meta Pixel - Track Events (only Embedded)
How Track Google Analytics Events in Landbot with Google Tag Manager (GTM)
Google Adwords - How to track Google Adwords in Landbot
Set a timer to get the time spent during the flow
Workarounds and How To's
Workflows
How to build an event registration Landbot (to be used in one screen by many attendees) (web only)
How to let user select a time of booking (with a minimum 45 minutes notice)
Send Files Hosted in Landbot to Your Google Drive with Make
Two-Step Email Verification
Fixing Web Bot Loading Issues for iOS Devices in Meta Campaigns with Disclaimers
Progress Bar Workaround
How to Add User Verification to Your Chatbot
How to set up questions with a countdown
HTML Template for Emails
Creating a Simple Cart in WhatsApp
More Topics
Table of Contents
- Requirements to send WhatsApp Message Templates
- The API method to Send a template
- Request Examples
- 1. Message Media Template Picture (with parameter) and Body (with parameters)
- 2. Message Media Template Header (with parameters) and Body (with parameters)
- 3. Message Media Template Header and Body (with parameters)
- 4. Message Media Template with Buttons (Quick reply cannot use parameters)
- 5. Message Media Template with Header (with parameter), Body (with parameters) and Button (with Parameters)
- 6. Message Media Template with Body (with parameters) and Click to Action (Button with Parameter)
- 7. Message Media Template with Header (document) and Body (with parameter)
- Default Agent Assignation, and how to assign it to a bot via API
- Error Logs
- All Categories
- For Developers & Designers
- Landbot API
- Send WhatsApp Messages with Landbot API
Send WhatsApp Messages with Landbot API
Updated
by Elisa
- Requirements to send WhatsApp Message Templates
- The API method to Send a template
- Request Examples
- 1. Message Media Template Picture (with parameter) and Body (with parameters)
- 2. Message Media Template Header (with parameters) and Body (with parameters)
- 3. Message Media Template Header and Body (with parameters)
- 4. Message Media Template with Buttons (Quick reply cannot use parameters)
- 5. Message Media Template with Header (with parameter), Body (with parameters) and Button (with Parameters)
- 6. Message Media Template with Body (with parameters) and Click to Action (Button with Parameter)
- 7. Message Media Template with Header (document) and Body (with parameter)
- Default Agent Assignation, and how to assign it to a bot via API
- Error Logs
It's possible to send Message Templates to your WhatsApp contacts using our API!
Let's look in detail how to do it!
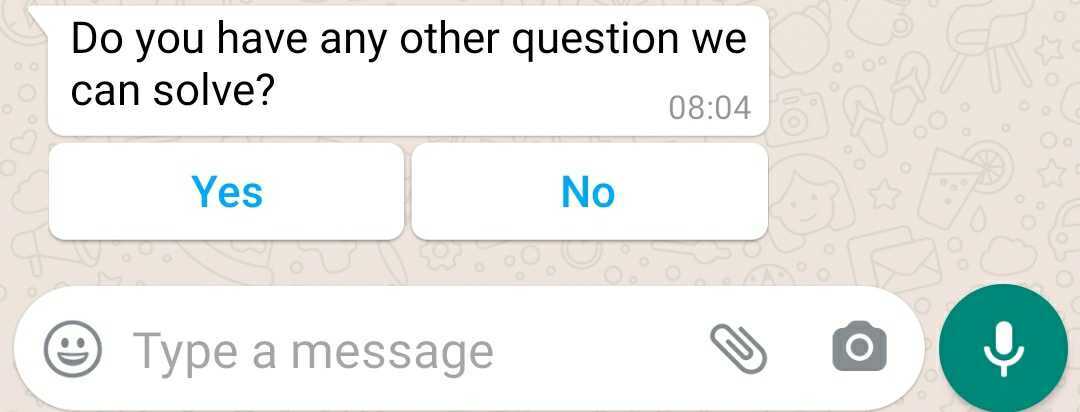
Requirements to send WhatsApp Message Templates
1. The WhatsApp Business Channel
You need to have your official WhatsApp channel, for now, it doesn't work in WhatsApp Testing channels.
2. The user's Opt-in
You need to previously have collected the user opt-in to send proactive messages to your WhatsApp contacts, please click here for more information.
3. An Approved WhatsApp Message Templates
The Message WhatsApp has to be validated previously by Meta, please click here for more information.
The API method to Send a template
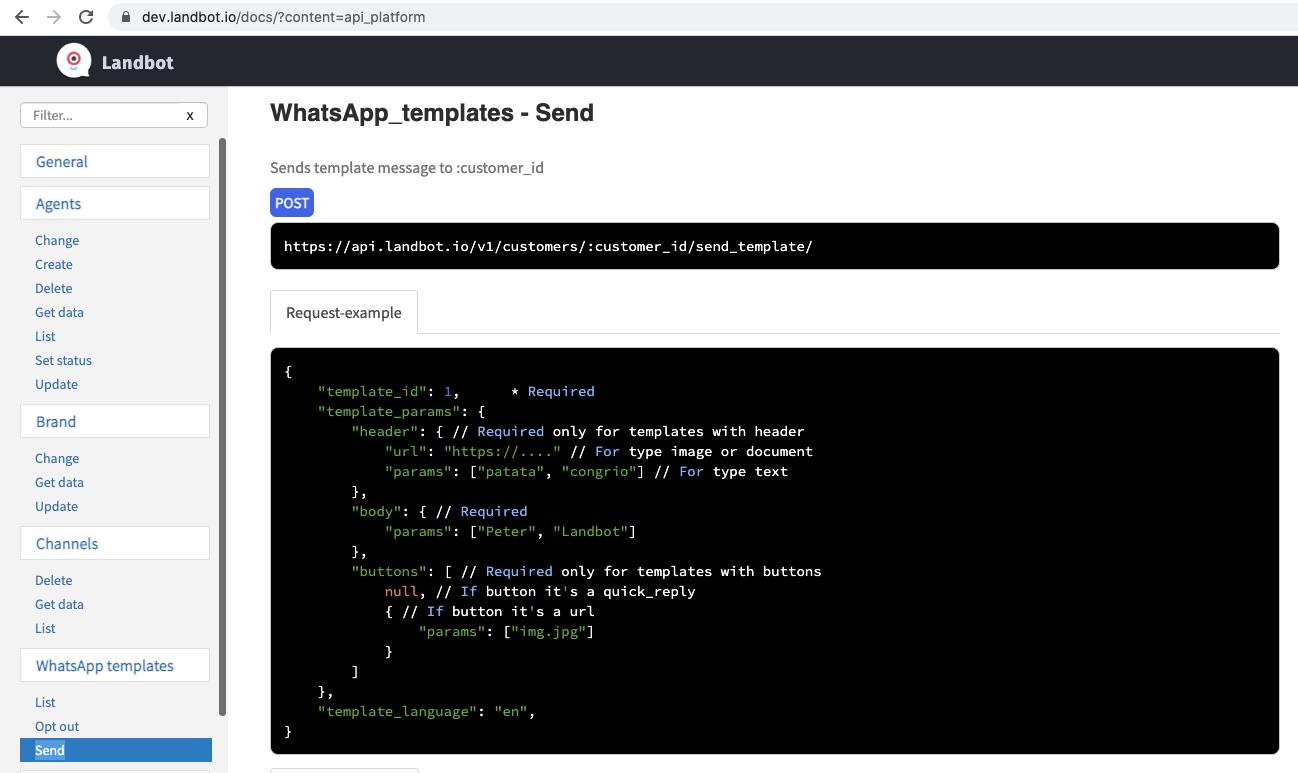
Here you can find the url to the Dev Docs documentation: Link
API Requests Authorization
Landbot Platform API requires the following header for the authorization:
Header
Field | Description |
Authorization | Default value: |
Content-Type | Default value: |
Find agent_token (API Token)
You can find your :agent_token (API Token) in https://app.landbot.io/gui/settings/account
Bear in mind that it is unique per teammate, and will behave according to the permissions granted to that teammate
Find Template ID
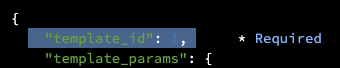
On the Message Templates section of your WhatsApp channel you will need to filter by Active templates
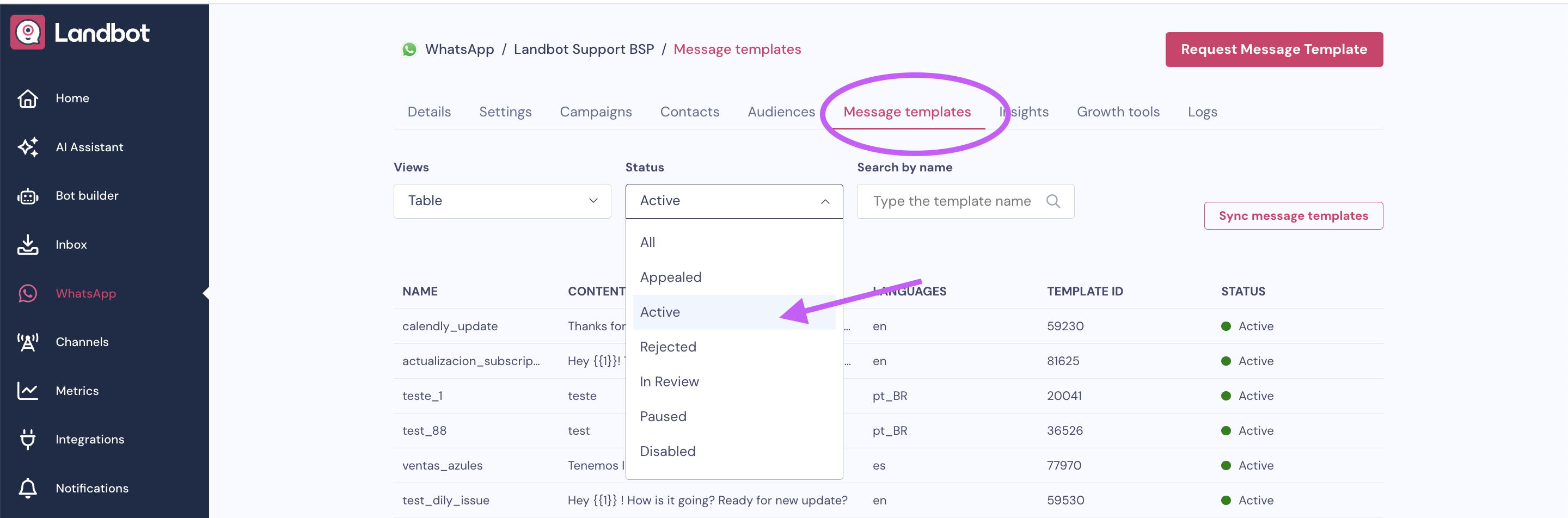
And you will see a column with the specific ID of the desired templates:
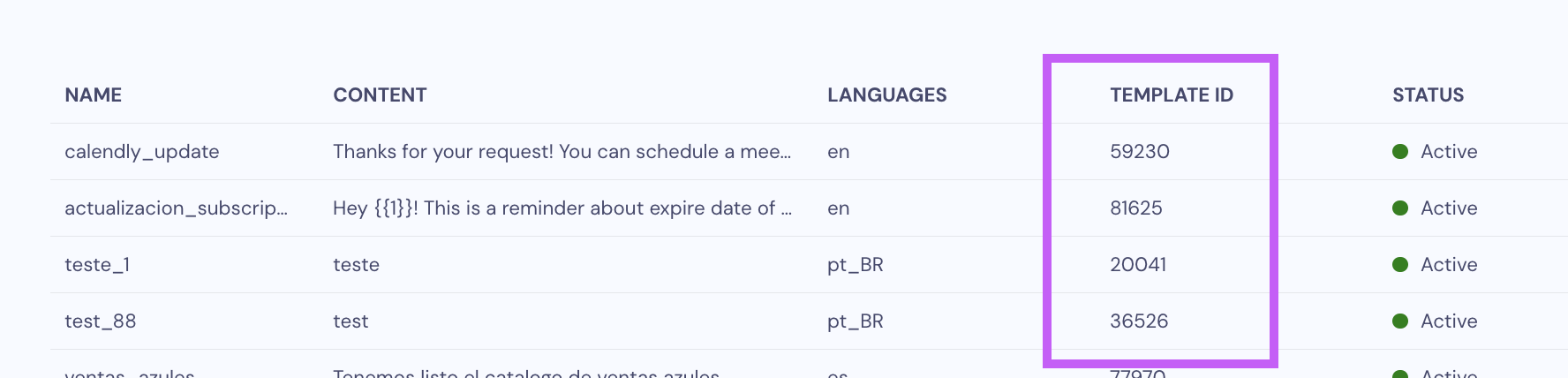
Find Template Language code
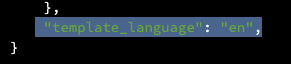
Currently the best way to find the template_language code is using the list method of the templates: Link to method
curl --location --request GET 'https://api.landbot.io/v1/channels/whatsapp/templates/' \--header 'Content-Type: application/json'
\--header 'Authorization: Token XXXXXXXXX'
Find customer id
The customer id is the basic identifier, as templates need to be sent one by one, you will need to specify the customer_id

There are several methods you can use to find the customer id of a WhatsApp user that has done the opt-in:
1. Opt-in request response
If you use the end point for the opt-in of customers, in the response of the request, it will return the id value, that is the customer_id
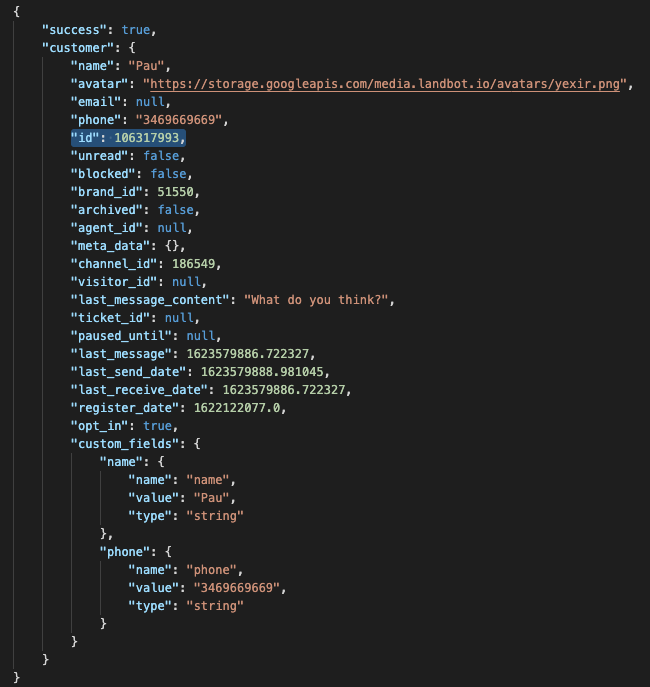
2. Via API using phone as a filter
curl --location --request GET 'https://api.landbot.io/v1/customers/?search_by=phone&search={{phone number}}&opt_in=true' \--header 'Content-Type: application/json'
\--header 'Authorization: Token XXXXXXXXX'
3. In the bot from the Analyze section under the column Customer Id
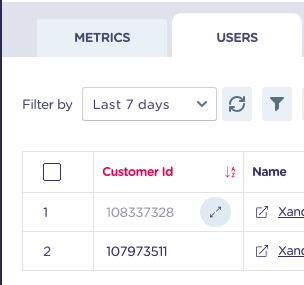
4. From the Inbox section in the top right panel
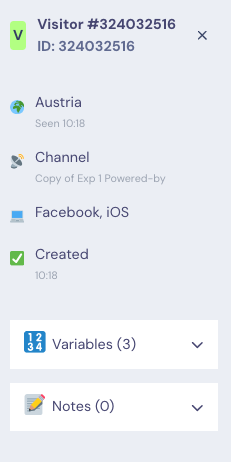
Request Examples
Here are different examples with the required code you need to send different models of Templates
1. Message Media Template Picture (with parameter) and Body (with parameters)
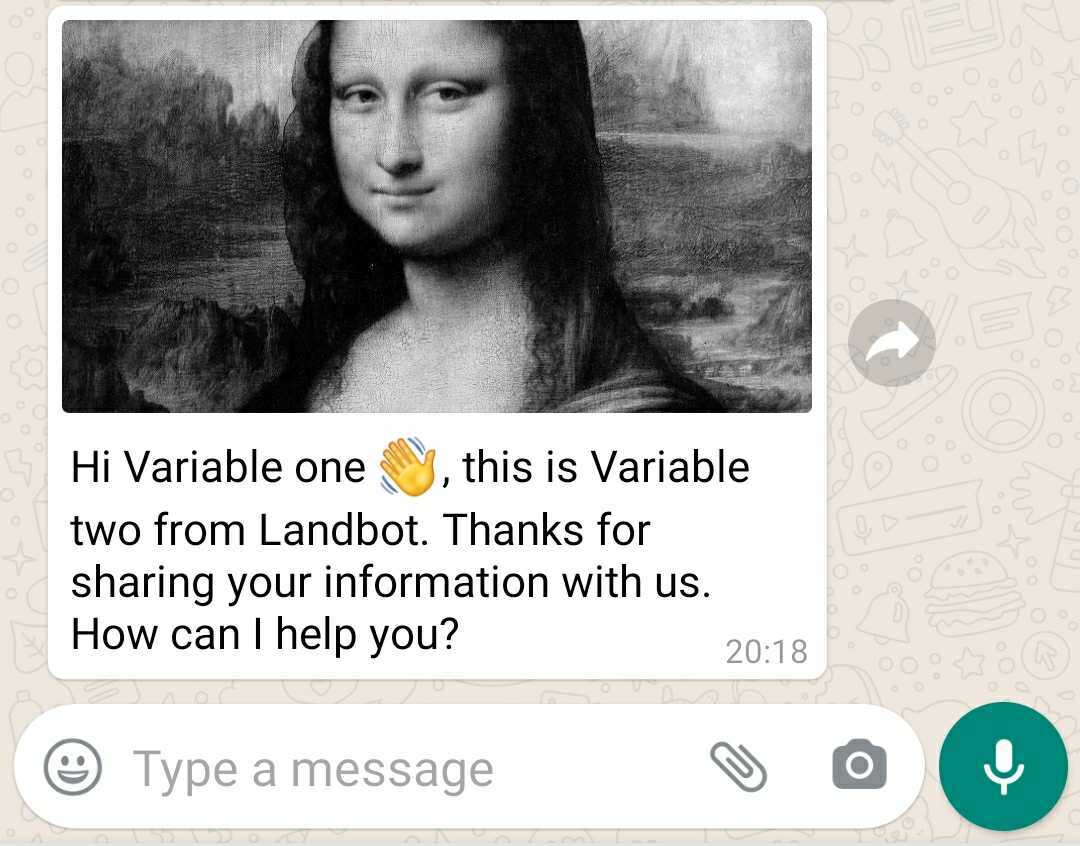
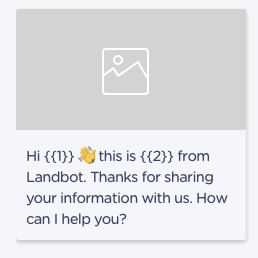
cURL snippet
curl --location --request POST 'https://api.landbot.io/v1/customers/:customerID/send_template/' \
--header 'Content-Type: application/json' \
--header 'Authorization: Token XXXXXXXXX' \
--data-raw '{
"template_id": 1082,
"template_params": {
"header": { "url":"https://upload.wikimedia.org/wikipedia/commons/6/6e/Mona_Lisa_bw_square.jpeg"
},
"body": {
"params": ["Variable one", "Variable two"]
}, "buttons":[]
},
"template_language": "en"
}'
2. Message Media Template Header (with parameters) and Body (with parameters)
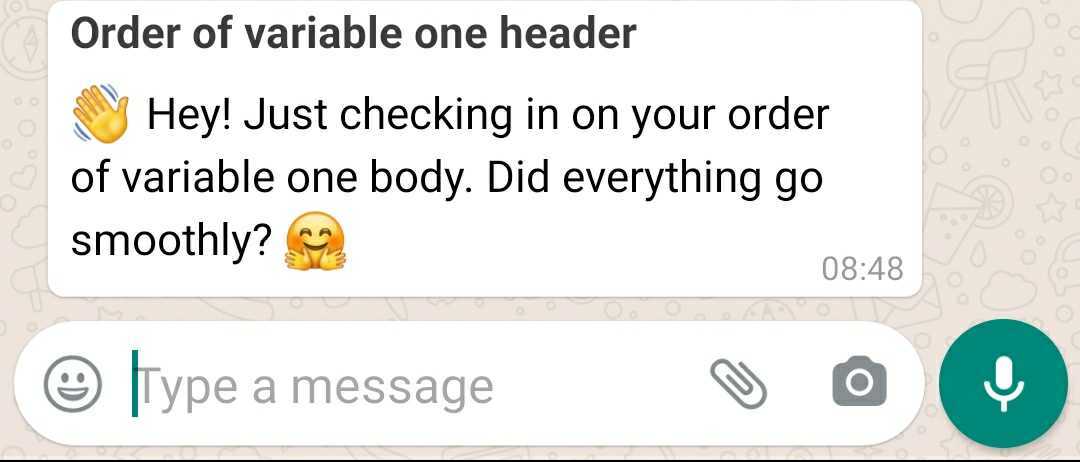
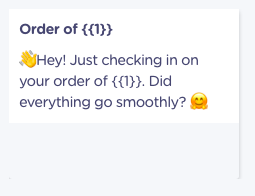
cURL snippet
curl --location --request POST 'https://api.landbot.io/v1/customers/:customerID/send_template/' \
--header 'Content-Type: application/json' \
--header 'Authorization: Token XXXXXXXXX' \
--data-raw '{
"template_id": 1137,
"template_params": {
"header": { "params": ["variable one header"] },
"body": { "params": ["variable one body"] },
"buttons":[]
},
"template_language": "en"
}'
3. Message Media Template Header and Body (with parameters)
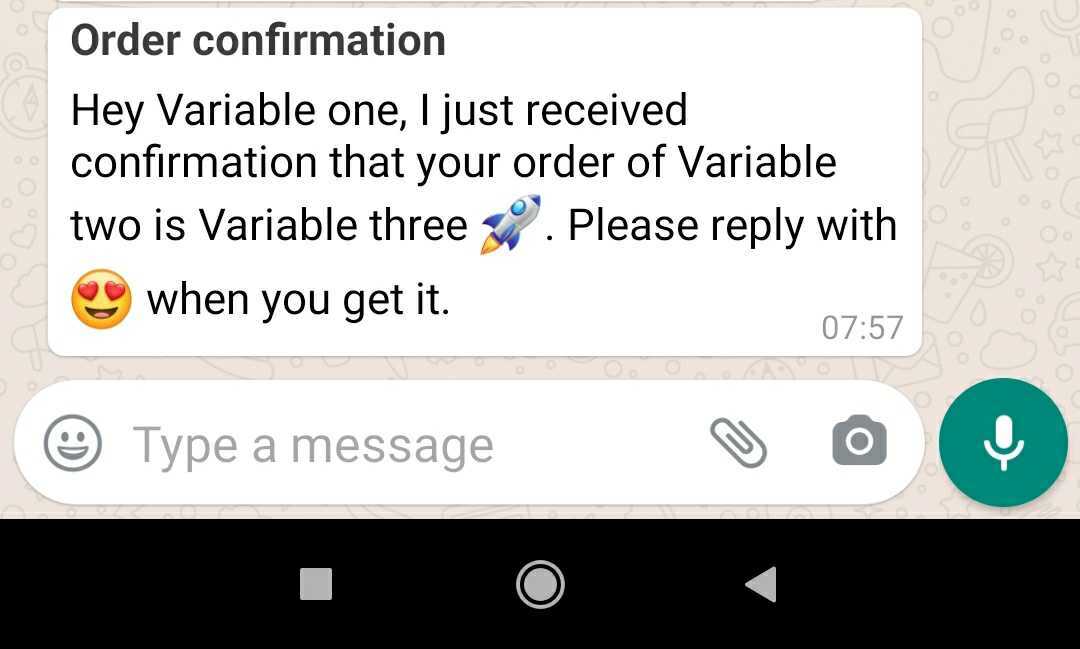
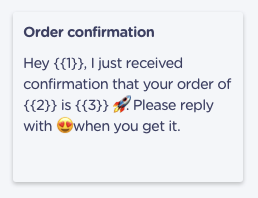
cURL snippet
curl --location --request POST 'https://api.landbot.io/v1/customers/:customerID/send_template/' \
--header 'Content-Type: application/json' \
--header 'Authorization: Token XXXXXXXXX' \
--data-raw '{
"template_id": 1074,
"template_params": {
"header": {
"params": []
},
"body": {
"params": ["Variable one", "Variable two","Variable three"]
},
"buttons":[]
},
"template_language": "en"
}
}'
4. Message Media Template with Buttons (Quick reply cannot use parameters)
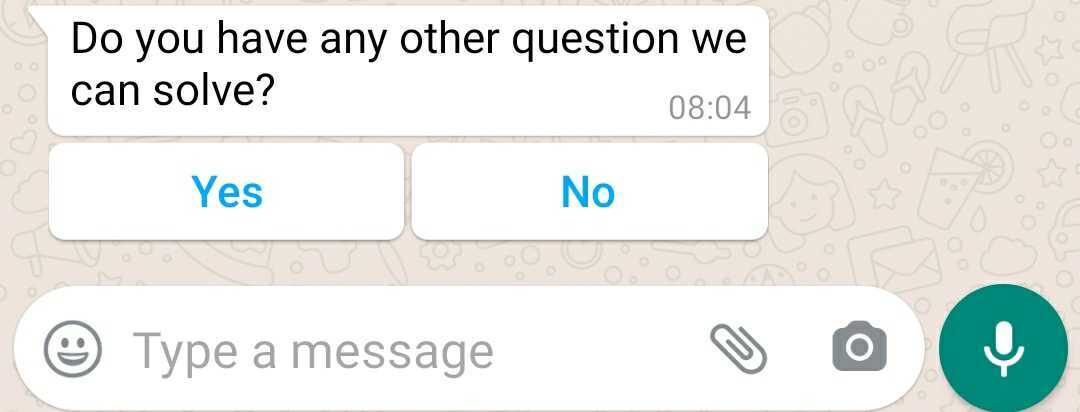
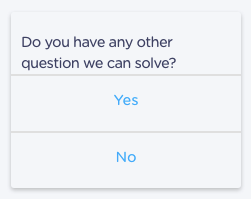
cURL snippet
curl --location --request POST 'https://api.landbot.io/v1/customers/:customerID/send_template/' \
--header 'Content-Type: application/json' \
--header 'Authorization: Token XXXXXXXXX' \
--data-raw '{
"template_id": 1200,
"template_params": {
"header": {
"params": []
},
"body": {
"params": []
},
"buttons":[]
},
"template_language": "en"
}'
5. Message Media Template with Header (with parameter), Body (with parameters) and Button (with Parameters)
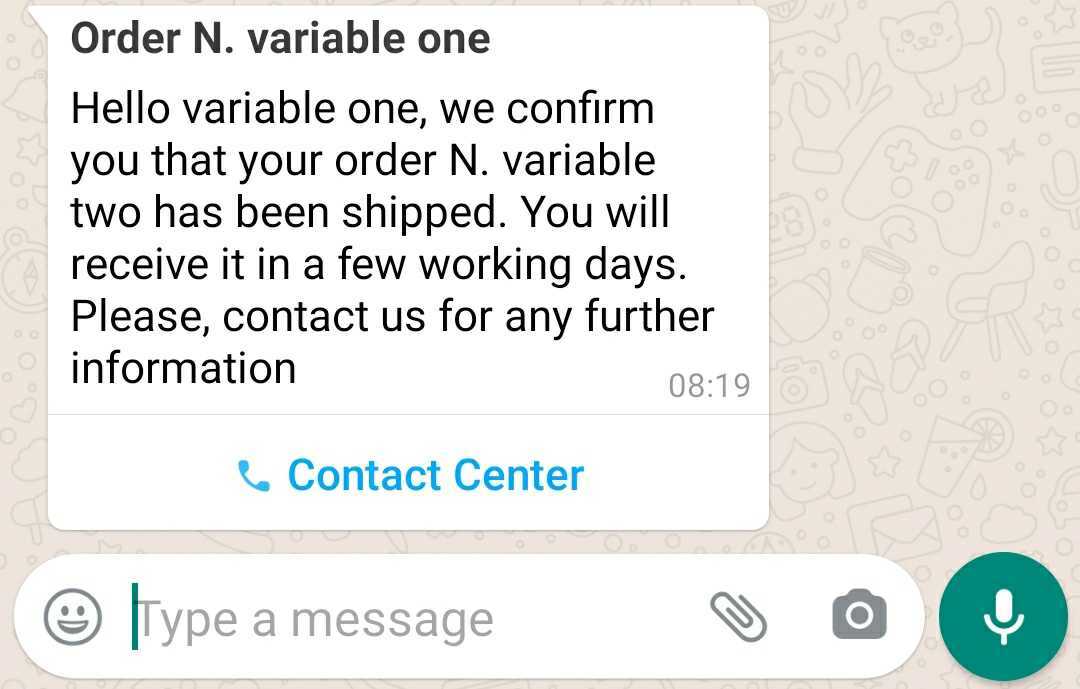
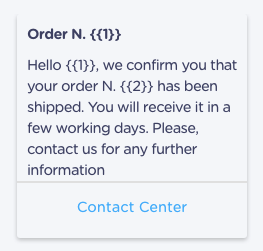
cURL snippet
curl --location --request POST 'https://api.landbot.io/v1/customers/:customerID/send_template/' \
--header 'Content-Type: application/json' \
--header 'Authorization: Token XXXXXXXXX' \
--data-raw '{
"template_id": 1243,
"template_params": {
"header": {
"params": ["variable one"]
},
"body": {
"params": ["variable one","variable two"]
},
"buttons":[]
},
"template_language": "en"
}'
6. Message Media Template with Body (with parameters) and Click to Action (Button with Parameter)
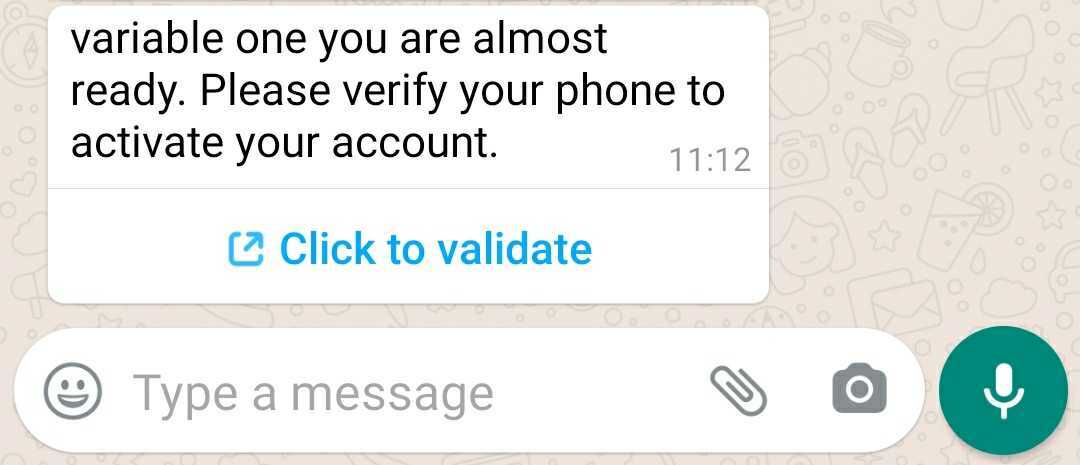
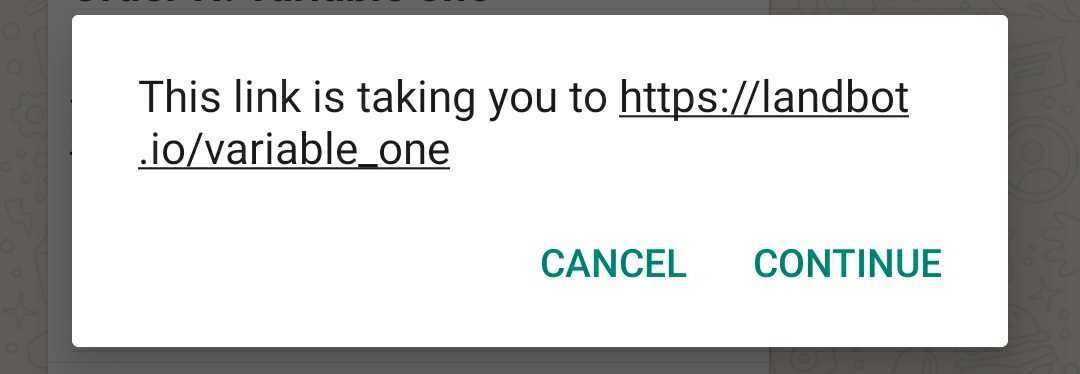
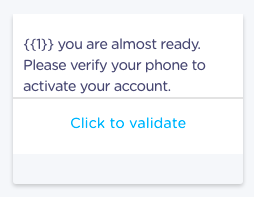
cURL snippet
curl --location --request POST 'https://api.landbot.io/v1/customers/:customerID/send_template/' \
--header 'Content-Type: application/json' \
--header 'Authorization: Token XXXXXXXXX' \
--data-raw '{
"template_id": 1725,
"template_params": {
"header": {
"params": []
},
"body": {
"params": ["variable one"]
},
"buttons":[ {
"params": ["variable_one"]
} ]
},
"template_language": "en"
}'
7. Message Media Template with Header (document) and Body (with parameter)
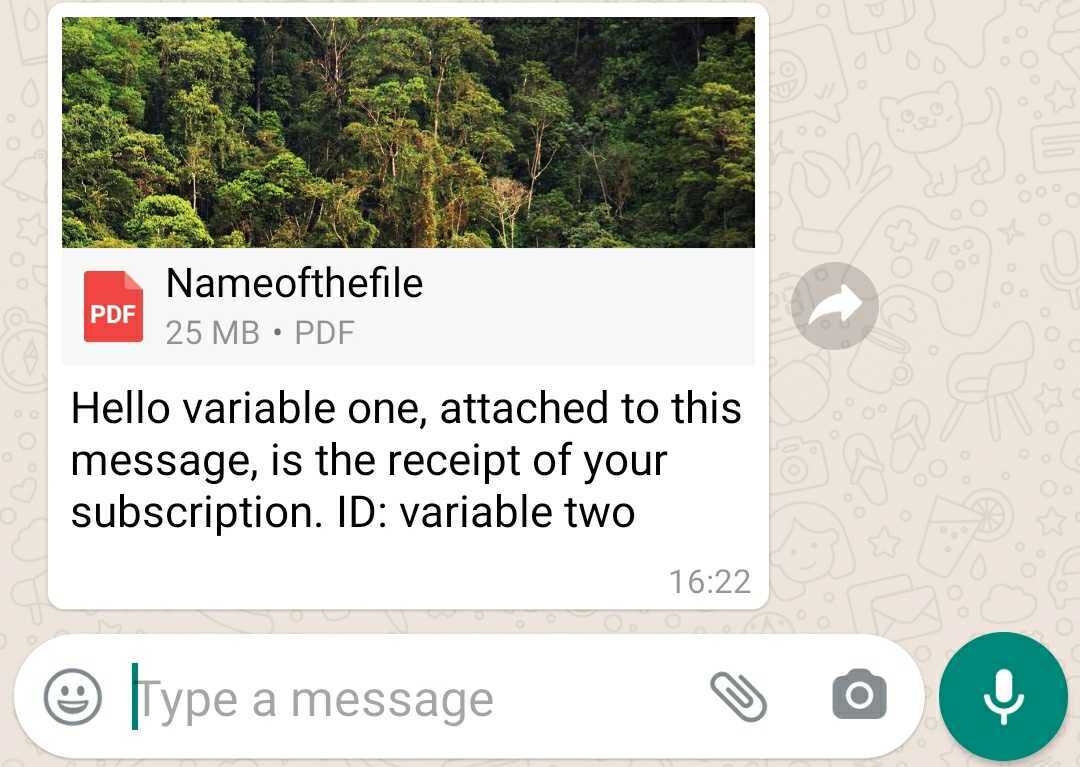
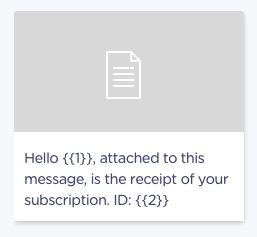
cURL snippet
curl --location --request POST 'https://api.landbot.io/v1/customers/:customerID/send_template/' \
--header 'Content-Type: application/json' \
--header 'Authorization: Token XXXXXXXXX' \
--data-raw '{
"template_id": 1726,
"template_params": {
"header": { "url": "https://www.domain.com/file.pdf",
"filename": "Nameofthefile"
},
"body": {
"params": ["variable one","variable two"]
},
"buttons":[]
},
"template_language": "en"
}'
Default Agent Assignation, and how to assign it to a bot via API
By default, once a Message Template has been sent via API, as described before, the chat will be assigned to the Agent which was used the API token
There are 2 possible approaches that you can use to redirect the user to an automated conversation, right after the first request (Send WhatsApp Message)
Unassign
It will "unassign" the chat from the agent, and after the first interaction from the user. It will be redirected to the first message, of the bot linked to the WhatsApp Channel
Link to docs: https://dev.landbot.io/api/index.html#api-Customers-PutHttpsApiLandbotIoV1CustomersCustomer_idUnassign
cURL snippet
curl --location --request PUT 'https://api.landbot.io/v1/customers/:customerID/unassign/' \
--header 'Content-Type: application/json' \
--header 'Authorization: Token XXXXXXXXX' \
--data-raw ''
Assign to bot (and to a specific block)
If you want to assign the user to a specific bot and a specific block, instead of the default first block in of the linked bot to the WhatsApp Channel
Link to docs: https://dev.landbot.io/api/index.html#api-Customers-PutHttpsApiLandbotIoV1CustomersCustomer_idAssign_botBot_id
cURL snippet
curl --location --request PUT 'https://api.landbot.io/v1/customers/:customer_id/assign_bot/:bot_id/' \
--header 'Content-Type: application/json' \
--header 'Authorization: Token XXXXXXXXX' \
--data-raw '{
"launch": false,
"node": "block_id"
}'
Find :bot_id
To find the bot id, go to the bot inside the app, and the number in the url, is the bot id
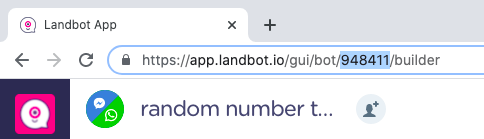
Find :block_id
To retrieve the block id, in the bot builder, select the block where you want the bot to use, click on the three dots of the block and select Copy block id, will display. Once you click it will set the number in your clipboard
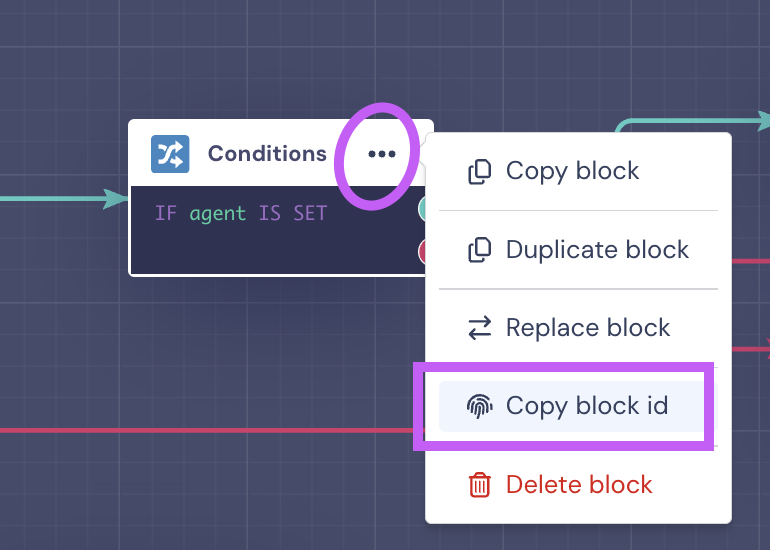
Error Logs
On the Logs section of your WhatsApp channel you'll be able too se If there was any issue generated when sending the Message Templates on the 'Errors' sections
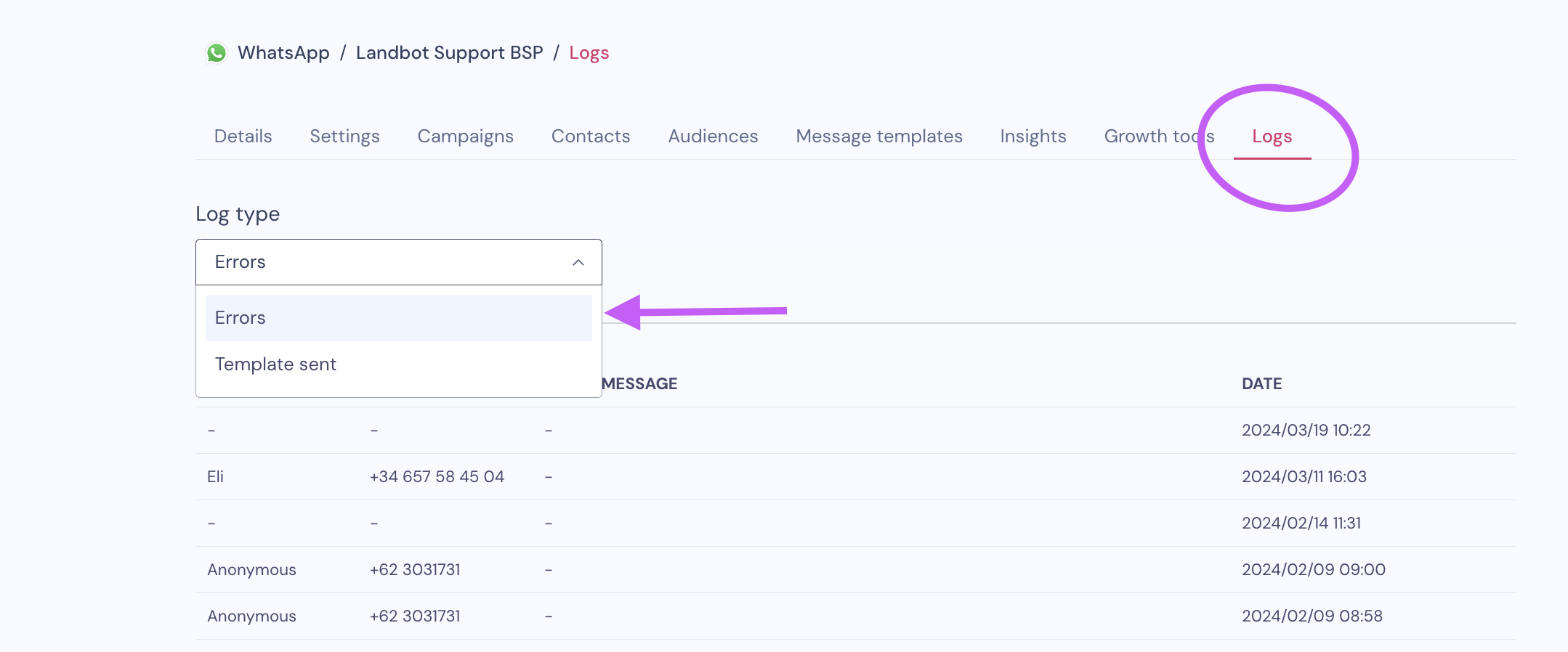