Build a bot
Getting started
Basics and builder
Managing Data in Your Chatbot: A Guide to Using Fields
Builder's keyboard shortcuts and mechanics! 🏗
Builder Interface
How to "debug" (troubleshoot) your bot's flow to spot possible errors (for non coders)
Starting Point
How to disable a bot
Bricks
Bot's Settings
Bot General Settings
Second Visit Settings
Custom System Messages
Hidden Fields (Get params / UTMs from url and use it as variables)
Landbot native SEO & Tracking tools
Typing Emulation (Message Delay)
How to Import a Chatbot Flow Without JSON – Use "Build It For Me" Feature
Getting started - build a bot
Messages, Questions and Logic & Technical blocks
Messages
Media Block
Media block
How to display images with a variable URL source
How to embed a .gif file inside a message
Different ways to embed Videos in Landbot
Display video and hide button to continue until video has ended
Send a Message block - Simple Message
Goodbye block
Question blocks
Date Block
Scale Block
Buttons block
Ask for a Name block
Ask for an Email block
Ask a Question block
Question: Phone block
Forms block
How to set up Multiple Choice questions
Question: Address block
Question: Autocomplete block
Question: File block
Question: Number block
Question: Picture Choice block
Question: Rating block
Question: URL block
Question: Yes/No block
Logic & Technical blocks
Code Blocks
Dynamic Data
Dynamic Data (Arrays)
Get the array's index of the user selection and extract information from array
Formulas
How to Perform Basic Calculations
Get started with the Formulas block
Formulas Blocks Dashboard
Formulas - Regex
Formulas - Date
Formulas - String
Formulas - Logical
Formulas - Math
Formulas - Object
Formulas - Comparison
Formulas - Array
Persistent Menu
Trigger Automation
Webhook
Webhook Block Dashboard
Webhook Block: Simplify API Integrations in Landbot
How to TEST your Http Request (Webhook block)
Landbot System Fields
Set a Field block
Any of the above Output
Global Keywords 🌍
Keyword Jump
Lead Scoring block
Jump To block
AB Test
Conditions block
Conditions block II (with Dates, Usage and Agents variables)
Close Chat block
How to ask a question based on a variable not being set (empty URL params)
Business Hours block
Custom Goals
Note block
Using Flow Logic in Landbot
Share & Embed
Redirect Users
How to open a new URL in another tab (window)
How to redirect visitors to a URL (web only)
Conditional Welcome (Non-embedded bots)
How to add a Click-to-Call/Email/WhatsApp button
Redirect User Based on Language Input (DeepL)
Generate a URL that has variables from user answers
Popup on Exit Intent
Share
Customized Embed Actions
How to redirect user to another url in your site with Livechat open to continue conversation
How to Detect Visitors Browser
Customize and embed your WhatsApp Widget
Modifying Embed Size
Conditional Welcome: Direct the Flow of Your Bot Based on External Parameters
Detect if bot was opened
Customized Behavior in Mobile Browsers
Load script and display bot on click button
Launch Bot On Exit Intent
Display Bot During Business Hours Only (Livechat & Popup)
Open / Close a Web bot (embedded)
Launching a bot depending on browser language
How to pass WordPress logged in user data to Landbot
Set the flow depending on the url path (for embedded landbots)
How to launch a Landbot by clicking a button
Open LiveChat bot as soon as page loads
Detect if a visitor is on Mobile/Tablet or Desktop
Embed
Embed your bot into your website and use a custom domain
Embed Landbot in an iframe
Landbot in Wix
Landbot in your web with Google Tag Manager
Landbot in Webflow
Embed in Sharetribe
Landbot in Shopify
Embedding Landbot in Carrd
Landbot in Wordpress
Landbot in Squarespace
Customizing the Proactive Message
Design section (web bots)
Verification & Security
Account Settings and Billing
Billing
Account Settings
Trial period
Account Settings
Common reasons for not receiving account activation email
How to create your Landbot account, set it up and invite teammates
Navigating the Landbot Interface 🧭
Privacy and Security
Landbot's Privacy & Security Policies
Compliance with the GDPR - General Data Protection Regulation
Landbot's Abuse Policy - How to Report an Abusive Use of Landbot
Delete Customer Data in Flow
Teammates
Agent Status and Log out
Roles & Permissions for Teammates
Manage Landbot Teammates - Add and Customize Agents
Notifications section
Startup Discounts
NGOs and Educational Organizations Discount
AI in Landbot
Landbot AI Agent
AI Agents Overview
How to connect a Chatbot flow to an AI Agent
How to Create an AI Agent to Detect User Intent
Exit Conditions
Tips to migrate from old AI Assistants to AI Agents
How to create custom Instructions for your Landbot AI Agent with AI (ChatGPT, Claude...)
Capture, generate and use data with AI Agents
Custom AI Integrations
Create a JSON format response from OpenAI in WhatsApp
Connect OpenAI Assistant with Landbot
AI in WhatsApp
How to build a FAQ chatbot with GPT-3
GPT-4 in Landbot
OpenAI
Prompt Engineering for GPT-3
Build a Customer Service Bot with ChatGPT and Extract Information
Google Gemini in Landbot
Build a Chatbot with DeepSeek
How to Build a Hybrid AI Bot
Integrations with Landbot
Native Integrations
Airtable
Airtable integration block
Get data filtered from Airtable with a Brick- Shop example
20 different ways to GET and filter data from Airtable
How to add/update different field types in Airtable (POST, PATCH & PUT)
How to Create, Update, Retrieve and Delete records in Airtable (POST, PATCH, GET & DELETE)
Get more than 100 items from Airtable
Insert Multiple Records to Airtable with a Loop
How to Get an Airtable Token
Advanced filters formulas Airtable block
Airtable usecase: Create an event registration bot with limited availability
Update Multiple Records in Airtable Using a Loop
Reservation bot with Airtable
Calendly
Dialogflow
Dialogflow & Landbot course
Dialogflow & Landbot intro: What is NLP, Dialogflow and what can you do with it?
Dialogflow & Landbot lesson 1: Create your first agent and intent in Dialogflow
Dialogflow & Landbot lesson 2: Get the JSON Key
Dialogflow & Landbot lesson 3: Setting up of Dialogflow in Landbot
Dialogflow & Landbot lesson 4: Training phrases and responses for a FAQ
Dialogflow & Landbot lesson 5: Entities and Landbot variables
Dialogflow & Landbot lesson 6: Redirect user depending on Dialogflow response parameters (intent, entities and more)
Learn more about Dialogflow - Courses and communities
Integrations > Dialogflow Block
How to extract parameters from Dialogflow response with Formulas
Dialogflow Integration Dashboard
Dialogflow in Unsupported Languages (& Multilingual)
Dialogflow - How to get JSON Key
Google Sheets
Google Sheets (Insert, Update and Get data)
How to use Google Sheets to create a simple verification system for returning visitors
How to Upload Document & store link
How to give unique Coupon Codes (with Google Spreadsheets)
Google Sheets Integration Dashboard
How to insert a new row with data and formulas in Google Sheets
How to use Google Spreadsheet as a Content Management System for your bot
Hubspot
MailChimp
Salesforce
Segment
SendGrid
Send an Email
Sendgrid Integration Dashboard
How to create a custom SendGrid email - (Custom "from" email)
Slack
Stripe
Zapier
How to Configure the Landbot and Zapier Integration Using the Zapier Block
Zapier Integration Dashboard
How to insert a row to Google Spreadsheet by Zapier
How to generate a document with PDFMonkey by Zapier
Send WhatsApp Templates from Zapier
How to send an email with Gmail by Zapier
Get Opt-ins (Contacts) from Facebook Leads using Zapier
How to extract data from an external source with Zapier and use it in Landbot
Zapier trigger
How to complete a digital signature flow by Zapier
Make a survey with Landbot and display the results in a Notion table using Zapier
Custom Integrations
ActiveCampaign
Google Calendar
Google Fonts
Google Maps
Embed Google Maps
Google Maps API Key for Address block
Extract Data With Google Maps Geocoding API
Calculate Distances With Google Maps API
Google Meet
IFTTT
Integrately
Intercom
Make
Connecting MySQL with Make.com (formerly Integromat)
Send WhatsApp Message Template from Make
Make Integration With Trigger Automation Block
How to send an email through Sendinblue by Make.com (formerly Integromat)
Get Opt-ins (Contacts) from Facebook Leads using Make
How to extract data from an external source with Make.com and use it in Landbot
OCR
Pabbly
Paragon
Pipedream
PDF Monkey
Store Locator Widgets
Xano
Zendesk
WhatsApp Channel
Getting started!
WhatsApp Testing
Build a WhatsApp Bot - Best Practices and User guide
Build a WhatsApp Bot - Best Practices for Developers
Types of Content and Media you can use in WhatsApp 🖼
1. WhatsApp Article Directory
2. WhatsApp Integration FAQ's
Adding & Managing your WhatsApp Channel
Facebook Business Verification - Best Practices 🇬🇧
WhatsApp Number Deletion (WA Channel management)
Adding a WhatsApp number to your account
WhatsApp’s Messaging Policy: New Accepted Industry verticals
Meta processes guide: FBM verification, Official Business Account (OBA) requests, Appeals
Additional Number integration: Limitations and Requirements (Number integration)
Existing WhatsApp Number Migration
Key Insights for Migrating to WhatsApp Business API Cloud
How to's, Compatibility & Workarounds
WhatsApp bots - Feature Compatibility Guide
WhatsApp - How to direct a user through a different bot flow on their second visit
WhatsApp - Get user out of error message loop
How to do Meta ads conversion tracking in WhatsApp bot using the Conversion API
Getting Subscribers: Opt-in, Contacts
How to get Opt-ins (Contacts) for your WhatsApp 🚀
WhatsApp Quality - Best Practices
Opt-In block for WhatsApp 🚀
Opt-in Check Block
WhatsApp Channel Settings
Parent Bot/Linked Bot - Add a main bot to your WhatsApp number
WhatsApp Channel Panel (Settings)
Growth Tools for WhatsApp
Duplicating bots
Messaging and contacting your users
WhatsApp Campaigns 💌
WhatsApp's Message Templates
Audience block
WhatsApp Marketing Playbook: Best Practices for Leadgen
WhatsApp Error Logs: Troubleshooting guide
Audiences
WhatsApp for Devs
How to calculate the number of days between two selected dates (WhatsApp)
Creating a Loop in WhatsApp
Recognise the users input when sending a Message Template with buttons
Trigger Event if User Abandons Chat
Calculate Distances in WhatsApp
Send Automated Message Templates based on Dates
reply from Slack: How to create an integration to allow agents reply WhatsApp users from Slack (with Node JS)
Set Up a Delay Timer in Bot
Notify Teammates of Chat via WhatsApp
Native blocks for WhatsApp
Other Channels - Messenger and APIChat
Facebook Messenger
The Facebook Messenger Ultimate Guide
Types of content you can use in Messenger bots 🖼
How to Preview a Messenger bot
API Chat (for Developers)
Human Takeover & Inbox
Metrics and Data Management
Metrics Section
How to export the data from your bots
Export data: How to open a CSV file
Bot's Analyze Section
For Developers & Designers
JavaScript and CSS
CSS and Design Customizations
Design Customizations
Advanced (Custom CSS & Custom JS)
Components CSS Library Index
Background Class CSS
Identify Blocks CSS
Buttons Class CSS
Header Class CSS
Media Class CSS
Message Bubble Class CSS
Miscellaneous Classes CSS
CSS Customization Examples: "Back to School" Theme
Get started guide for CSS Design in Landbot
CSS Customization Examples: Call To Action: WhatsApp
CSS Examples: Lead Gen
CSS Customization Examples: "Translucid"
CSS Customization Examples: "Minimalist" Theme
Dynamic Data CSS
Form Block CSS
CSS for Typewriter Effect
CSS Customization Examples: Carrd Embed Beginner
Dynamically Change a Bot's Background
Proactive Message Customizations with Javascript and CSS
Landbot v3 - Web CSS - RTL
CSS Customization Examples: Video Bubble
Dynamically Change Any Style
CSS Customization Examples: CV Template
Change Landbot custom CSS dynamically from parent page onload
Widget/Bubble Customizations with Javascript and CSS
JavaScript
How to change Avatar dynamically
Javascript in WhatsApp
Landbot JavaScript Integration
Different ways to format numbers with JS
How to display an HTML Table and a List in Landbot v3 web
Trigger a Global Keyword with JS (web v3)
Create Dynamic Shopping Cart with JS and CSS
Add a Chart (with Chart JS library) in your Landbot
Different ways to format numbers with JS (WhatsApp)
Pop up modal to embed third party elements
Landbot API
Send WhatsApp Messages with Landbot API
How to "send" a user to a specific point in the flow with Javascript and with the API
APIs
Get Opt-ins (Contacts) using Landbot API
MessageHooks - Landbot Webhooks
Resume flow based on external process with Landbot API (Request, Set, Go)
Tracking
Google Analytics - Track Events (Not embedded)
Google Analytics - Track Events (Embedded)
Meta Pixel - Track Events (only Embedded)
How Track Google Analytics Events in Landbot with Google Tag Manager (GTM)
Google Adwords - How to track Google Adwords in Landbot
Set a timer to get the time spent during the flow
Workarounds and How To's
Workflows
How to build an event registration Landbot (to be used in one screen by many attendees) (web only)
How to let user select a time of booking (with a minimum 45 minutes notice)
Send Files Hosted in Landbot to Your Google Drive with Make
Two-Step Email Verification
Fixing Web Bot Loading Issues for iOS Devices in Meta Campaigns with Disclaimers
Progress Bar Workaround
How to Add User Verification to Your Chatbot
How to set up questions with a countdown
HTML Template for Emails
Creating a Simple Cart in WhatsApp
More Topics
Table of Contents
- All Categories
- WhatsApp Channel
- WhatsApp for Devs
- Trigger Event if User Abandons Chat
Trigger Event if User Abandons Chat
Updated
by Abby
Trigger Event with Pipedream
If a user abandons a chat there are several actions we can take to get them to re-engage.
Here we will use Pipedream, where we will set a delay to a custom amount of time, then after that time has passed we will launch the bot assigning the user to a conditions block, this conditions block will check if the variable 'flow completed' is false, if so we have several options, if we've already obtained their email address we can add a 'Send an email' block to send them an email and re-engage them
For WhatsApp we could add a 'Send an HSM' block after the condition 'flow completed' has been verified
The flow:
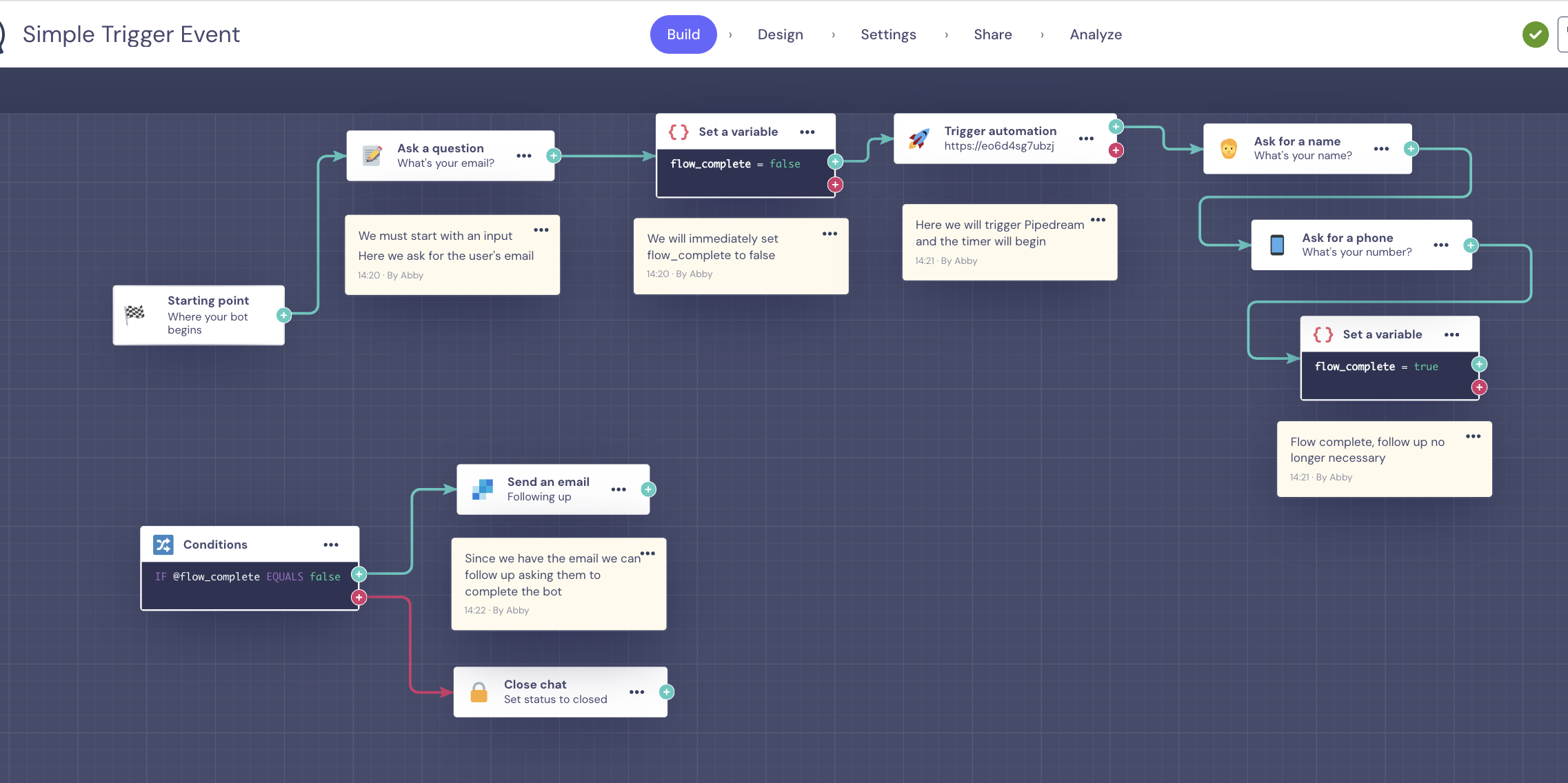
At the beginning of our flow we have the variable 'flow_complete' that equals false, if you change the name of this variable you will need to change condition block as well
At the end of our flow we will change 'flow_complete' to true, this means we won't send any follow up if they reach this point, we will simply close the chat
Pipedream:
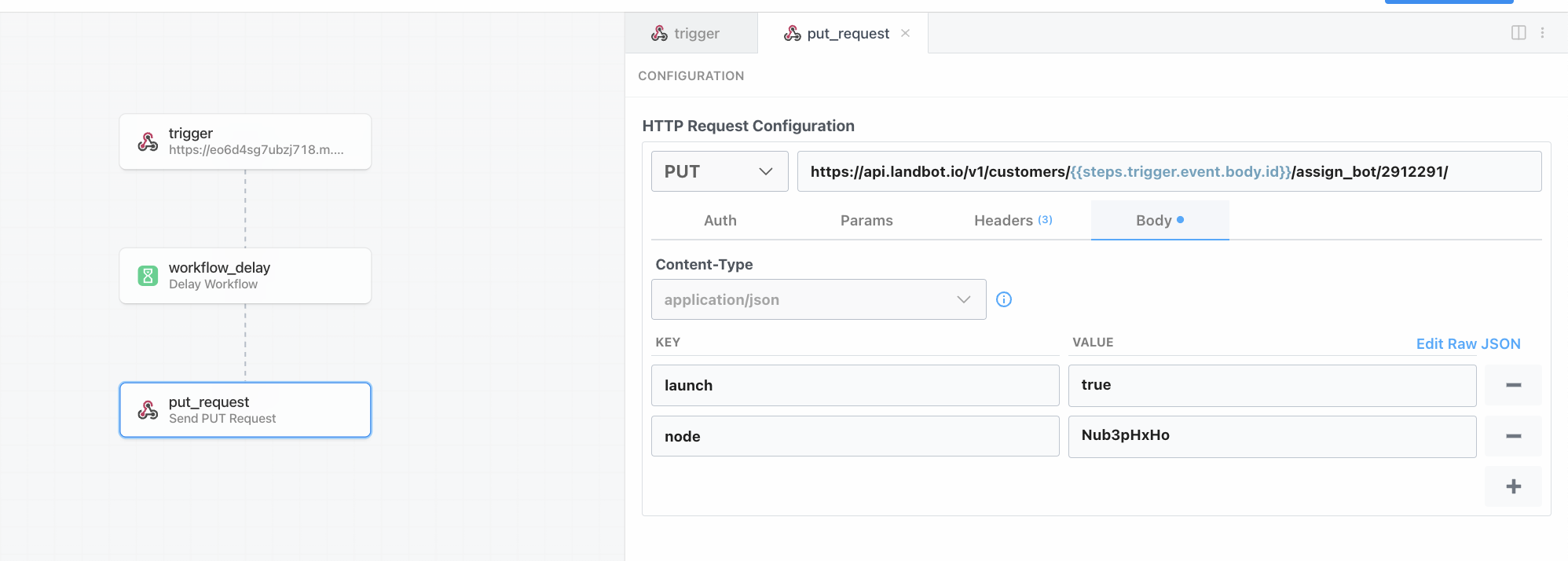
The first module we use here is a trigger, this takes in the user ID from Landbot (we'll get to that later)
This is what the configuration will look like:
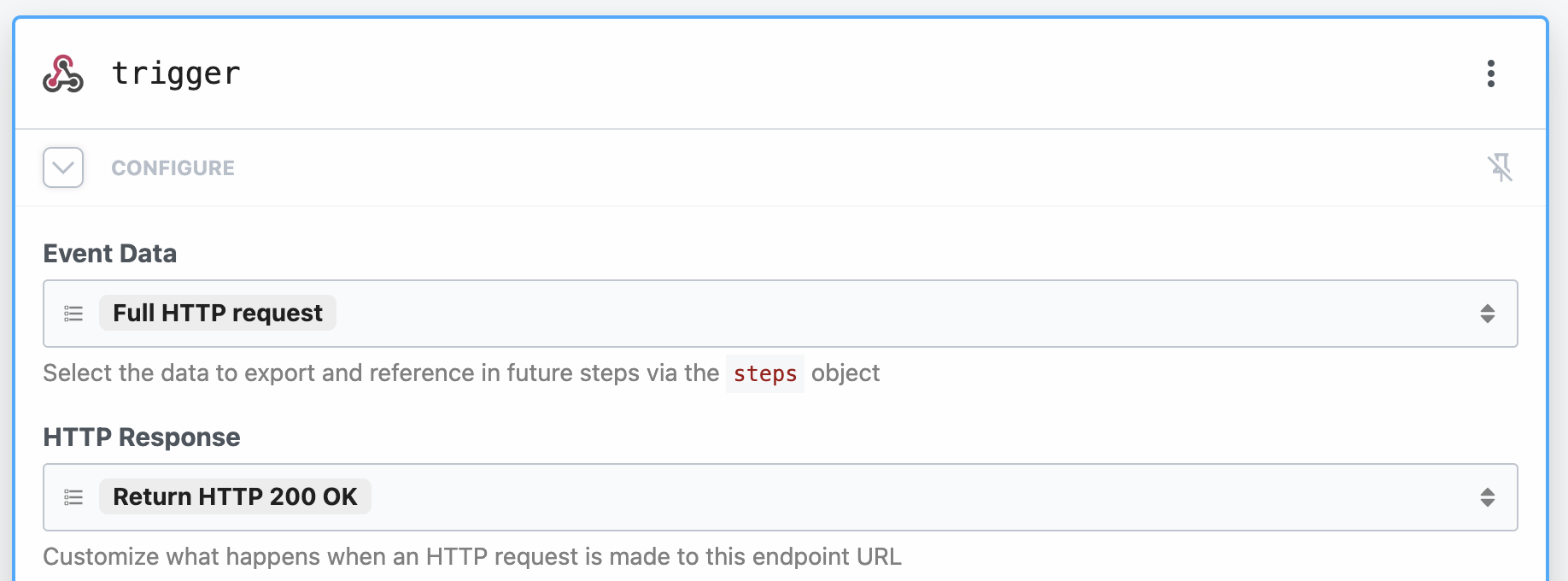
Next we'll copy the unique URL that it gives us:
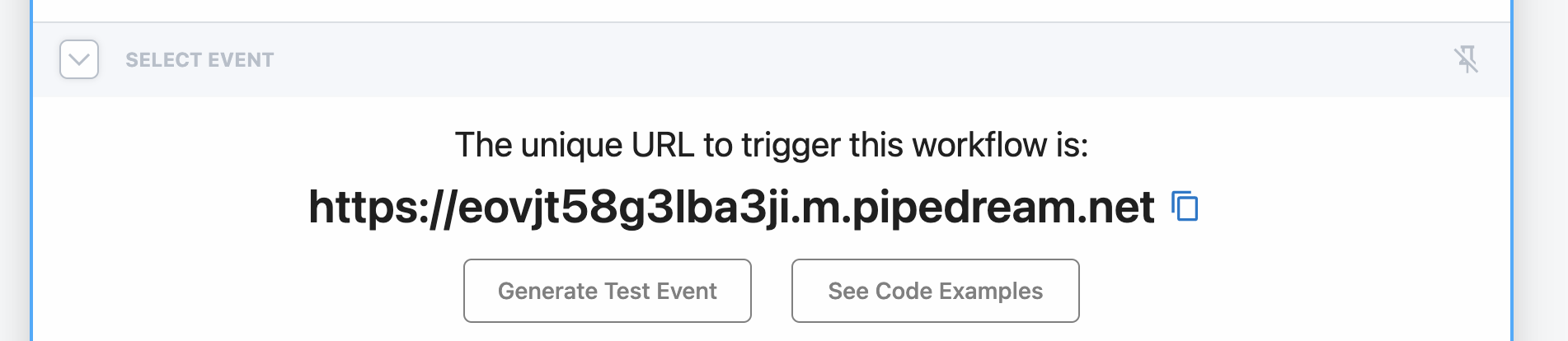
Let's go back to the bot, we'll add a Trigger Automation block with the following test values:
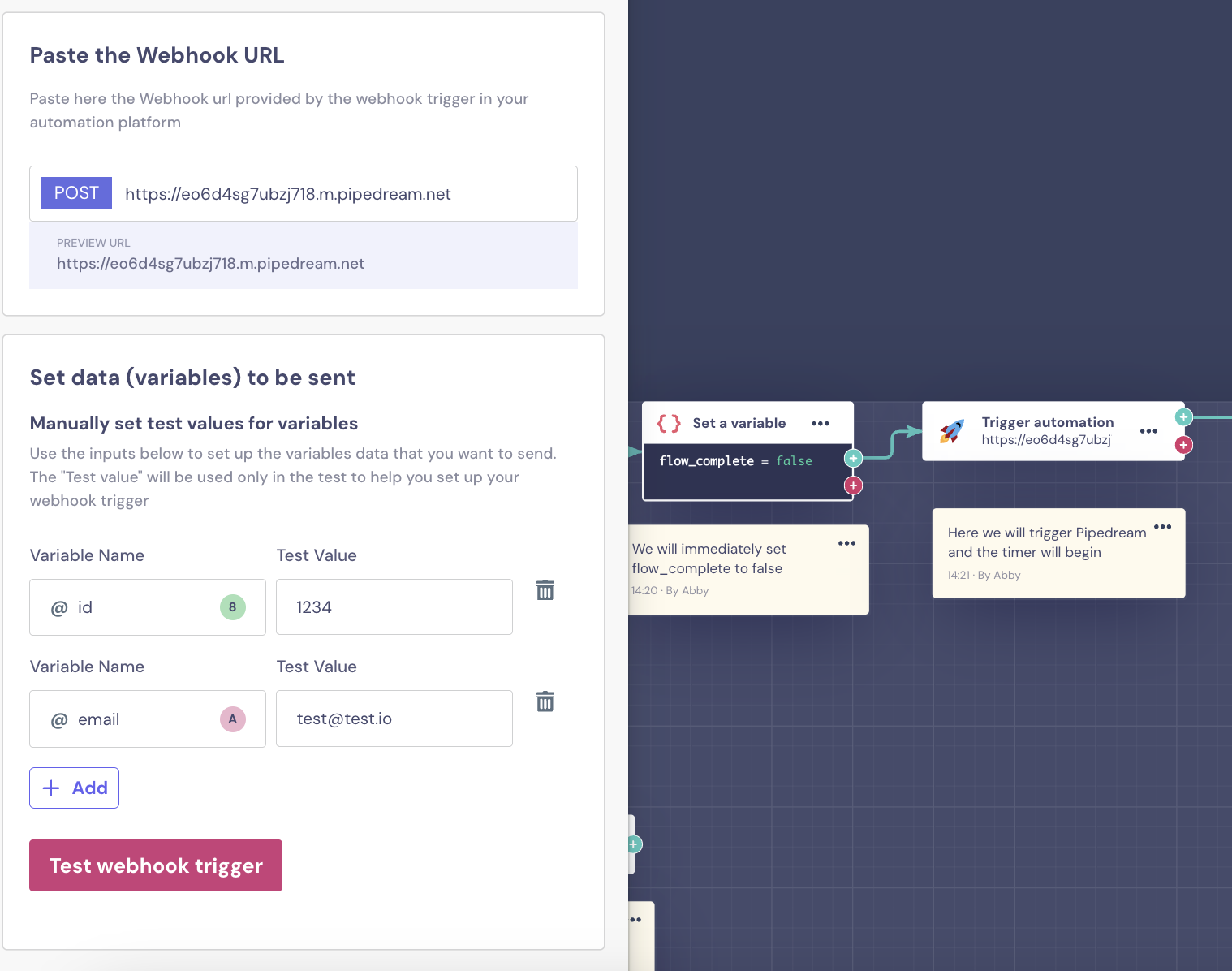
We'll test this using 'Test webhook trigger' just to be sure we've got the right URL, it should return a 200 in Landbot, and the results should look like this in Pipedream:
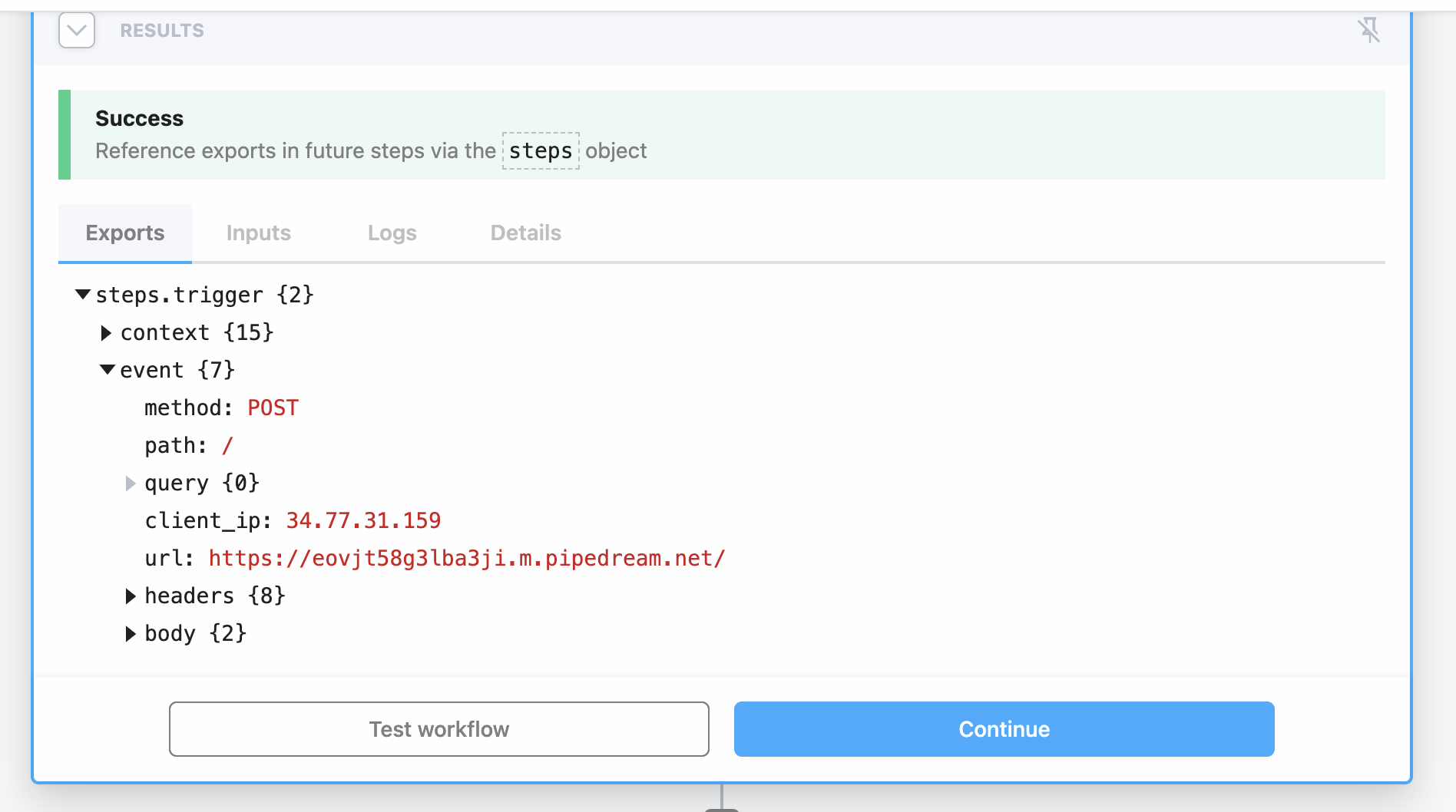
Let's press continue
Next we'll add the workflow_delay module, we can select seconds, minutes, or hours. I've selected 23 hours for the bot in production, however for testing I've selected 8 seconds
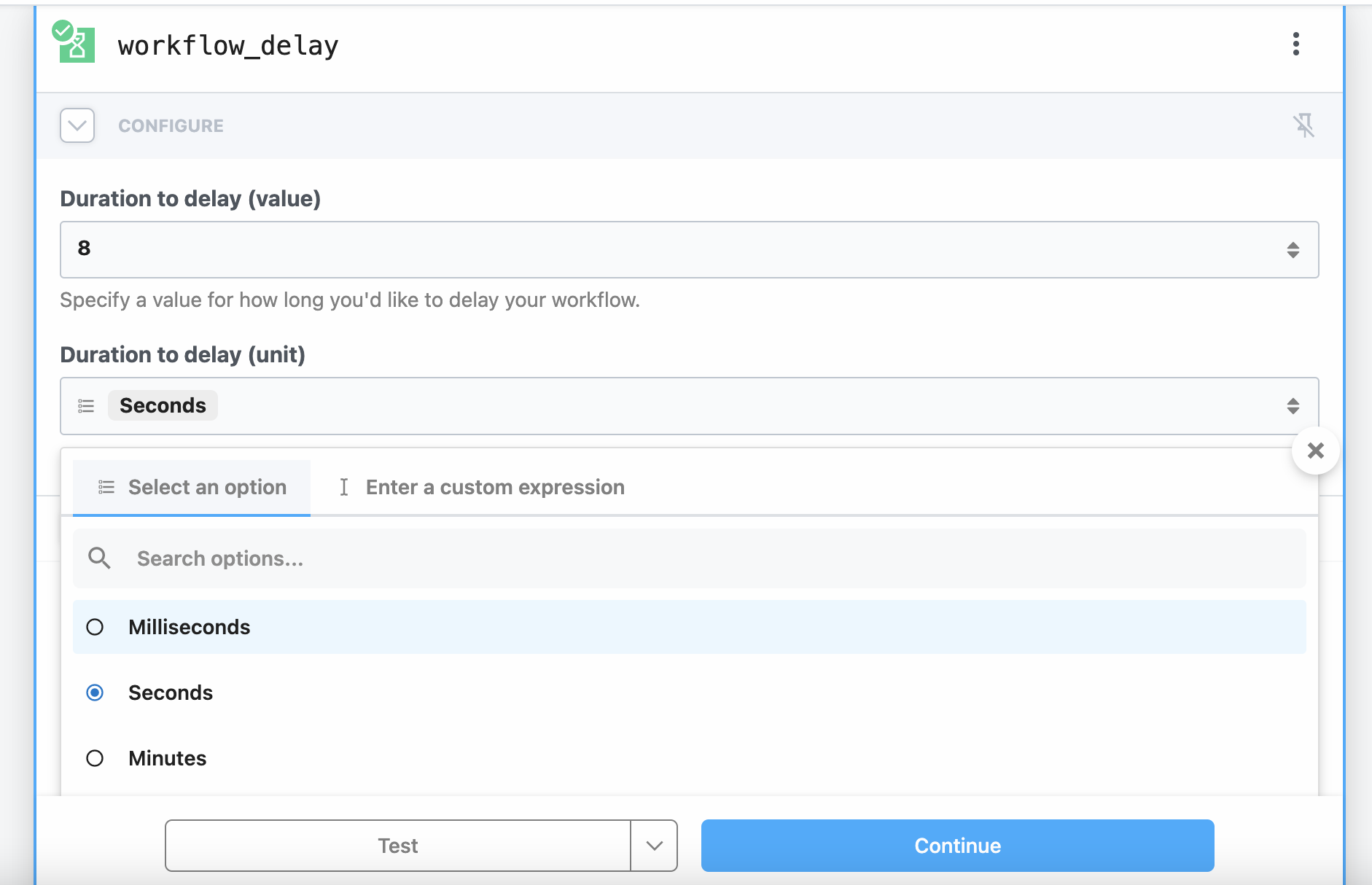
Let's press continue.
Next we'll add an HTTP PUT request module, here you'll add the following url:
https://api.landbot.io/v1/customers/{{steps.trigger.event.body.id}}/assign_bot/XXXXXX/
Make sure that {{steps.trigger.event.body.id}} is correctly pointing to the ID you sent in the trigger
You'll also need the bot ID (this is the ID in the url of your bot), and the block ID of the condition (see photo below)
We'll need two keys, launch true and node XXXXX:
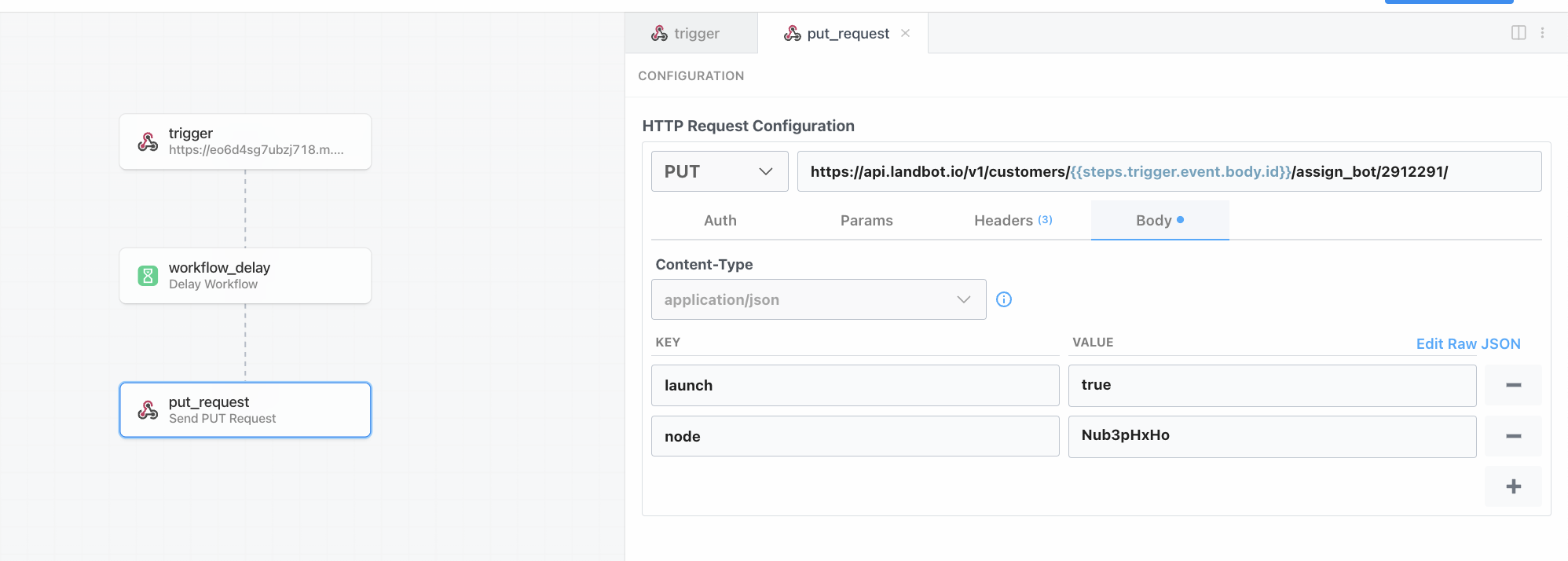
For the block ID you'll add the key launch and the value will be the Conditions block ID
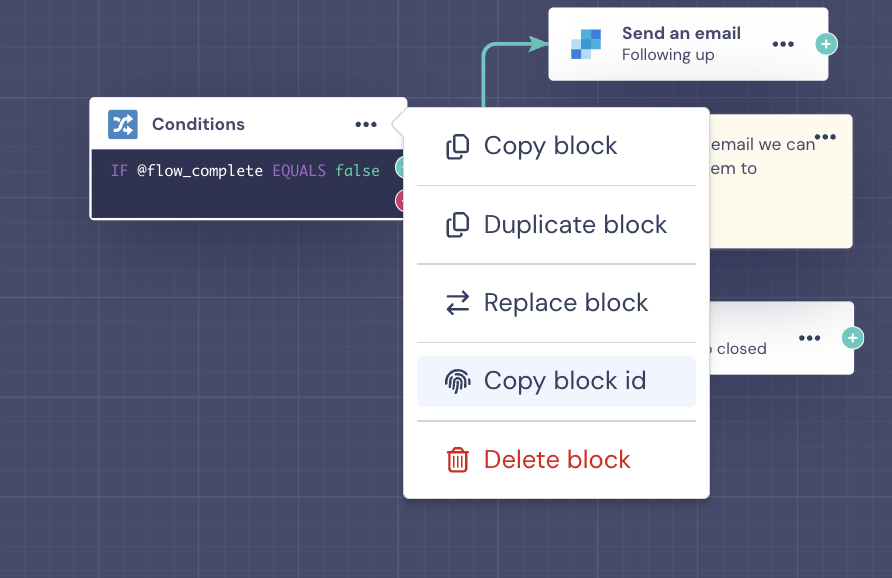
You'll add the bot ID to the end of the url (https://api.landbot.io/v1/customers/{{steps.trigger.event.body.id}}/assign_bot/BOT_ID_HERE/):

The last step is to add the headers, you will need your API key for this:
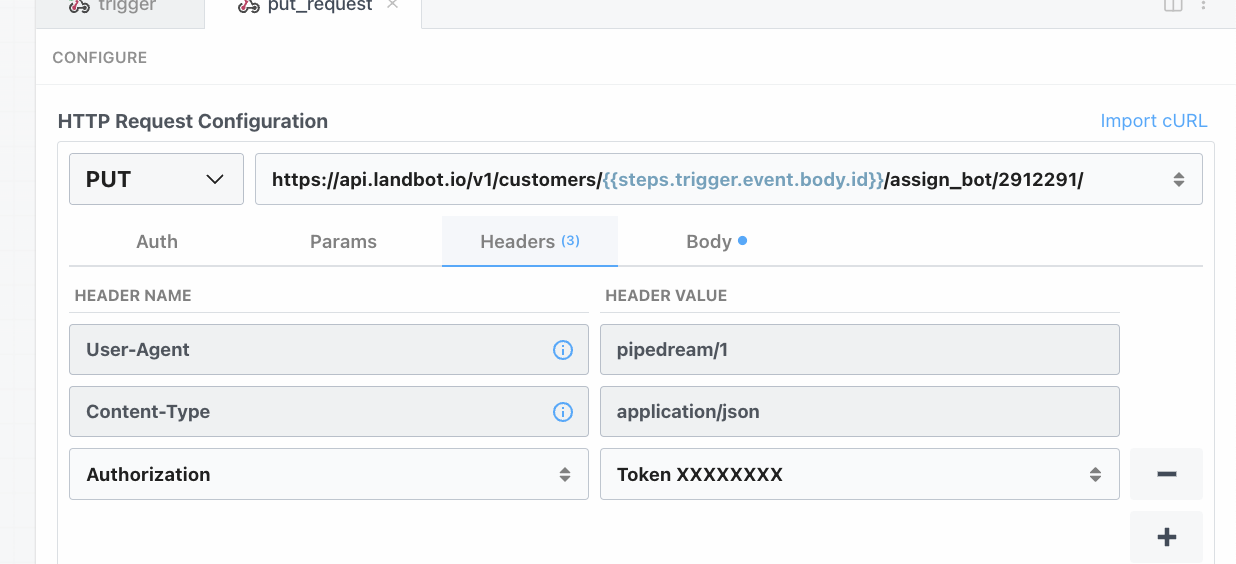
Trigger Event with Zapier
If a user abandons a chat there are several actions we can take to get them to re-engage.
Here we will use Zapier, where we will set a delay to a custom amount of time, then after that time has passed we will launch the bot assigning the user to a conditions block, this conditions block will check if the variable 'flow completed' is false, if so we have several options, in this example we'll add a 'Send an HSM' block after the condition 'flow completed' has been verified
In order to follow up with WhatsApp the user must be opted-in, to do this you can simply include an opt-in block at the beginning of the flow - if the users are already opted-in you don't need to take any further action
The flow:
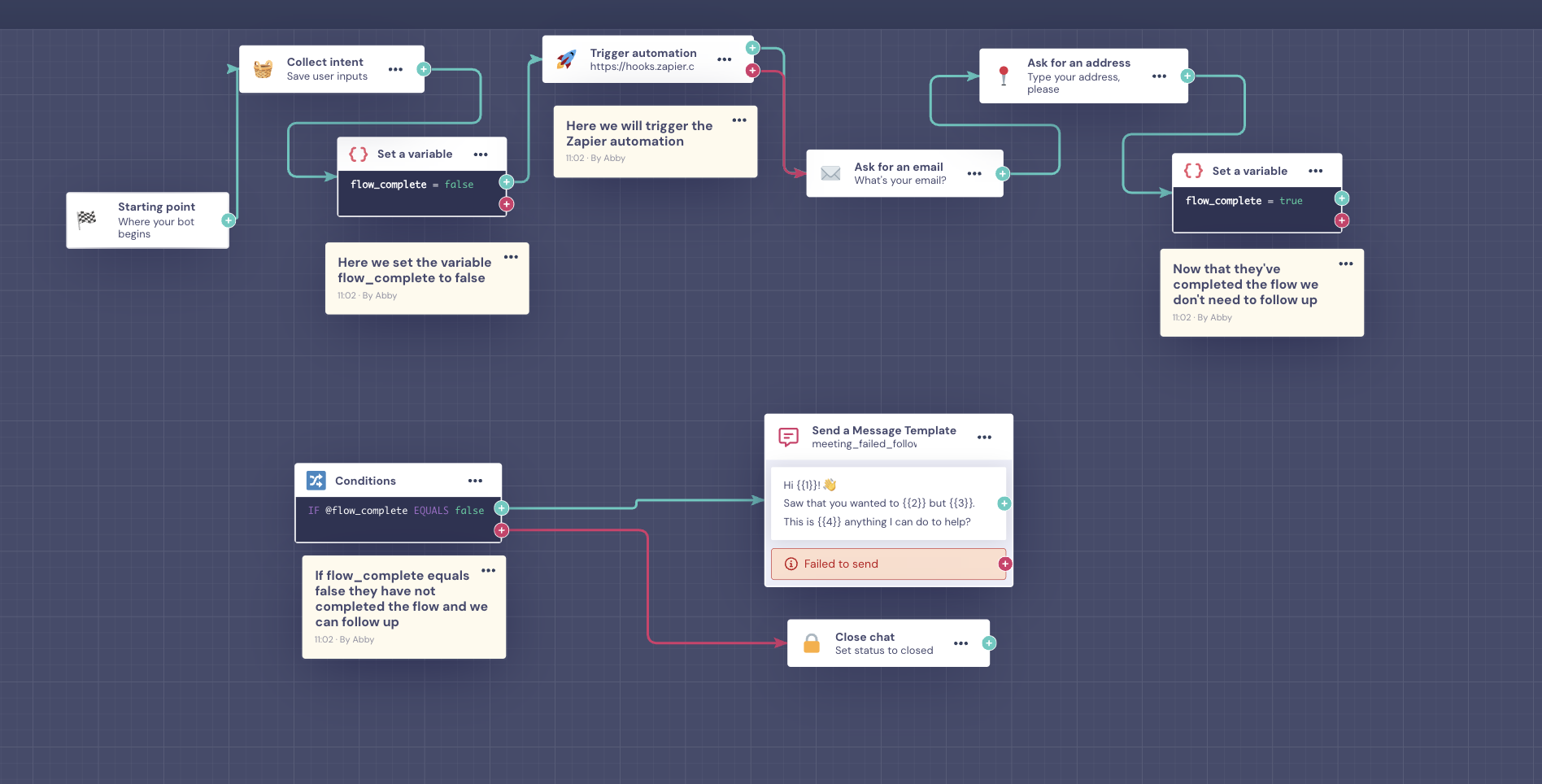
At the beginning of our flow we have the variable 'flow_complete' that equals false, if you change the name of this variable you will need to change condition block as well
At the end of our flow we will change 'flow_complete' to true, this means we won't send any follow up if they reach this point, we will simply close the chat
Zapier:
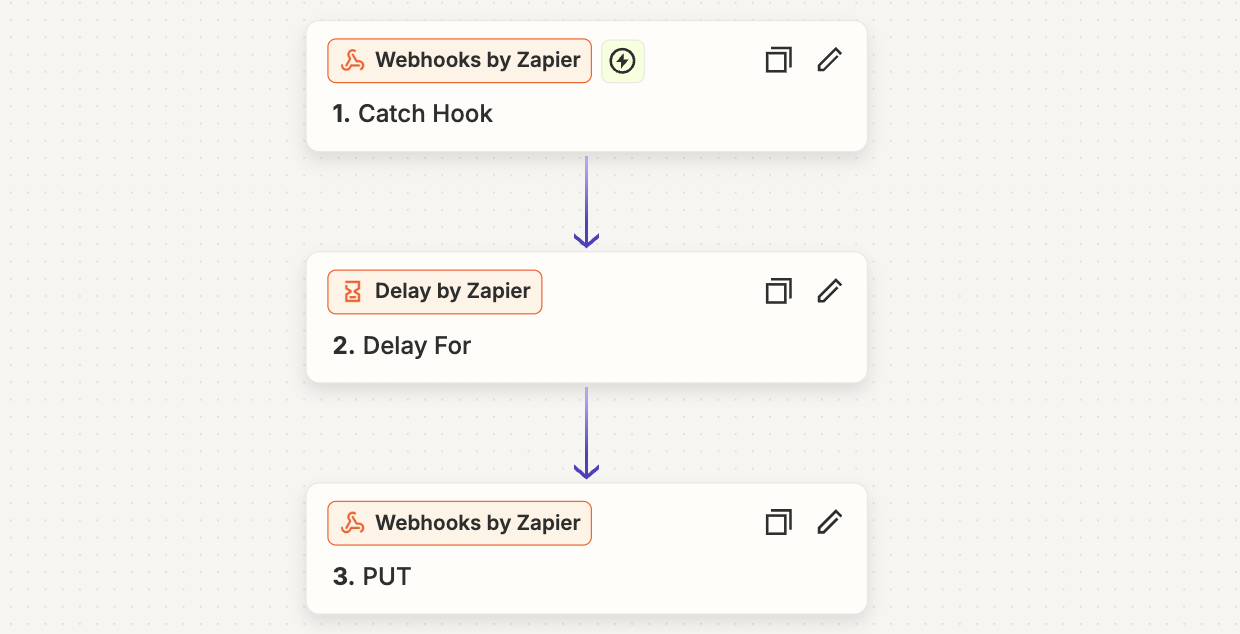
The first module we use here is a webhook, this takes in the user ID from Landbot (we'll get to that later)
This is what the configuration will look like:
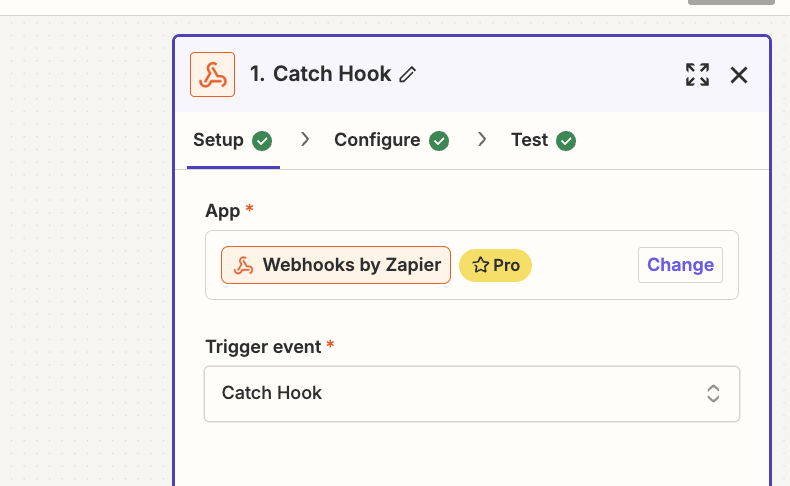
Next we'll copy the unique URL that it gives us:
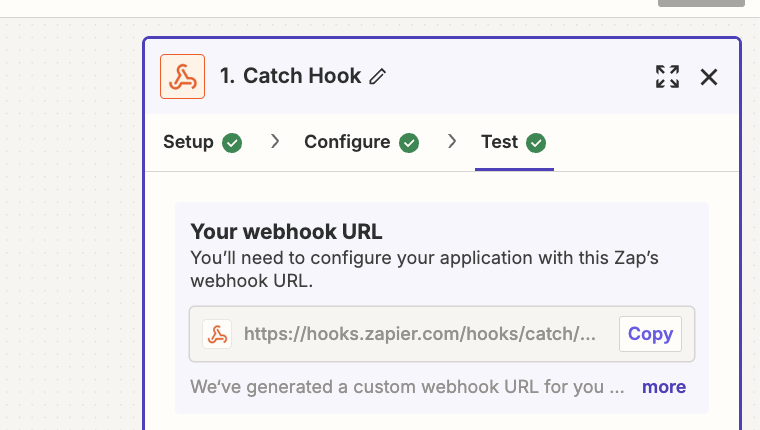
Let's go back to the bot, we'll add a Trigger Automation block with the following test values:
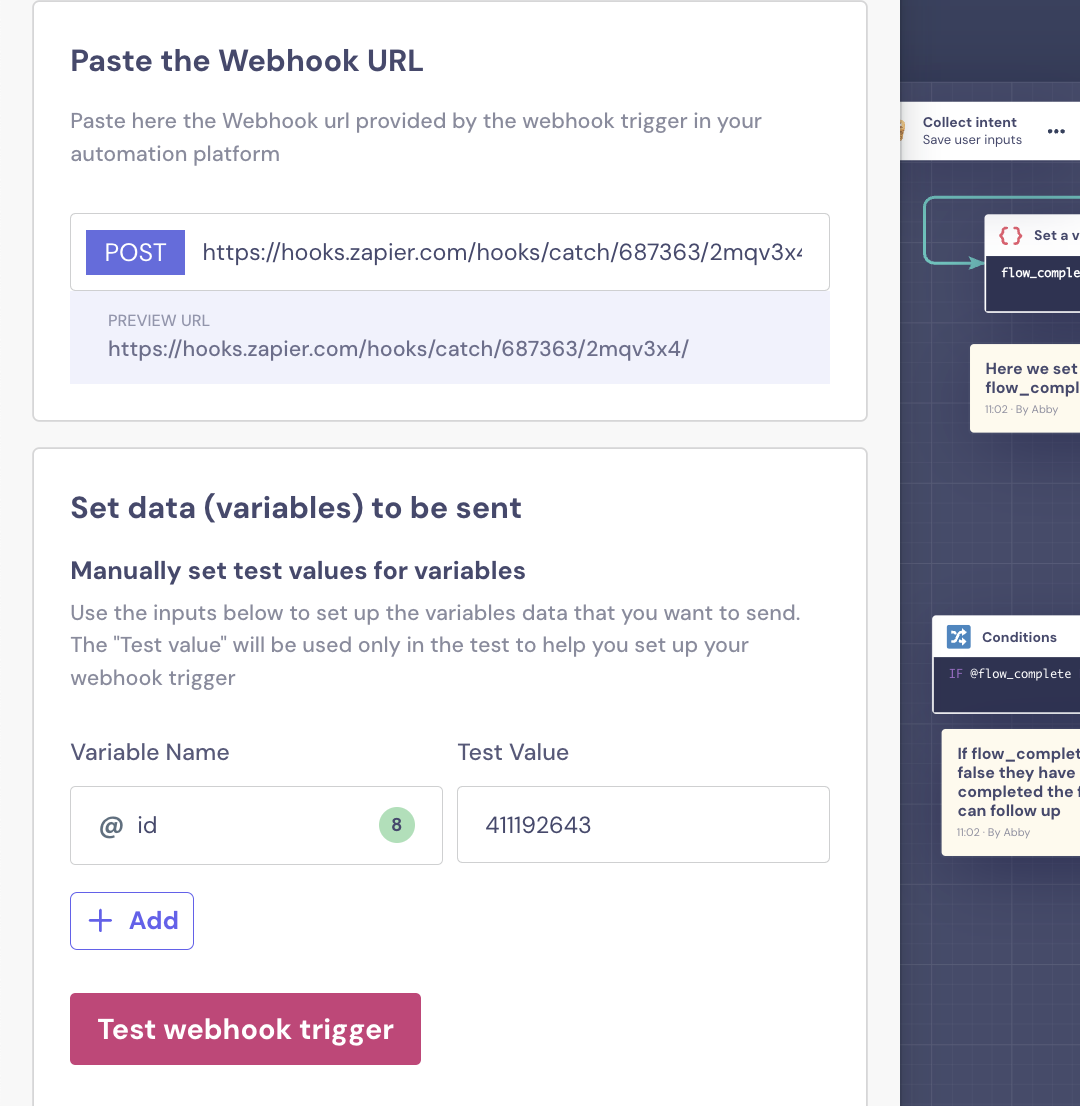
We'll test this using 'Test webhook trigger' just to be sure we've got the right URL, it should return a 200 in Landbot, and the results should look like this in Zapier:
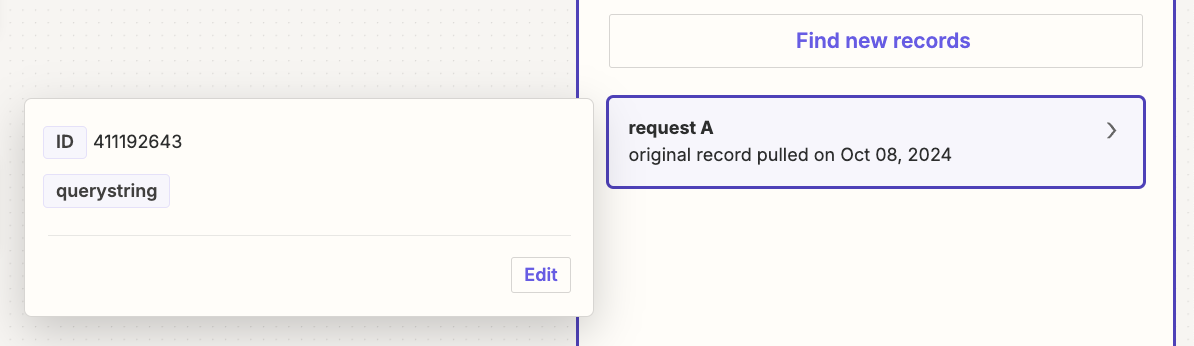
Let's press continue
Next we'll add the delay by Zapier module, then we select 'delay for' we can select minutes, or hours. I've selected 23 hours for the bot in production, however for testing I've selected 2 minutes
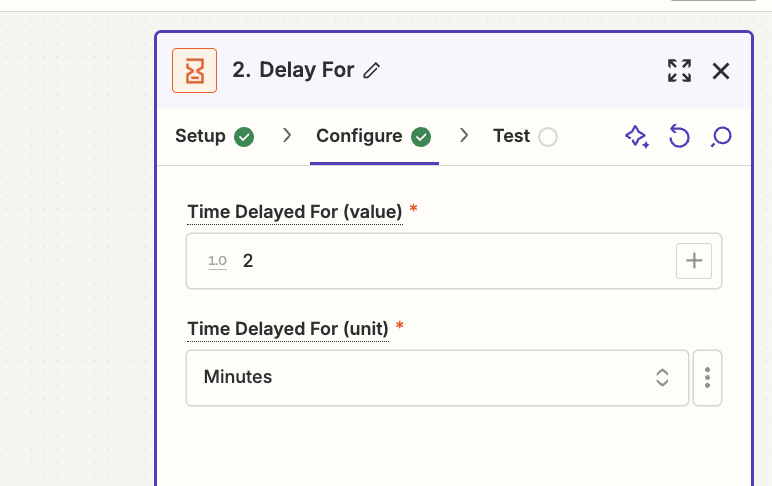
Let's press continue and test this step.
Next we'll add another webhook module, this time we'll select PUT
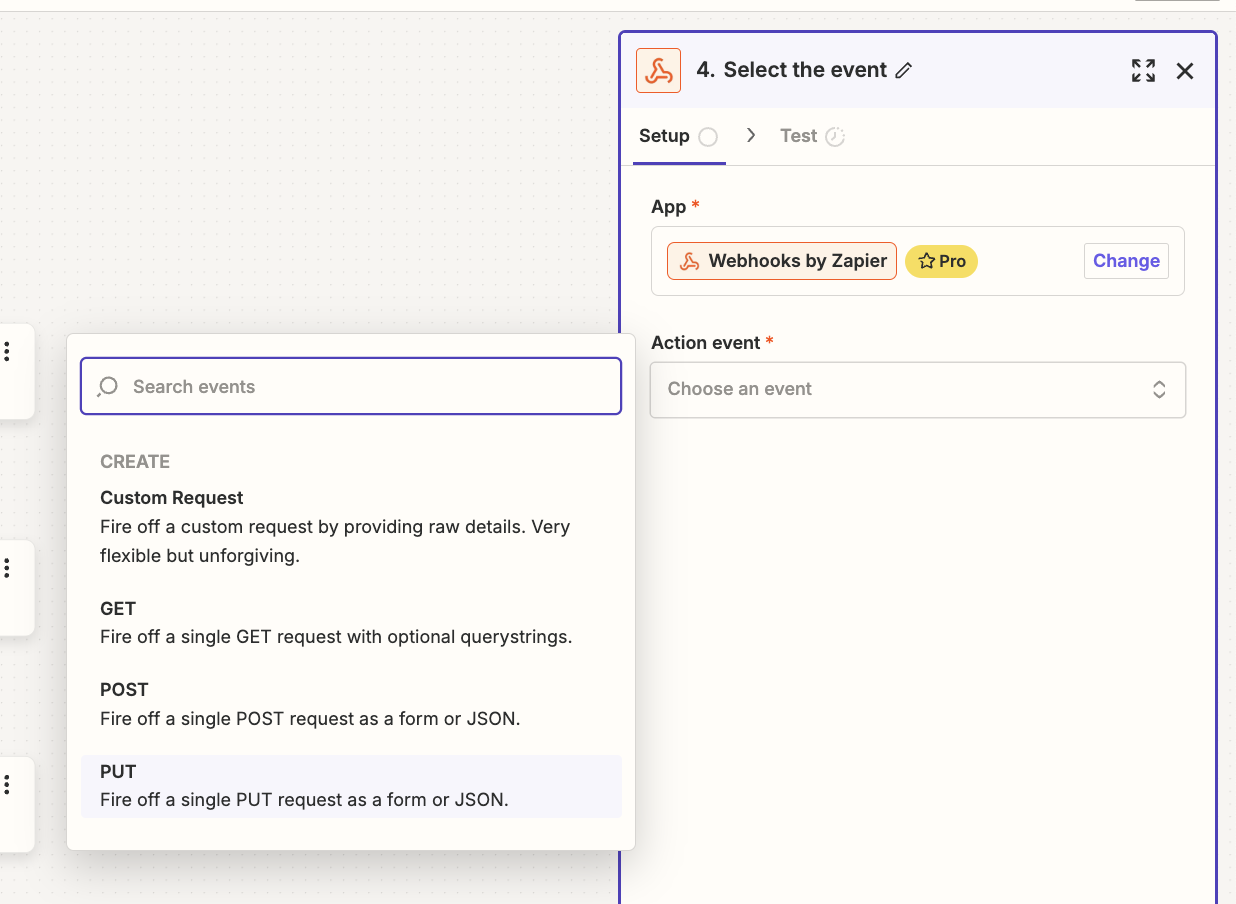
here you'll add the following url:
https://api.landbot.io/v1/customers/XCUSTOMER IDX/assign_bot/XBOT IDX/
Make sure that XCUSTOMER IDX is correctly pointing to the ID you sent in the trigger, you can click on the plus icon and add it from the 'catch hook' step:
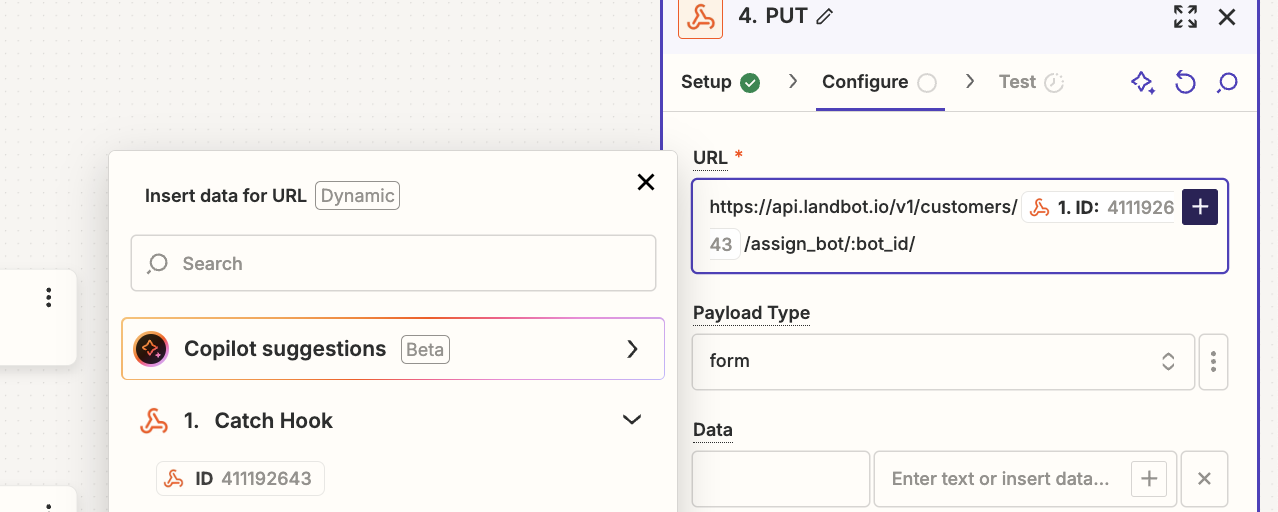
You'll also need the bot ID (this is the ID in the url of your bot), and the block ID of the condition (see photo below)
We'll select payload type JSON, then we'll add two data value sets, launch true and node XXXXX:
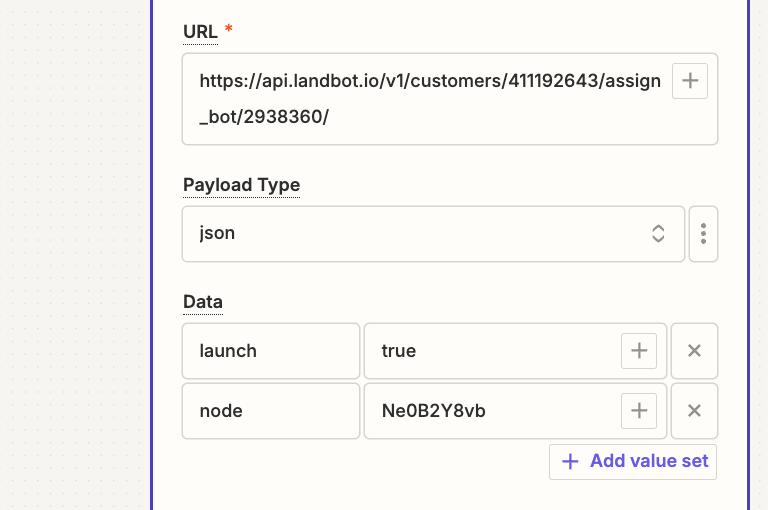
For the block ID you'll add the key launch and the value will be the Conditions block ID
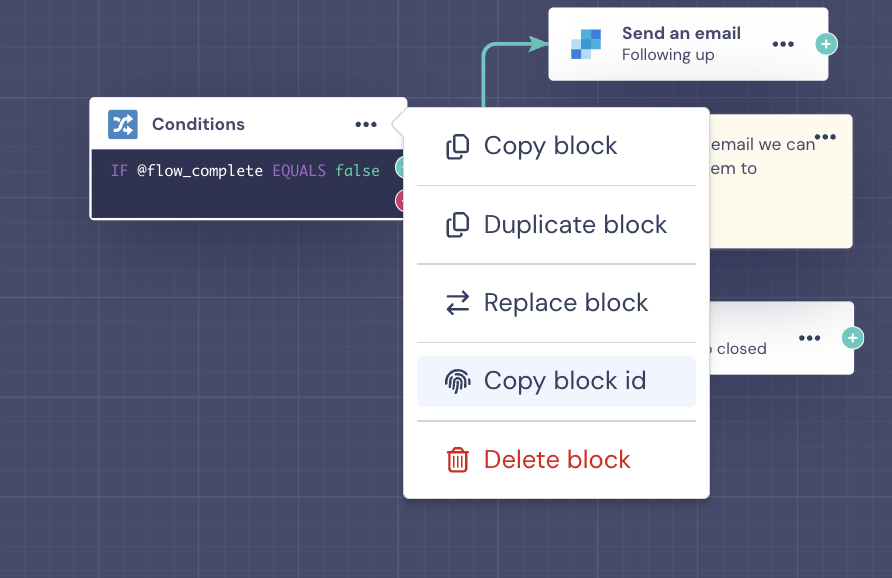
You'll add the bot ID to the end of the url (https://api.landbot.io/v1/customers/XCUSTOMER_IDX/assign_bot/BOT_ID_HERE/):

The last step is to add the headers, you will need your API key for this:
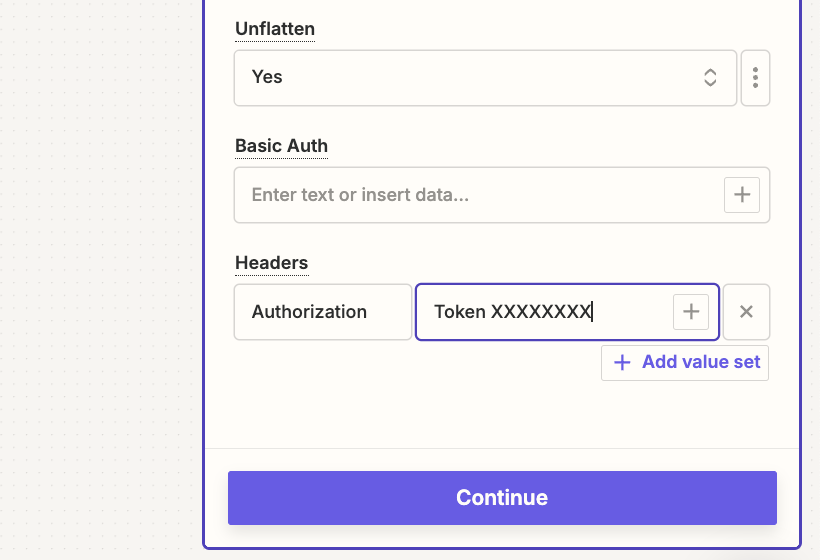
Alternative: Node.JS (Pipedream)
If you would like to do more custom actions you can use the below workflow, this involves code and you will likely need a developer or someone technical to help you set it up
In this example, we wait 48 hours after the chat has been initiated, then use Pipedream to check the Landbot API to see if the variable we added for 'flow completed' is false, if so we send a follow up message with an HSM template
However, if we set the timer to 23 hours (within the 24 hour window) you could also assign them to a webhook block to create a ticket in Zendesk or Intercom, or simply send a message without the need to use HSM
The flow:
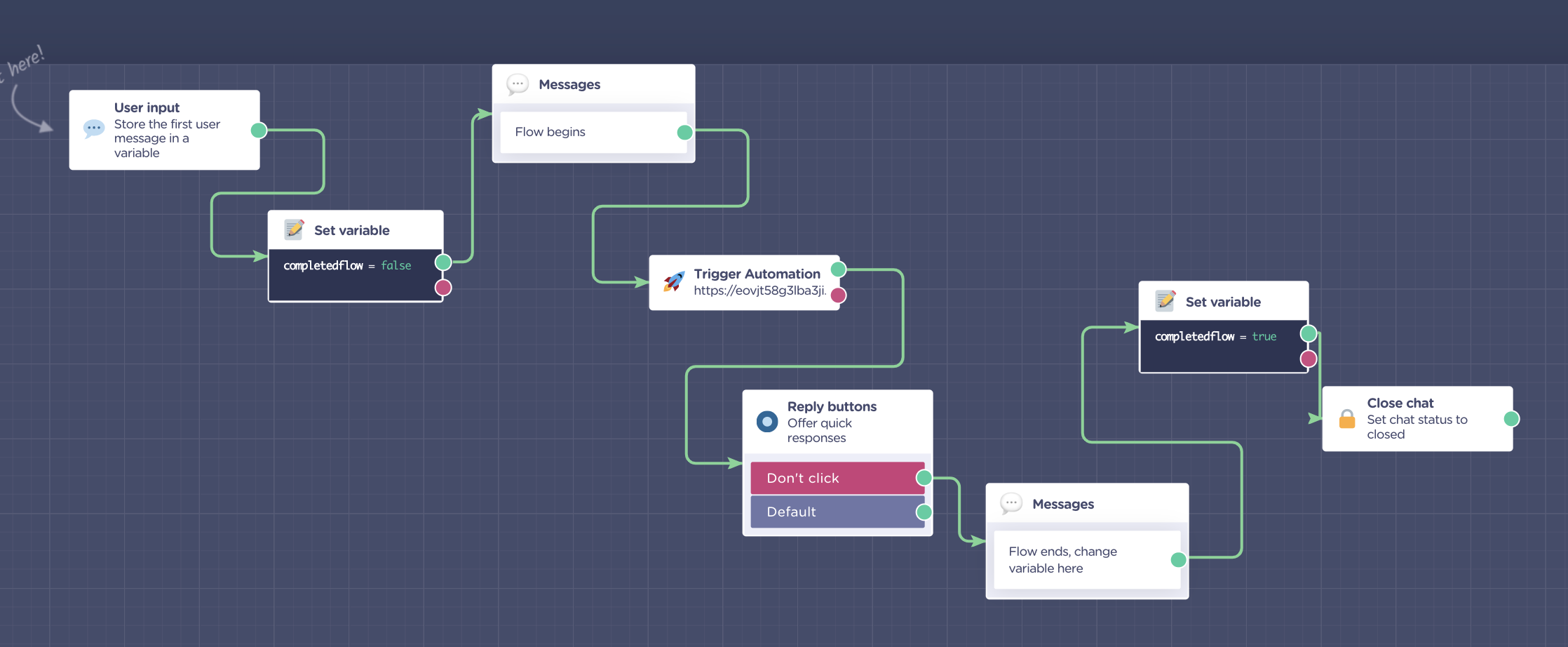
We can make our flow as complicated, or as simple as we'd like, the important things here are the variables and the Trigger Automation block
At the beginning of our flow we need a variable 'completedflow' that equals false, if you change the name of this variable you will need to change the code in Pipedream, so unless you have a developer it's not advised to change the name here
At the end of our flow we will change 'completedflow' to true, this means we won't send any follow up if they reach this point
Let's go to Pipedream now (you can use this Pipedream template to get started)
Pipedream:
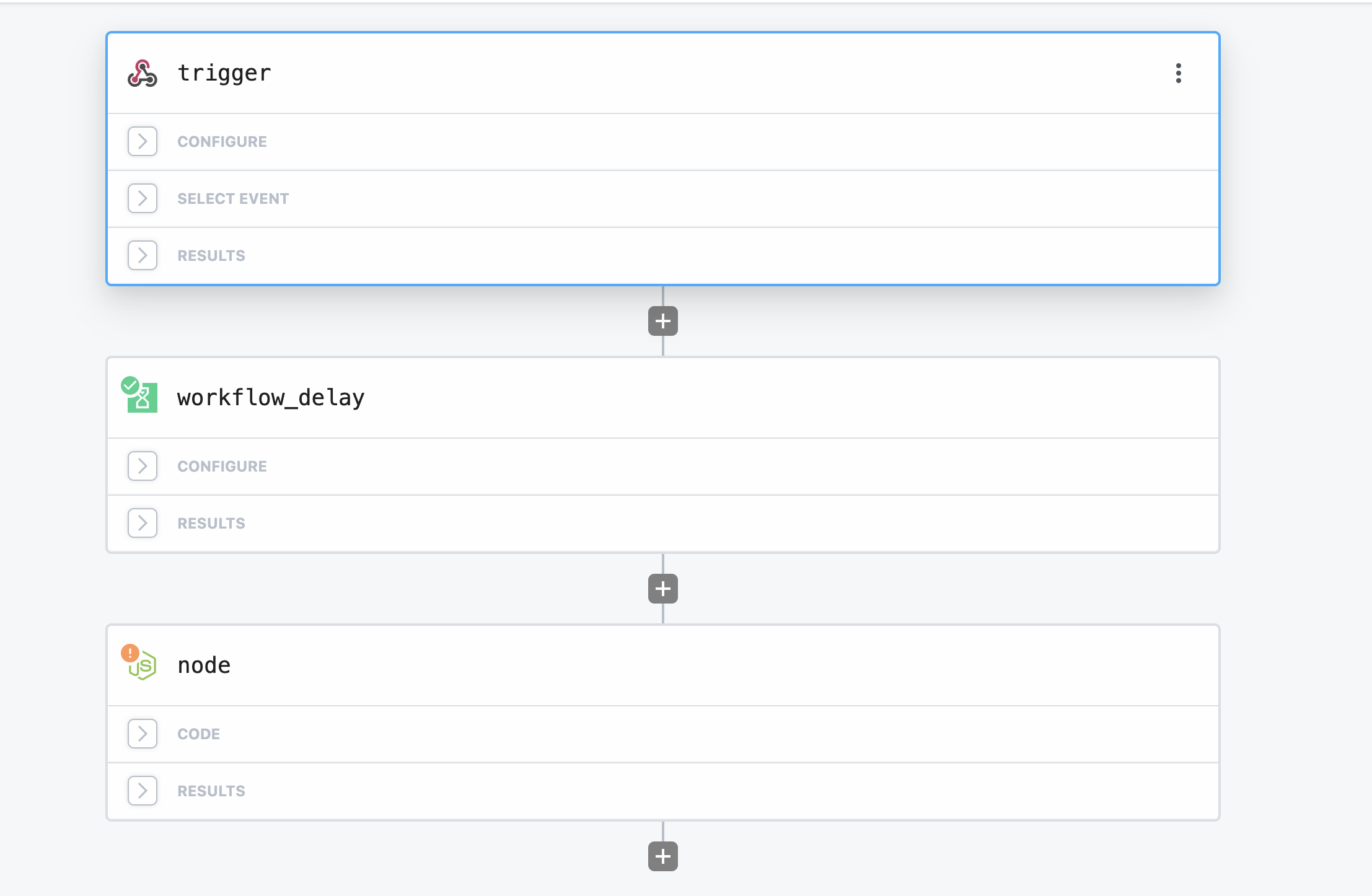
The first module we use here is a trigger, this takes in the user ID from Landbot (we'll get to that later)
This is what the configuration will look like:
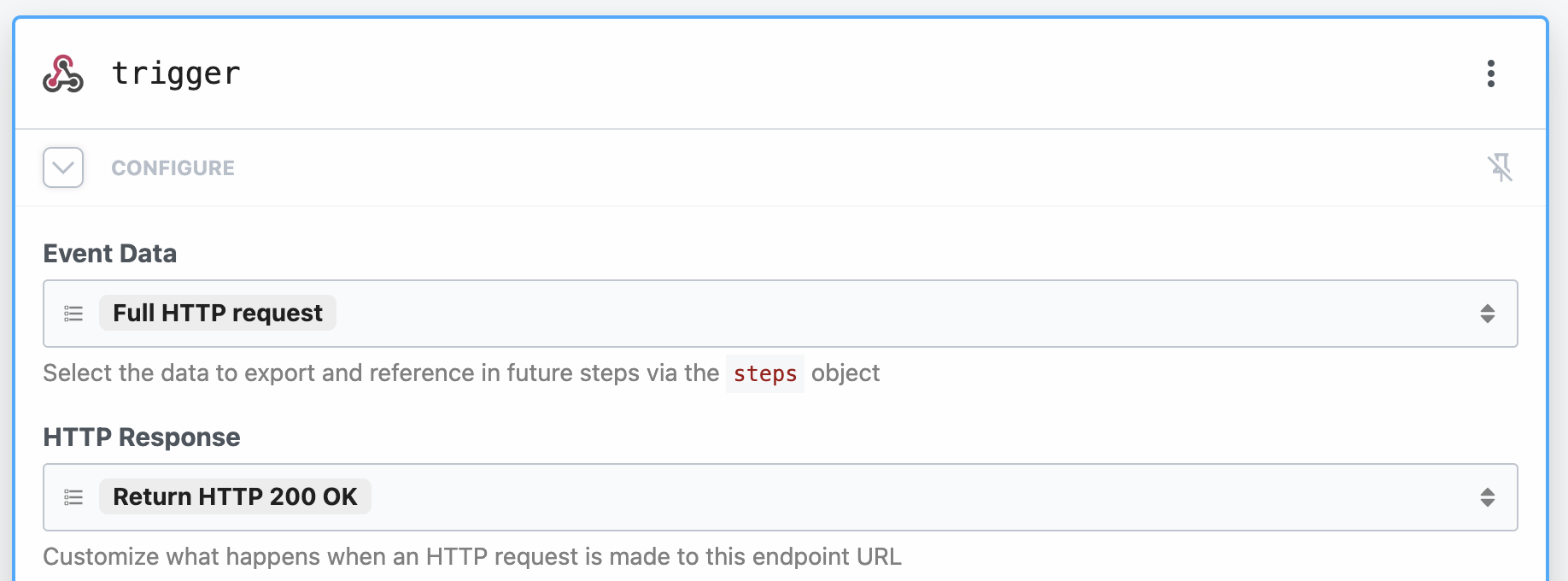
Next we'll copy the unique URL that it gives us:
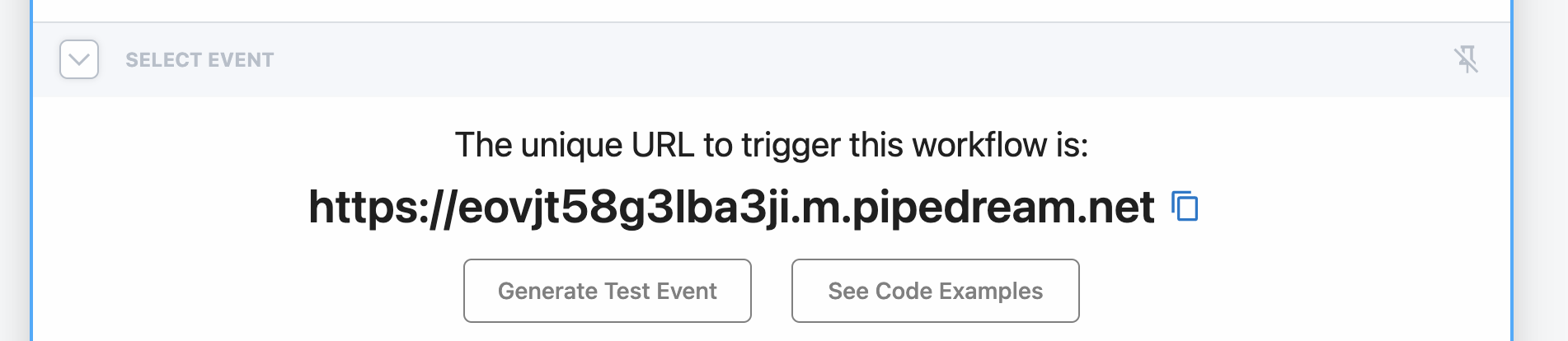
Let's go back to the bot, we'll add a Trigger Automation block with the following test values:
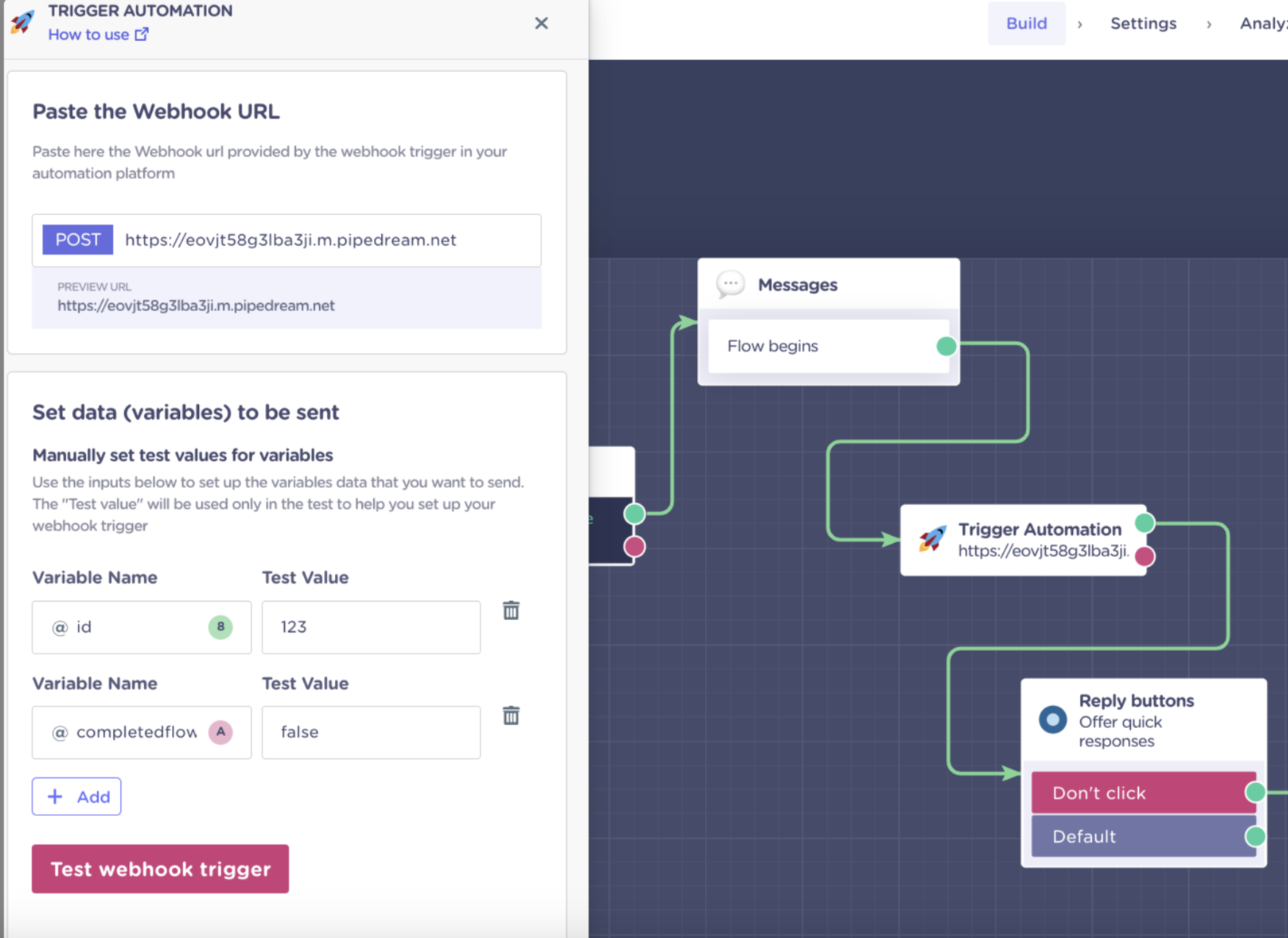
We'll test this using 'Test webhook trigger' just to be sure we've got the right URL, it should return a 200 in Landbot, and the results should look like this in Pipedream:
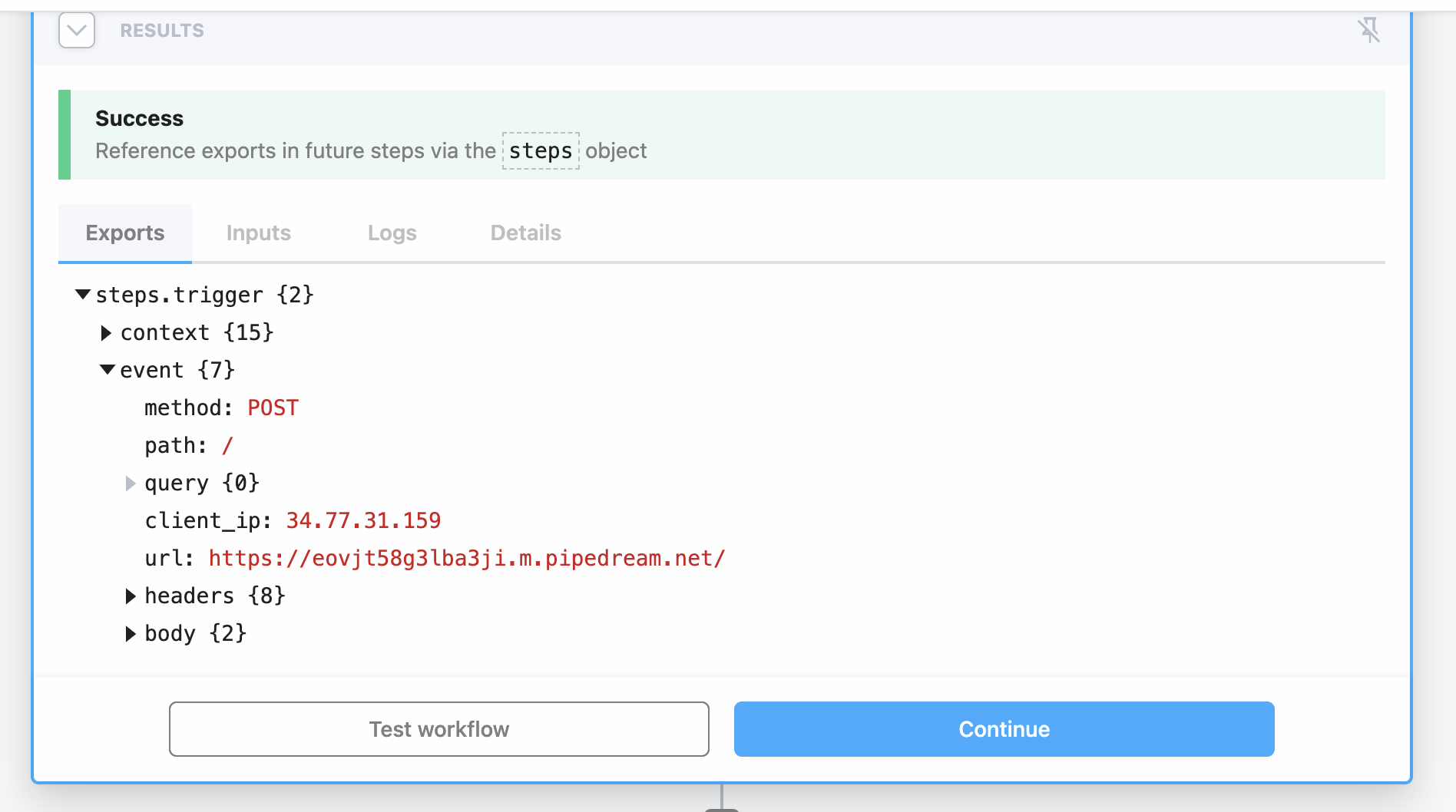
Let's press continue
Next we'll add the workflow_delay module, we can select seconds, minutes, or hours. I've selected 48 hours for the bot in production, however for testing I've selected 8 seconds
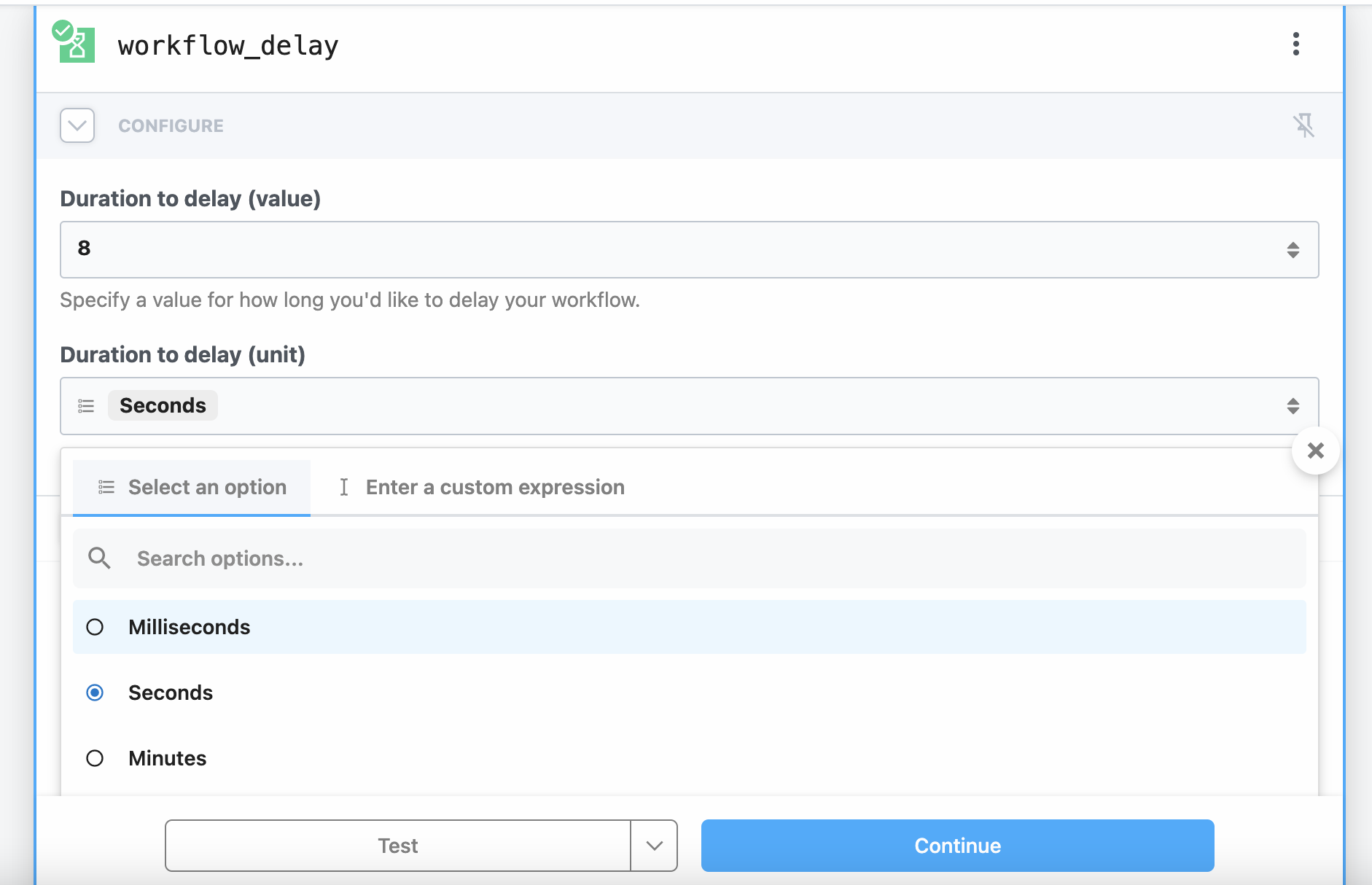
Let's press continue.
Next we'll add a Node.JS module, here you'll need to add your own API Token where you see XXXXXX, and change the HSM template ID for the one you choose to send.
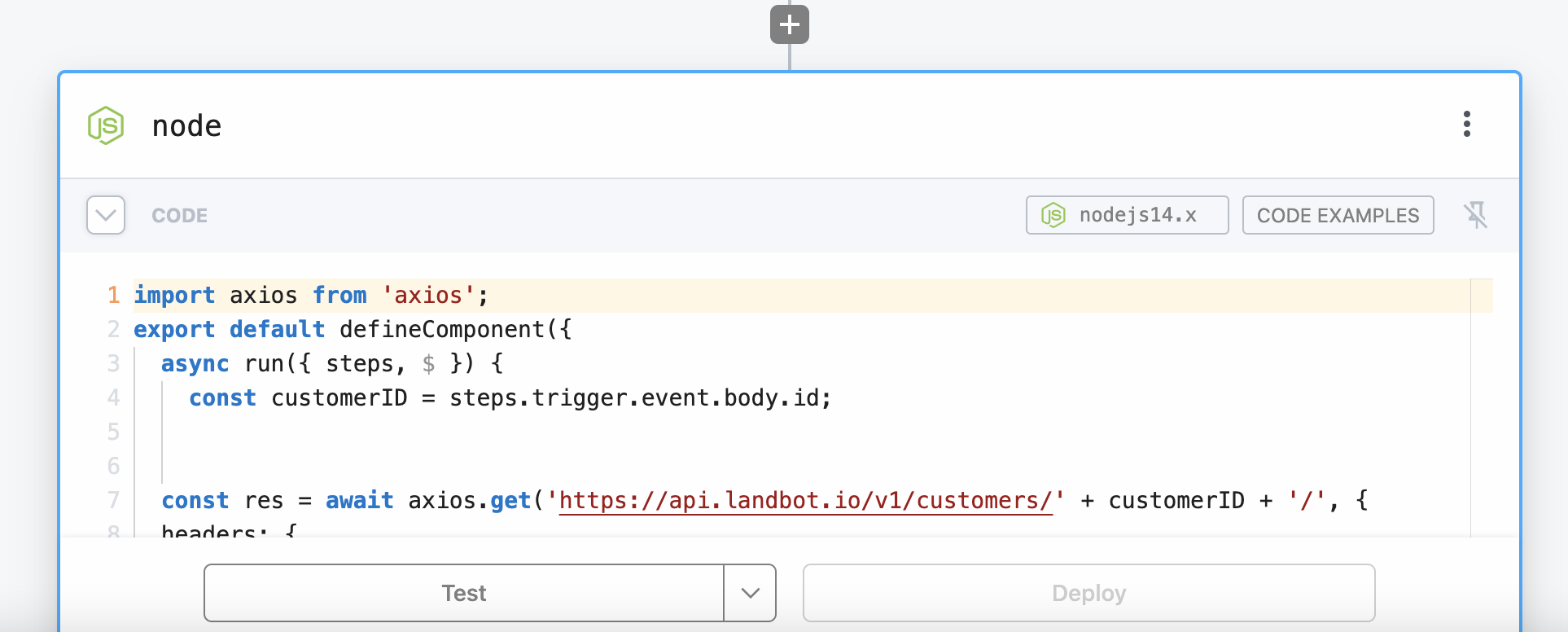
You can paste the following code in the Node module to send a Template:
import axios from 'axios';
export default defineComponent({
async run({ steps, $ }) {
const customerID = steps.trigger.event.body.id;
const res = await axios.get('https://api.landbot.io/v1/customers/' + customerID + '/', {
headers: { 'Authorization': 'Token XXXXXXXXXXXXXX' }});
let completedFlow = res.data.customer.completedflow;
console.log(completedFlow);
if(completedFlow == 'false'){
let auth = 'XXXXXXXXXXXXXXXXXX';
const options = {headers: {'Content-Type': 'application/json','Authorization': 'Token ' + auth}};
return await axios.post(`https://api.landbot.io/v1/customers/${customerID}/send_template/`, {"template_id": 3424,"template_params": { "header": { "params": [] }, "body": { "params": [] }, "buttons":[ { "params": [] } ] },"template_language": "en"} , options)
}
else{
}
}
})
Let's test, deploy and start using our workaround!
In addition to send a Template, you can perform other actions using the Landbot API:
- In case you need just to Send a Text, use this code instead:
import axios from 'axios';
export default defineComponent({
async run({ steps, $ }) {
const customerID = steps.trigger.event.body.id;
const res = await axios.get('https://api.landbot.io/v1/customers/' + customerID + '/', {
headers: { 'Authorization': 'Token XXXXXXXXXXXXXX' }});
let completedFlow = res.data.customer.completedflow;
console.log(completedFlow);
if(completedFlow == 'false'){
let auth = 'XXXXXXXXXXXXXXXXXX';
const options = {headers: {'Content-Type': 'application/json','Authorization': 'Token ' + auth}};
return await axios.post(`https://api.landbot.io/v1/customers/${customerID}/send_text/`, { "message": "Hello" } , options)
}
else{
}
}
})
- If you'd like to Close the Conversation you can use this snippet:
import axios from 'axios';
export default defineComponent({
async run({ steps, $ }) {
const customerID = steps.trigger.event.body.id;
const res = await axios.get('https://api.landbot.io/v1/customers/' + customerID + '/', {
headers: { 'Authorization': 'Token XXXXXXXXXXXXXX' }});
let completedFlow = res.data.customer.completedflow;
console.log(completedFlow);
if(completedFlow == 'false'){
let auth = 'XXXXXXXXXXXXXXXXXX';
const options = {headers: {'Content-Type': 'application/json','Authorization': 'Token ' + auth}};
return await axios.put(`https://api.landbot.io/v1/customers/${customerID}/archive/`, options)
}
else{
}
}
})
- If you want to Assign the customer to a specific point in the bot flow you can use this snippet:
import axios from 'axios';
export default defineComponent({
async run({ steps, $ }) {
const customerID = steps.trigger.event.body.id;
const bot_id = XXXXX;
const block_id = XXXXX;
const res = await axios.get('https://api.landbot.io/v1/customers/' + customerID + '/', {
headers: { 'Authorization': 'Token XXXXXXXXXXXXXX' }});
let completedFlow = res.data.customer.completedflow;
console.log(completedFlow);
if(completedFlow == 'false'){
let auth = 'XXXXXXXXXXXXXXXXXX';
const options = {headers: {'Content-Type': 'application/json','Authorization': 'Token ' + auth}};
return await axios.put(`https://api.landbot.io/v1/customers/${customerID}/assign_bot/${bot_id}/`, { "launch": true, "node": block_id} }, options)}
else{
}
}
})