Build a bot
Getting started
Basics and builder
Fields in Landbot - Getting Started
Builder's keyboard shortcuts and mechanics! 🏗
Builder Interface
How to "debug" (troubleshoot) your bot's flow to spot possible errors (for non coders)
Starting Point
How to disable a bot
Bricks
Bot's Settings
Bot General Settings
Second Visit Settings
Custom System Messages
Hidden Fields (Get params / UTMs from url and use it as variables)
Landbot native SEO & Tracking tools
Typing Emulation (Message Delay)
Build it for me!
Getting started - build a bot
Messages, Questions and Logic & Technical blocks
Messages
Media Block
Media block
How to display images with a variable URL source
How to embed a .gif file inside a message
Different ways to embed Videos in Landbot
Display video and hide button to continue until video has ended
Send a Message block - Simple Message
Goodbye block
Question blocks
Date Block
Email Block
Form Block
Scale Block
Buttons block
How to set up Multiple Choice questions
Multi-Question block
Question: Address block
Question: Autocomplete block
Question: File block
Ask for a name
Question: Number block
Question: Phone block
Question: Picture Choice block
Question: Rating block
Question: Text block
Question: URL block
Question: Yes/No block
Logic & Technical blocks
Code Blocks
Dynamic Data
Dynamic Data (Arrays)
Get the array's index of the user selection and extract information from array
Formulas
How to Perform Basic Calculations
Get started with the Formulas block
Formulas Blocks Dashboard
Formulas - Regex
Formulas - Date
Formulas - String
Formulas - Logical
Formulas - Math
Formulas - Object
Formulas - Comparison
Formulas - Array
Persistent Menu
Trigger Automation
Webhook
Webhook Block Dashboard
Webhook block (External API REST)
How to TEST your Http Request (Webhook block)
Landbot System Variables
Set a Field block
Any of the above Output
Global Keywords 🌍
Keyword Jump
Lead Scoring block
Jump To block
AB Test
Conditions block
Conditions block II (with Dates, Usage and Agents variables)
Close Chat block
How to ask a question based on a variable not being set (empty URL params)
Business Hours block
Custom Goals
Note block
Share & Embed
Redirect Users
How to open a new URL in another tab (window)
How to redirect visitors to a URL (web only)
Conditional Welcome (Non-embedded bots)
How to add a Click-to-Call/Email/WhatsApp button
Redirect User Based on Language Input (DeepL)
Generate a URL that has variables from user answers
Popup on Exit Intent
Share
Customized Embed Actions
How to redirect user to another url in your site with Livechat open to continue conversation
How to Detect Visitors Browser
Customize and embed your WhatsApp Widget
Modifying Embed Size
Conditional Welcome: Direct the Flow of Your Bot Based on External Parameters
Detect if bot was opened
Customized Behavior in Mobile Browsers
Load script and display bot on click button
Launch Bot On Exit Intent
Display Bot During Business Hours Only (Livechat & Popup)
Open / Close a Web bot (embedded)
Launching a bot depending on browser language
How to pass WordPress logged in user data to Landbot
Set the flow depending on the url path (for embedded landbots)
How to launch a Landbot by clicking a button
Open LiveChat bot as soon as page loads
Detect if a visitor is on Mobile/Tablet or Desktop
Embed
Embed the bot into your Website
Embed Landbot in an iframe
Landbot in Wix
Landbot in your web with Google Tag Manager
Landbot in Webflow
Embed in Sharetribe
Landbot in Shopify
Embedding Landbot in Carrd
Landbot in Wordpress
Landbot in Squarespace
Customizing the Proactive Message
Design section (web bots)
Verification & Security
Account Settings and Billing
Billing
Account Settings
Account Settings
Account Homepage
Dashboard
Common reasons for not receiving account activation email
Privacy and Security
Landbot's Privacy & Security Policies
Compliance with the GDPR - General Data Protection Regulation
Landbot's Abuse Policy - How to Report an Abusive Use of Landbot
Delete Customer Data in Flow
Teammates
Agent Status and Log out
Roles & Permissions for Teammates
Teammates - Add extra agents (seats) to your Account
Notifications section
Startup Discounts
NGOs and Educational Organizations Discount
Human Takeover & Inbox
Integrations with Landbot
Native Integrations
Airtable
Airtable integration block
Get data filtered from Airtable with a Brick- Shop example
20 different ways to GET and filter data from Airtable
How to add/update different field types in Airtable (POST, PATCH & PUT)
How to Create, Update, Retrieve and Delete records in Airtable (POST, PATCH, GET & DELETE)
Get more than 100 items from Airtable
Insert Multiple Records to Airtable with a Loop
How to Get an Airtable Token
Advanced filters formulas Airtable block
Airtable usecase: Create an event registration bot with limited availability
Update Multiple Records in Airtable Using a Loop
Reservation bot with Airtable
Calendly
Dialogflow
Dialogflow & Landbot course
Dialogflow & Landbot intro: What is NLP, Dialogflow and what can you do with it?
Dialogflow & Landbot lesson 1: Create your first agent and intent in Dialogflow
Dialogflow & Landbot lesson 2: Get the JSON Key
Dialogflow & Landbot lesson 3: Setting up of Dialogflow in Landbot
Dialogflow & Landbot lesson 4: Training phrases and responses for a FAQ
Dialogflow & Landbot lesson 5: Entities and Landbot variables
Dialogflow & Landbot lesson 6: Redirect user depending on Dialogflow response parameters (intent, entities and more)
Learn more about Dialogflow - Courses and communities
Integrations > Dialogflow Block
How to extract parameters from Dialogflow response with Formulas
Dialogflow Integration Dashboard
Dialogflow in Unsupported Languages (& Multilingual)
Dialogflow - How to get JSON Key
Google Sheets
Google Sheets (Insert, Update and Get data)
How to use Google Sheets to create a simple verification system for returning visitors
How to Upload Document & store link
How to give unique Coupon Codes (with Google Spreadsheets)
Google Sheets Integration Dashboard
How to insert a new row with data and formulas in Google Sheets
How to use Google Spreadsheet as a Content Management System for your bot
Hubspot
MailChimp
Salesforce
Segment
SendGrid
Slack
Stripe
Zapier
How to Configure the Landbot and Zapier Integration Using the Zapier Block
Zapier Integration Dashboard
How to insert a row to Google Spreadsheet by Zapier
How to generate a document with PDFMonkey by Zapier
Send WhatsApp Message Templates from Zapier
How to send an email with Gmail by Zapier
Get Opt-ins (Subscribers) from Facebook Leads using Zapier
How to extract data from an external source with Zapier and use it in Landbot
Zapier trigger
How to complete a digital signature flow by Zapier
Make a survey with Landbot and display the results in a Notion table using Zapier
Zendesk
Non-Native Integrations
ActiveCampaign
Google Calendar
Google Fonts
Google Maps
Embed Google Maps
Google Maps API Key for Address block
Extract Data With Google Maps Geocoding API
Calculate Distances With Google Maps API
Google Meet
IFTTT
Integrately
Intercom
Make
Connecting MySQL with Make.com (formerly Integromat)
How to upload a file to Google Drive using Make.com (formerly Integromat)
Send WhatsApp Message Template from Make (ex Integromat)
Make Integration With Trigger Automation Block
How to send an email through Sendinblue by Make.com (formerly Integromat)
Get Opt-ins (Subscribers) from Facebook Leads using Make (ex Integromat)
How to extract data from an external source with Make.com and use it in Landbot
OCR
Pabbly
Paragon
Pipedream
PDF Monkey
Store Locator Widgets
Xano
Zendesk
WhatsApp Channel
Getting started!
WhatsApp Testing
Build a WhatsApp Bot - Best Practices and User guide
Build a WhatsApp Bot - Best Practices for Developers
Types of Content and Media you can use in WhatsApp 🖼
1. WhatsApp Article Directory
2. WhatsApp Integration FAQ's
Adding & Managing your WhatsApp Channel
Facebook Business Verification - Best Practices 🇬🇧
WhatsApp Number Deletion (WA Channel management)
Adding a WhatsApp number to your account
WhatsApp’s Messaging Policy: New Accepted Industry verticals
Meta processes guide: FBM verification, Official Business Account (OBA) requests, Appeals
Additional Number integration: Limitations and Requirements (Number integration)
Existing WhatsApp Number Migration
Key Insights for Migrating to WhatsApp Business API Cloud
How to's, Compatibility & Workarounds
WhatsApp bots - Feature Compatibility Guide
WhatsApp - How to direct a user through a different bot flow on their second visit
WhatsApp - Get user out of error message loop
Getting Subscribers: Opt-in, Contacts
How to get Opt-ins (Contacts) for your WhatsApp 🚀
WhatsApp Quality - Best Practices
Opt-In block for WhatsApp 🚀
Opt-in Check Block
WhatsApp Channel Settings
Parent Bot/Linked Bot - Add a main bot to your WhatsApp number
WhatsApp Channel Panel (Settings)
Growth Tools for WhatsApp
Duplicating bots
Messaging and contacting your users
WhatsApp Campaigns 💌
WhatsApp's Message Templates
Audience block
WhatsApp Marketing Playbook: Best Practices for Leadgen
Audiences
WhatsApp for Devs
How to calculate the number of days between two selected dates (WhatsApp)
Creating a Loop in WhatsApp
Recognise the users input when sending a Message Template with buttons
Trigger Event if User Abandons Chat
Calculate Distances in WhatsApp
Send Automated Message Templates based on Dates
Trigger Event if User Abandons Chat Using AWS
reply from Slack: How to create an integration to allow agents reply WhatsApp users from Slack (with Node JS)
Set Up a Delay Timer in Bot
Notify Teammates of Chat via WhatsApp
Native blocks for WhatsApp
Other Channels - Messenger and APIChat
Facebook Messenger
The Facebook Messenger Ultimate Guide
Types of content you can use in Messenger bots 🖼
How to Preview a Messenger bot
Facebook Ad connected to Messenger Bot
API Chat (for Developers)
AI in Landbot
Non-native AI Features
Create a JSON format response from OpenAI in WhatsApp
Connect OpenAI Assistant with Landbot
AI in WhatsApp
How to build a FAQ chatbot with GPT-3
GPT-4 in Landbot
OpenAI
Prompt Engineering for GPT-3
Build a Customer Service Bot with ChatGPT and Extract Information
Google Gemini in Landbot
Native AI Features
Metrics and Data Management
Metrics Section
How to export the data from your bots
Export data: How to open a CSV file
Bot's Analyze Section
For Developers & Designers
JavaScript and CSS
CSS and Design Customizations
Design Customizations
Advanced (Custom CSS & Custom JS)
Components CSS Library Index
Background Class CSS
Identify Blocks CSS
Buttons Class CSS
Header Class CSS
Media Class CSS
Message Bubble Class CSS
Miscellaneous Classes CSS
CSS Customization Examples: "Back to School" Theme
Get started guide for CSS Design in Landbot
CSS Customization Examples: Call To Action: WhatsApp
CSS Examples: Lead Gen
CSS Customization Examples: "Translucid"
CSS Customization Examples: "Minimalist" Theme
Dynamic Data CSS
Form Block CSS
CSS for Typewriter Effect
CSS Customization Examples: Carrd Embed Beginner
Dynamically Change a Bot's Background
Proactive Message Customizations with Javascript and CSS
Landbot v3 - Web CSS - RTL
Multi Questions CSS
CSS Customization Examples: Video Bubble
Dynamically Change Any Style
CSS Customization Examples: CV Template
Change Landbot custom CSS dynamically from parent page onload
Widget/Bubble Customizations with Javascript and CSS
JavaScript
How to change Avatar dynamically (Landbot v3)
Javascript in WhatsApp
Javascript in Landbot v3
Different ways to format numbers with JS
How to display an HTML Table and a List in Landbot v3 web
Trigger a Global Keyword with JS (web v3)
Create Dynamic Shopping Cart with JS and CSS
Add a Chart (with Chart JS library) in your Landbot
Different ways to format numbers with JS (WhatsApp)
Pop up modal to embed third party elements
Landbot API
Send WhatsApp Messages with Landbot API
How to "send" a user to a specific point in the flow with Javascript and with the API
APIs
Create Contacts (Opt-ins) using Landbot API
MessageHooks - Landbot Webhooks
Resume flow based on external process with Landbot API (Request, Set, Go)
Tracking
Google Analytics - Track Events (Not embedded)
Google Analytics - Track Events (Embedded)
Meta Pixel - Track Events (only Embedded)
How Track Google Analytics Events in Landbot with Google Tag Manager (GTM)
Google Adwords - How to track Google Adwords in Landbot
Set a timer to get the time spent during the flow
Workarounds and How To's
Workflows
How to build an event registration Landbot (to be used in one screen by many attendees) (web only)
How to let user select a time of booking (with a minimum 45 minutes notice)
Send Files Hosted in Landbot to Your Google Drive with Make
Two-Step Email Verification
Skip the Welcome Message
Fixing Web Bot Loading Issues for iOS Devices in Meta Campaigns with Disclaimers
Progress Bar Workaround
How to set up questions with a countdown
Creating a Simple Cart in WhatsApp
More Topics
Table of Contents
- All Categories
- Other Channels - Messenger and APIChat
- API Chat (for Developers)
- Building a Slack APP Bot with Landbot APIChat and Node JS
Building a Slack APP Bot with Landbot APIChat and Node JS
Updated
by Pau Sanchez
Building a bot with our APIChat bot channel, allows you to build bots for other platforms like Slack, using our simple visual builder interface. You just need to connect both platforms webhooks and translate the messages flowing from one side to the other, and we will show you how to do it here:
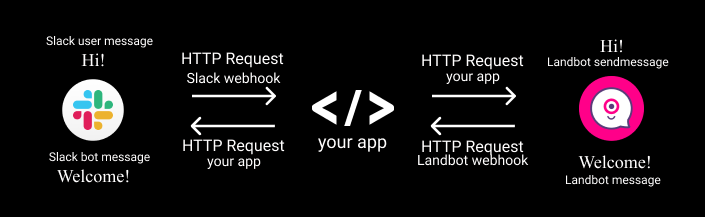
In Landbot
Creating an APIChat bot in Landbot builder
Once in the main dashboard, press BUILD A CHATBOT
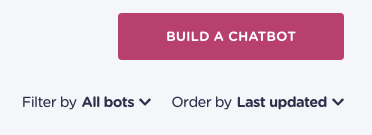
And select APIChatbot
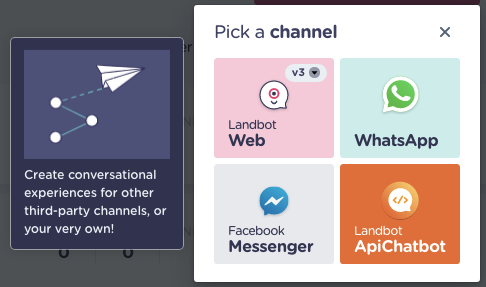
And the visual builder will create your first bot:
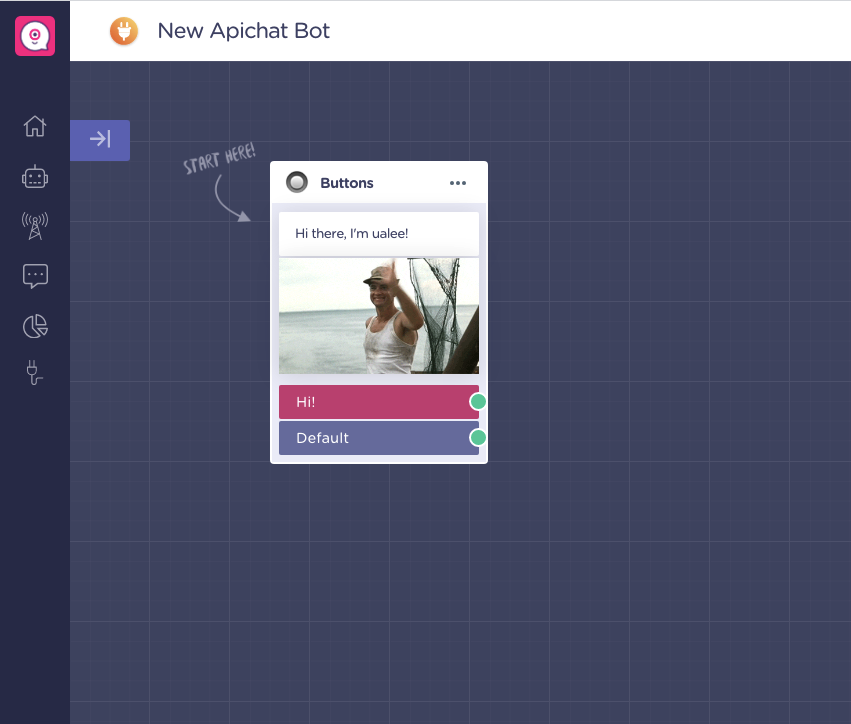
Using Ngrock To connect Landbot Hooks
We will use Ngrock to funnel the request from Landbot hooks, to do so from your terminal use this command:
ngrok http 80
We will have a url generated by ngrock were we can funnel temporarily our requests:
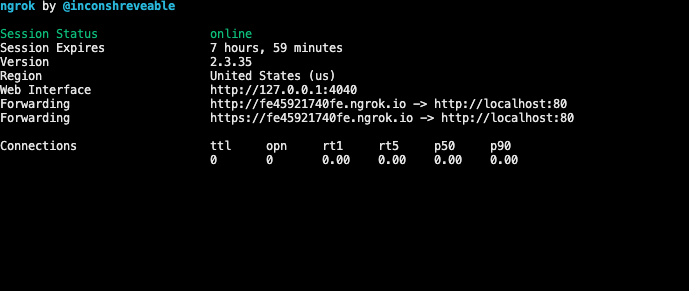
This is the url we will use:
https://fe45921740fe.ngrok.io
As we will funnel webhooks from Landbot and Slack we will add customise the route:
https://fe45921740fe.ngrok.io/landbot_hook
Creating a Channel to receive Landbot Hooks
Now let's go back to Landbot, to the Channels section, where we will link the new created bot:
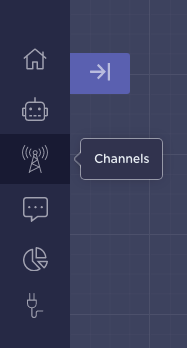
Select API
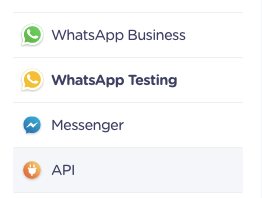
Select CREATE A NEW CHANNEL
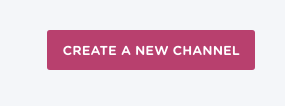
In this screen we will add first the recently created url by ngrok, we can change it later if needed:
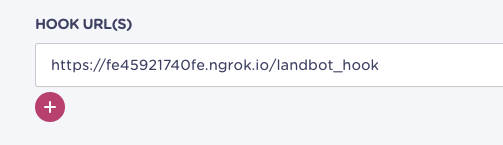
And press CONFIRM
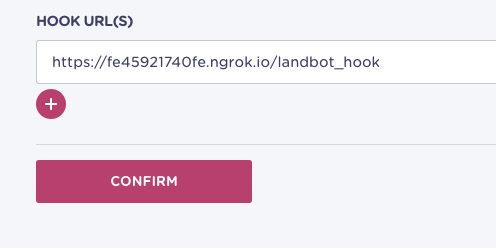
Once is confirmed, we will have our Channel created, as you can see an Auth Token will be generated:
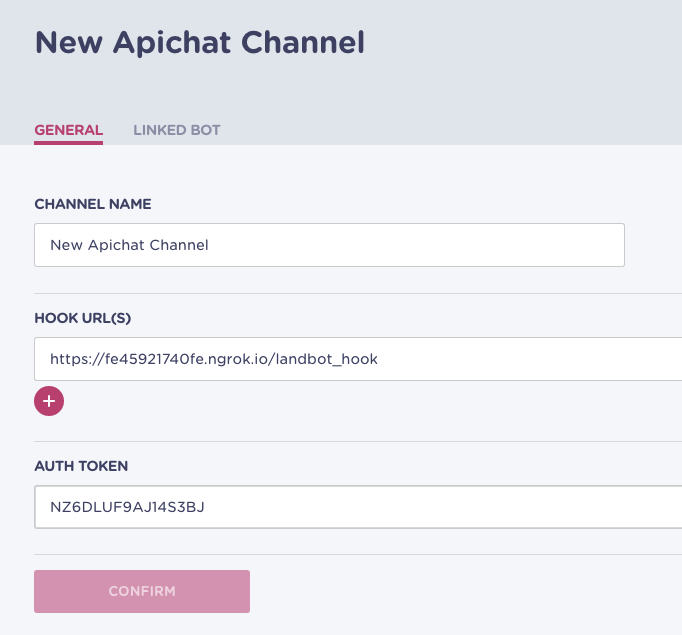
Now is time to link this channel to the bot we created early on, to do that press LINKED BOT:
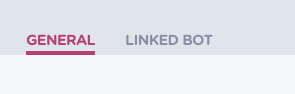
Now, press the dropdown to search for the bot:
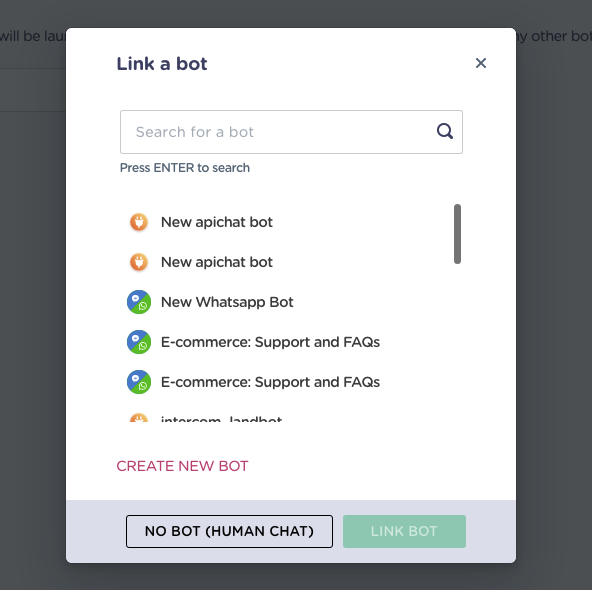
Select the bot and press Link Bot and press CONFIRM, and your bot will be linked to this channel. You can edit after again the details if needed.
Using Postman to trigger APIChat bot by sending a message (like a user)
Bots needs a message from the user to be initiated, to send a message we will use the following code:
curl --location --request POST 'https://chat.landbot.io/v1/send/' \
--header 'Authorization: Token <AUTH TOKEN GENERATED WITH THE CHANNEL>' \
--header 'Content-Type: application/json' \
--data-raw '{
"message": {
"type": "text",
"message": "Hello"
}
}'
We will use the import raw text option in Postman
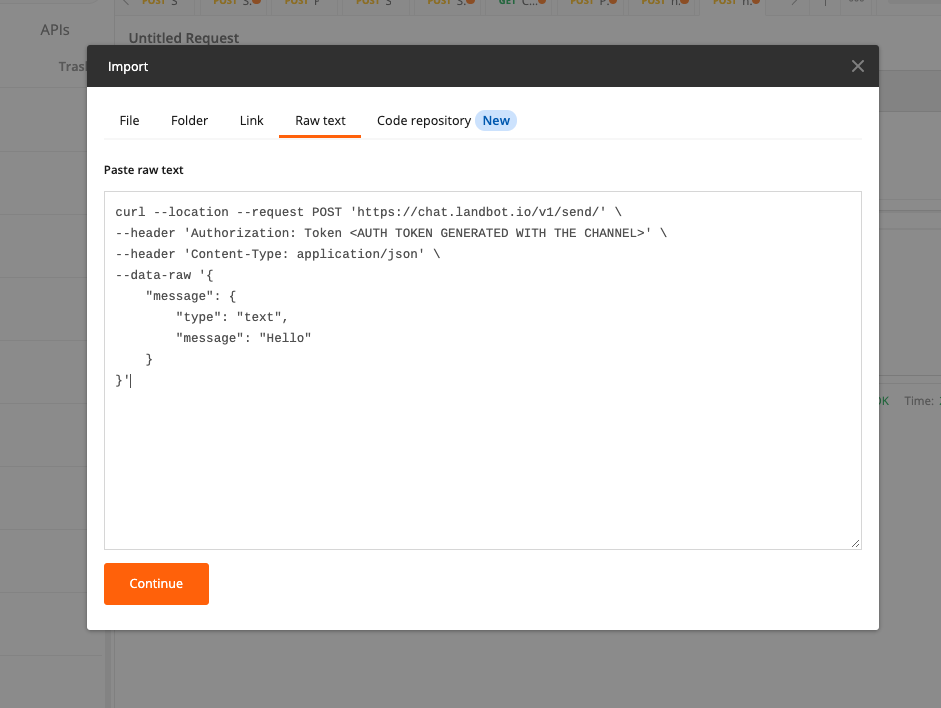
Remember to change edit to add the token you just generated in the creation of the Channel.
And the token added, make the Post request and we will have our response, bear in mind that if no "id" is given to the user, Landbot will create a new one for you:
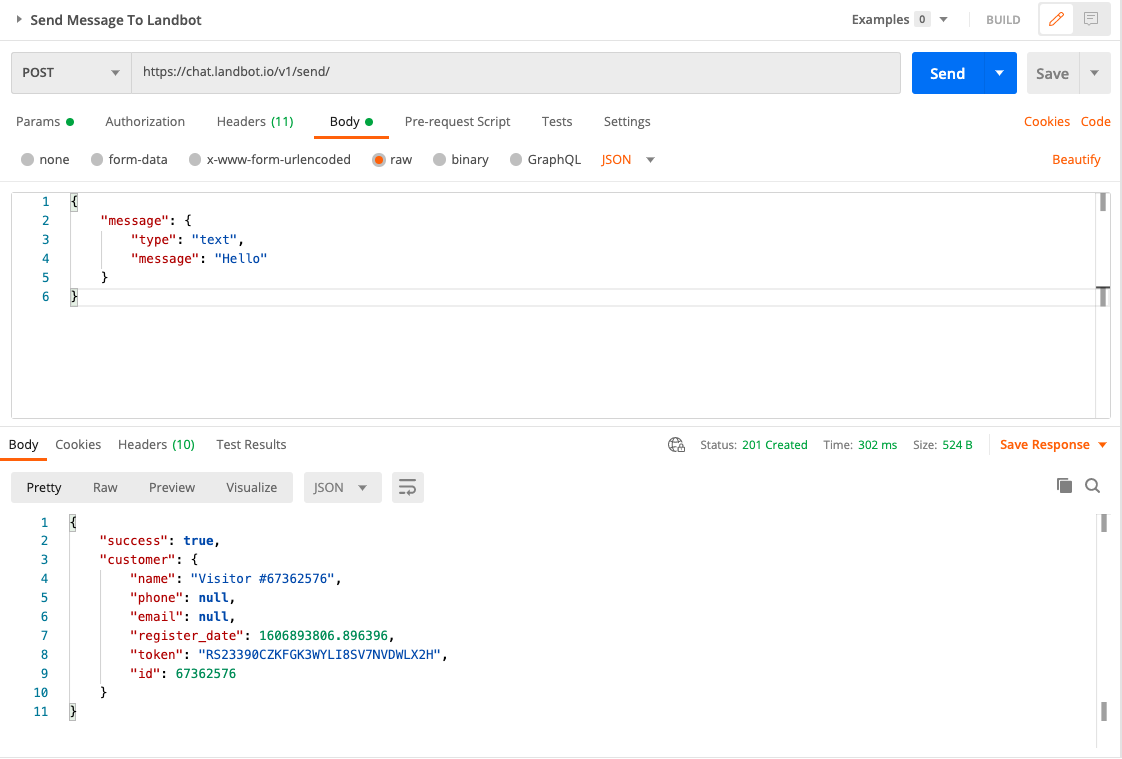
Inspect Hooks
We will use now the ngrok inspector to check from: http://localhost:4040/inspect/http
And as you can see below we will receive the first webhook from Landbot:
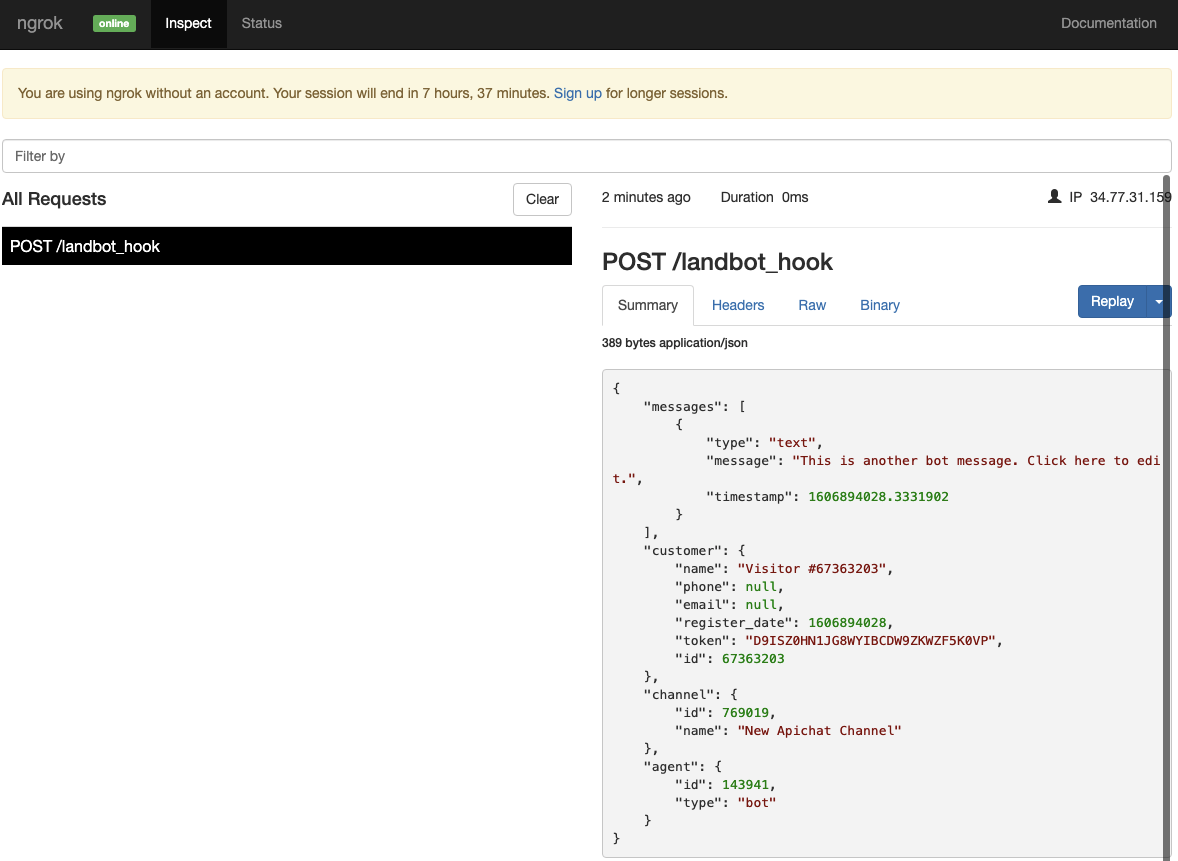
Great now we have Landbot APIChat receiving messages via API, and sending with to the hook the message that is designed in the logic
In Slack and your Node app
Create APP
Go to https://api.slack.com/apps
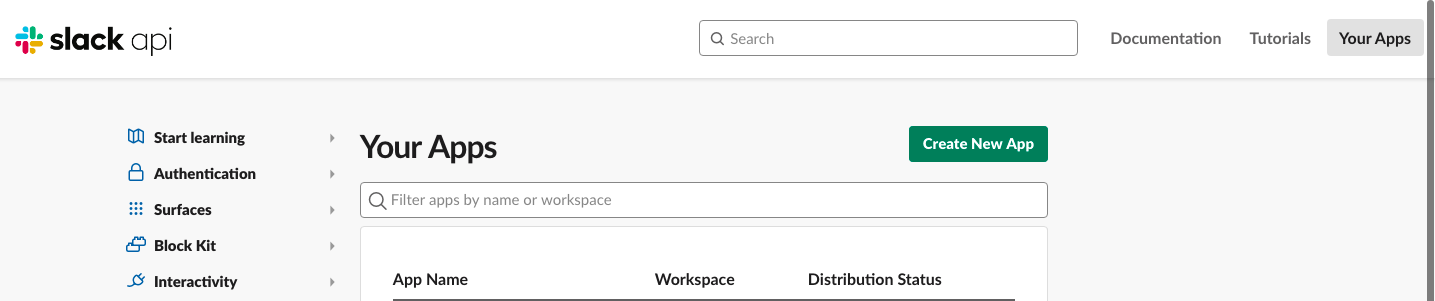
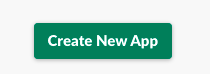
Press Create New App and this screen will appear:
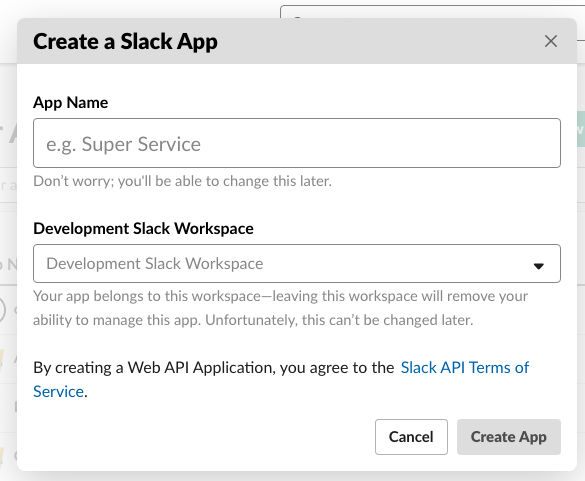
Fill the details and select a Slack Workspace, and press Create App:
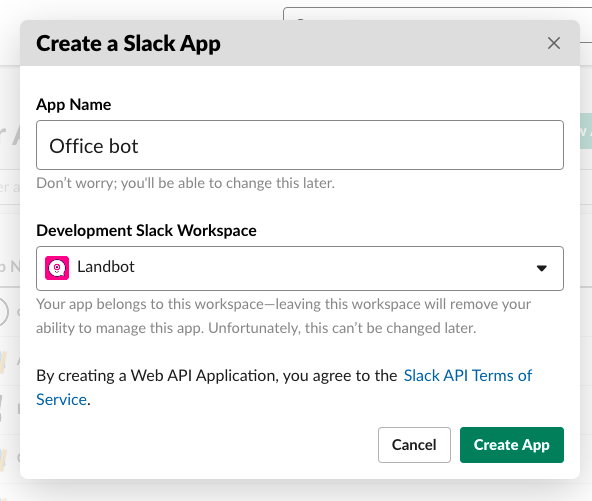
Once your App has been create select Basic Information:
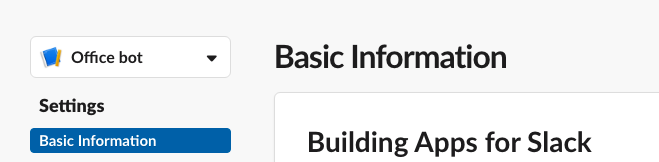
Scroll down to fill the details to customise the look of your App:
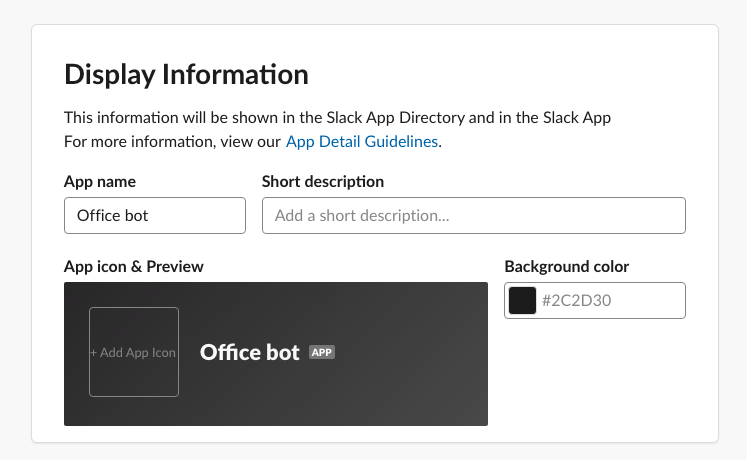
Set the scopes
Select from the side panel OAuth & Permissions:
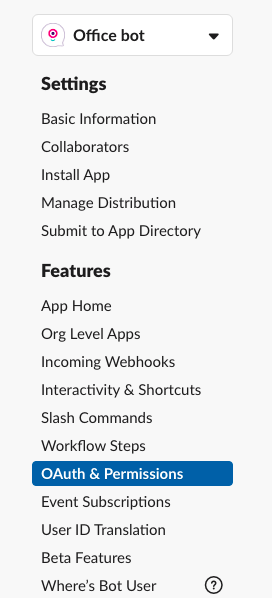
Scroll down to Scopes:
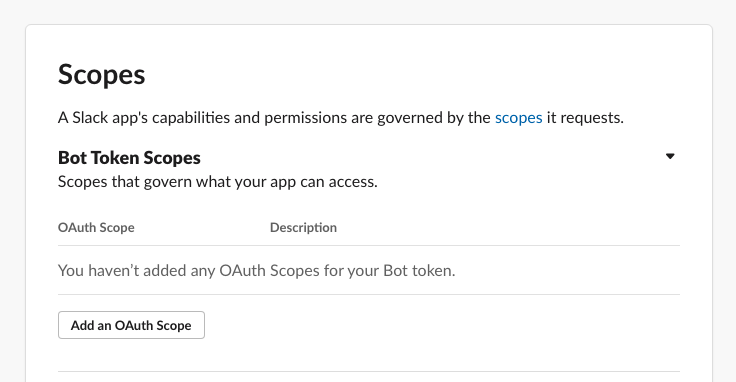
Under "Bot Token Scopes" press "Add an OAuth Scope":
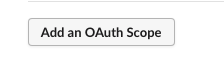
Add chat:write:
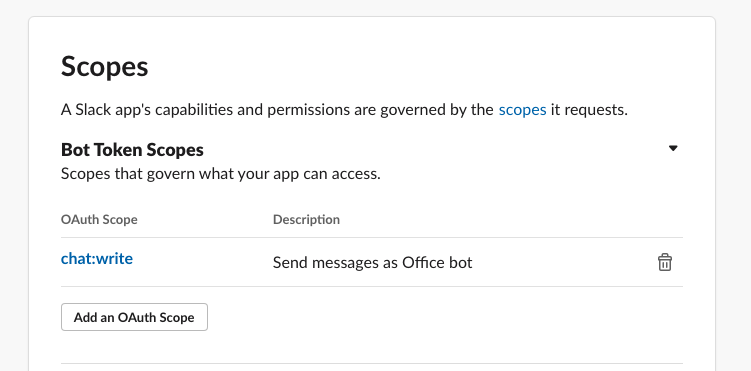
Scroll up again and select "Install to Workspace":
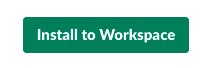
Grant permission to access your workspace:
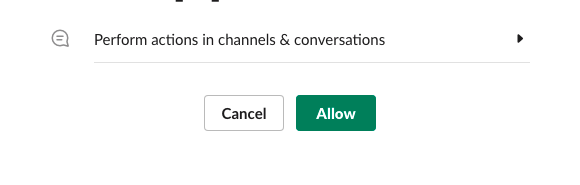
Once installed and allowed, you will have an Auth Token:
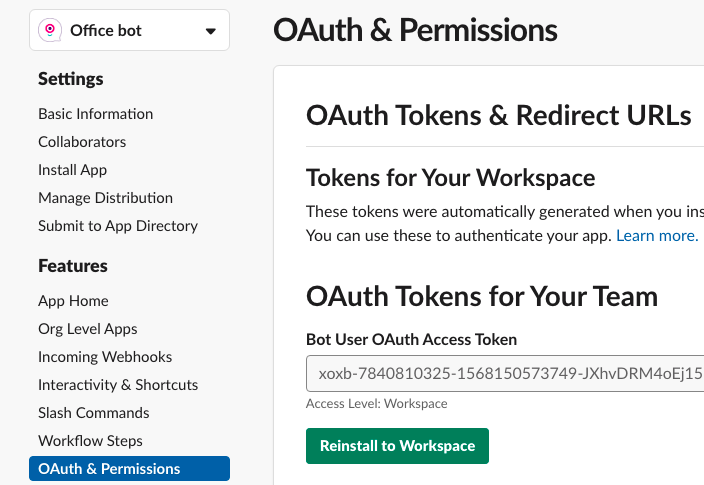
Now, go back to your Slack app. Search and select the APP we just created:
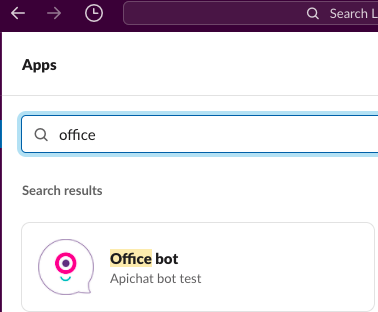
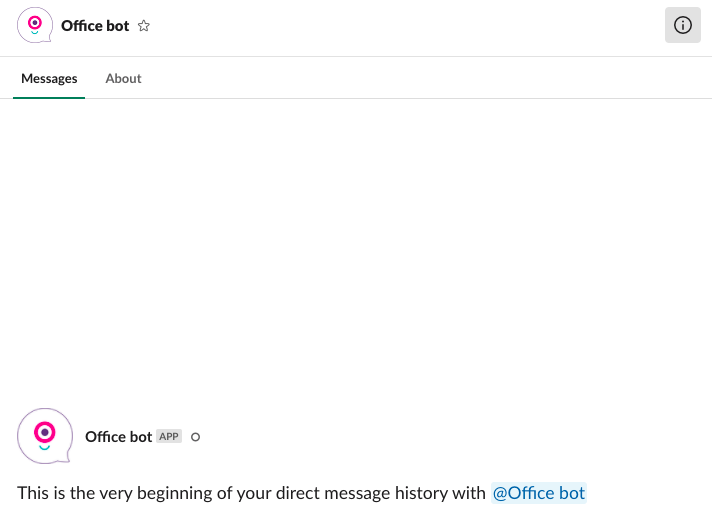
Send Message as the APP
To send a Message as our APP, first we will try with our integration:
First we need to find out our Channel id. To do that we will, press Right click on our app:
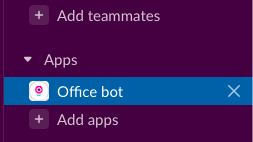
And press Right Click:
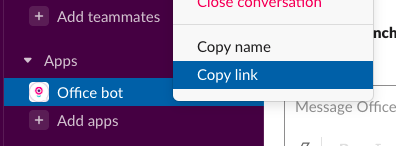
Paste the url in a browser, the last part is the Channel ID:

Test send message via PostMan with the channel id, and the OAuth we created early on:
curl --location --request POST 'https://slack.com/api/chat.postMessage' \--header 'Authorization: Bearer xoxb-7840810325-1570401658691-wmBIRKA7I8hPMTqK9d5Yd8wf' \--header 'Content-Type: application/json' \--data-raw '{ "channel": "D01H4QLTWC9", "text": "Hello, world"}'
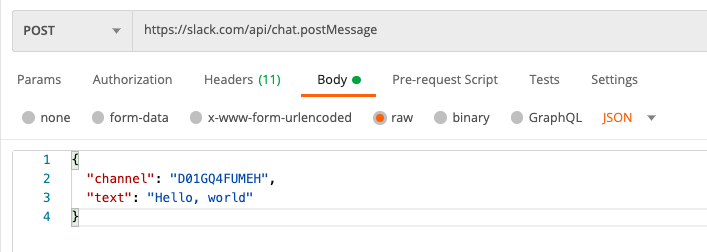
And you will see the message:
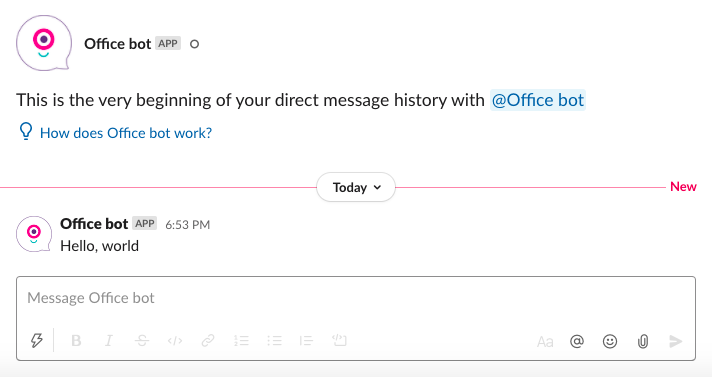
Set up basic Node APP
In the next steps we need to set up our Slack hook route to subscribe to the events happening to our Slack App, however, Slack needs to validate the endpoint with a "challenge". For that reason we will create the basic set up to be able to pass this challenge.
Let's create a new folder for our node app from the terminal
npm init -y
We will install the required packages for this app:
npm i express body-parser axios
And create index.js
touch index.js
In this index.js file we will add the initial code to be able to capture and respond to Slack webhook challenge:
Then initiate your app
node index.js
Once your app is up and running and listening, thanks to ngrock, we will continue to set up Slack webhooks in the Event Subscriptions
Subscribe to Events and Interactions
Go to the Event Subscriptions section in the side panel of the Slack app dashboard
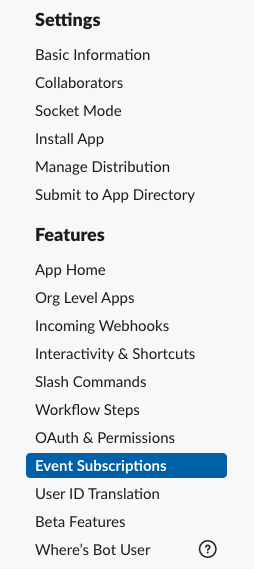
Turn on to Enable Events

And now we will use the ngrock url with our "slack_hook" route:
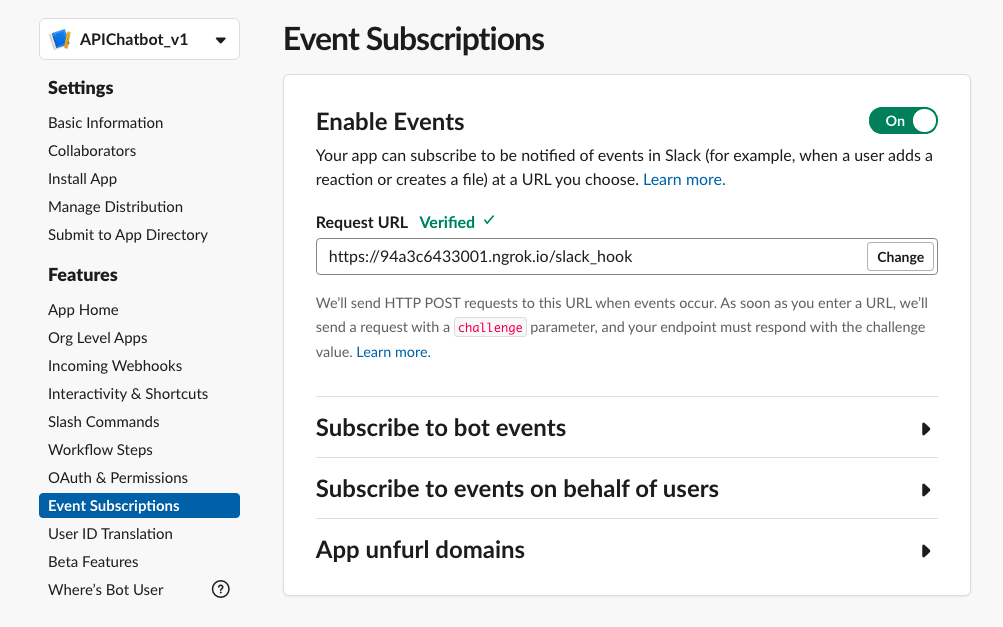
And make sure your endpoint is verified according to the challenge:

Once the Request URL has been verified, select "Subscribe to bot events":

Press "Add Bot User Event":
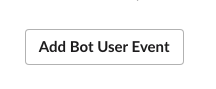
Search for "message.im" and select it:
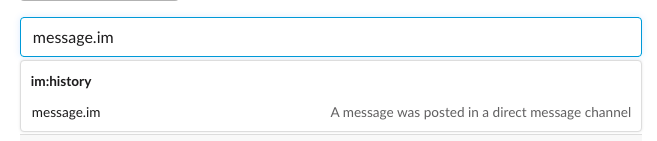
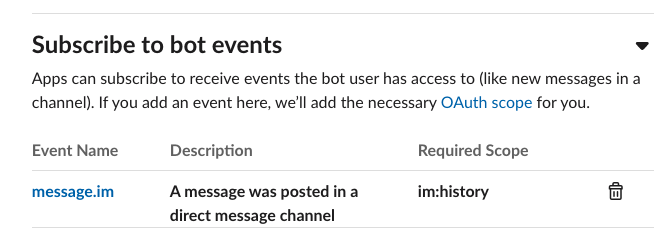
And press "Save Changes"
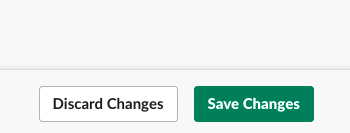
Then Slack will prompt you to "reinstall your app":

Press the link to "reinstall your app":
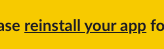
And select Allow:
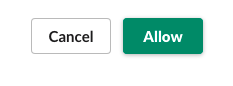
Now Slack is ready to send you events once the user types and send in the app created. Let's adapt our code in index.js file to receive the first event (that is not a challenge):
Now, once the changes had been done in the index.js file and your app is again listening, return to Slack, and send your first message to the app:
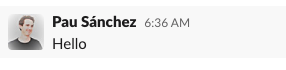
If you return to the terminal you will see printed the event that Slack has sent to us:
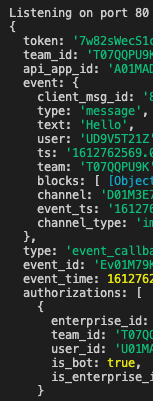
And also, you will be able to track the request in ngrok
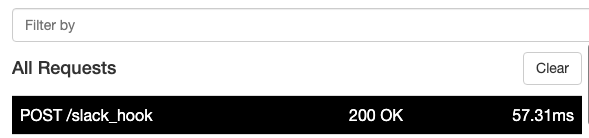
Finally, we will add also to the app the option to send us events if the user presses a button in Slack. To do so, go to "Interactivity & Shortcuts":
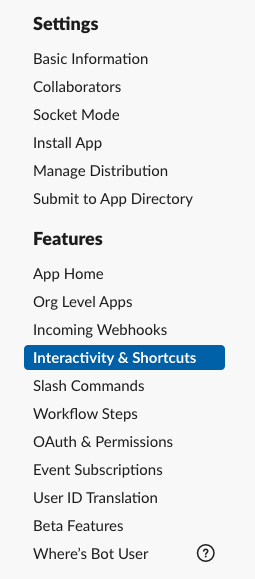
And switch On:
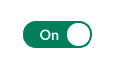
Add the same Request URL we used before, this time won't send a challenge :
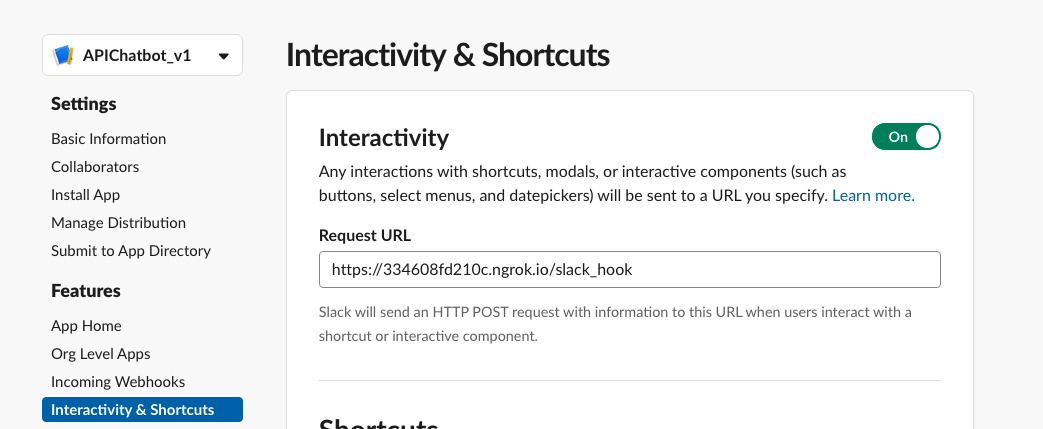
And press Save Changes

And that's it in the Slack APP settings. Bear in mind that you might need to change the url if ngrock expires.
Main file NODE APP
For this example all the code needed to communicate between both platforms, will be in a file called index.js, that we will add to our recently created folder.
Below is the code that you can copy and paste for your project
Please feel free to adapt this code to your production needs.
Please feel free to customise the file to your needs
Auth and tokens
The index.js file already has the variables created, you just need to replace accordingly:
// LANDBOT APIChatbot details
const landbot_token = "xxxxxxxxxxxxxxx" // Landbot API Channel TOKEN
const landbot_url = "https://chat.landbot.io/v1/send" // Landbot API base url//
OTHER PLATFORM API details, in this case Slack
const slack_token = "xxxx-12312312312" //Oauth
const slack_url= "https://slack.com/api/" // Slack API Send message base url
Builder Edit
Before we test the bot integration, will be better if we edit the bot to display simple two messages:
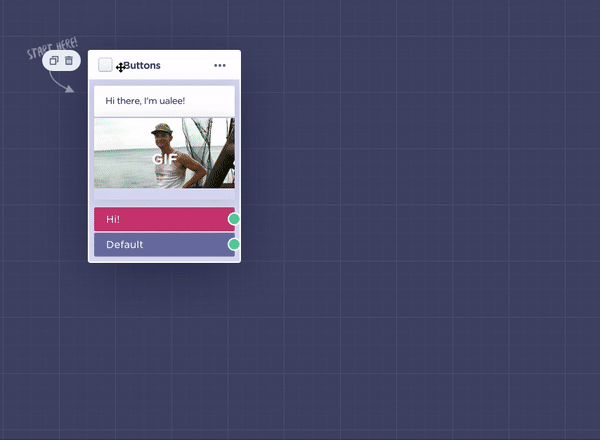
Also, before the test make sure the url endpoint settings are correct in both platforms (Slack and Landbot)
Test
Once is initiated, send a message from Slack. That will trigger the app, and send a message to Landbot, and Landbot will reply with a message that will be sent back to Slack
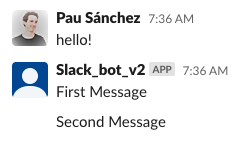
And now your Slack bot is ready to go! Please check the tips below they will help you to improve the bot
Important Tips!
Order of the messages (received and sent)
Even if messages by default are sent to the hook in order, as you have seen in the code, there might be times where it comes in a different order. The best approach will be to check the timestamp of the message as a reference.
At the same time, be aware that Slack API doesn't offer the possibility to set up a prior order has how the messages have to be displayed.
For that reason, short sequences offer much better results
First block ${body}
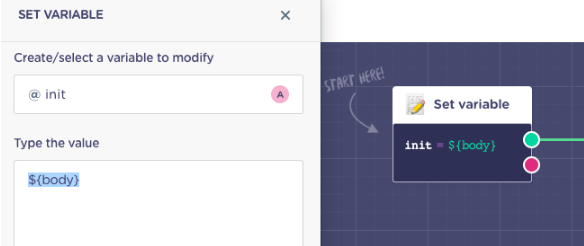
The best approach when building bots for channels like Slack, where the user has free input to start interacting with the bot, is to capture the first input. Thanks to do that we will be able to set up conditions.
To capture the firs input, please check this article
Individual messages and sequences length
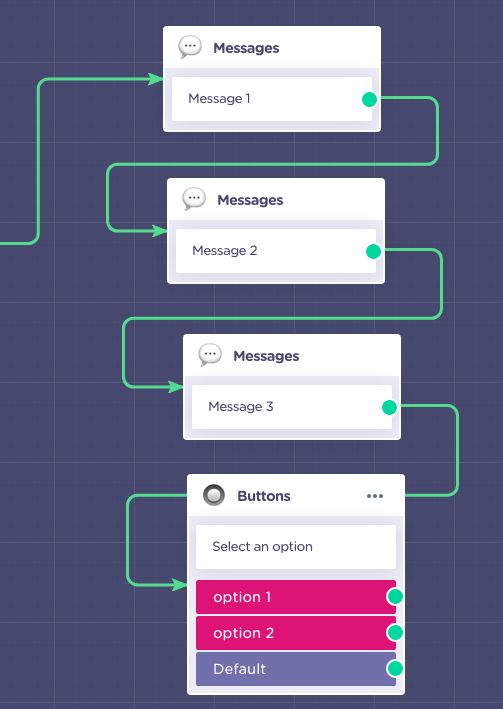
When building the bot, you can create blocks that contains more than one message or media, please avoid it. It will be much simpler to control the message delivery, order and process
Sequence of messages, are going to start after a user input/selection block is required and stop in the next user input/selection block. For better control of the order of the messages, avoid sequences of more than 4 blocks. The ideal built is like in the picture above.
Global Keywords block
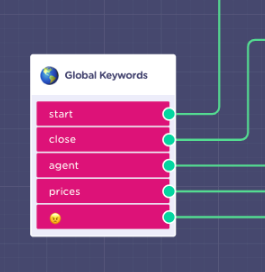
The global keywords block allow you to set up paths, based on a keyword input from the user (not bot or agent). It is very handy to redirect the user to specific flows. For more information, please check this article
When used, global keywords takes priority over other inputs used. Be careful to do not use the same keywords that might be used in other blocks like Buttons
Keyword Options

Very similar to the buttons block, this block has been designed for open text channels like Slack. It offers the chance to select a number or a text. For more information, please check this article
Chat Section
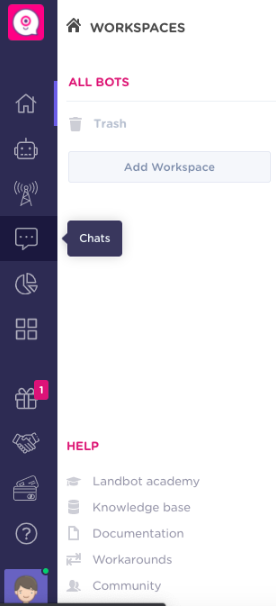
When testing the bot, it is very handy to see what is going on, like the messages and variables that had been used. Also, if you want to close the chat session to start from the beginning it will be simple to do it from this section. Please check more about the chat section here
Ngrock
If you choose to work with Ngrock in the free mode you must be aware of a couple of things that will help you to spot some issues:
1. The url will change after few hours. It is normal that after few ours of testing to forget about it.
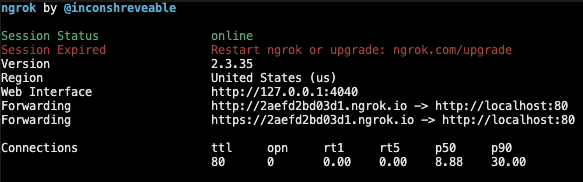
Once the session is again working, remember to change the url in Landbot and Slack
2. Ngrock has a limitation of requests per second. That is important as messages coming from the bot (landbot) will send many messages at once, and Ngrock might stop working as expected. When testing be aware of it