Build a bot
Getting started
Basics and builder
Managing Data in Your Chatbot: A Guide to Using Fields
Builder's keyboard shortcuts and mechanics! 🏗
Builder Interface
How to "debug" (troubleshoot) your bot's flow to spot possible errors (for non coders)
Starting Point
How to disable a bot
Bricks
Bot's Settings
Bot General Settings
Second Visit Settings
Custom System Messages
Hidden Fields (Get params / UTMs from url and use it as variables)
Landbot native SEO & Tracking tools
Typing Emulation (Message Delay)
Build it for me!
Getting started - build a bot
Messages, Questions and Logic & Technical blocks
Messages
Media Block
Media block
How to display images with a variable URL source
How to embed a .gif file inside a message
Different ways to embed Videos in Landbot
Display video and hide button to continue until video has ended
Send a Message block - Simple Message
Goodbye block
Question blocks
Date Block
Email Block
Form Block
Scale Block
Buttons block
How to set up Multiple Choice questions
Multi-Question block
Question: Address block
Question: Autocomplete block
Question: File block
Ask for a name
Question: Number block
Question: Phone block
Question: Picture Choice block
Question: Rating block
Ask a Question Block
Question: URL block
Question: Yes/No block
Logic & Technical blocks
Code Blocks
Dynamic Data
Dynamic Data (Arrays)
Get the array's index of the user selection and extract information from array
Formulas
How to Perform Basic Calculations
Get started with the Formulas block
Formulas Blocks Dashboard
Formulas - Regex
Formulas - Date
Formulas - String
Formulas - Logical
Formulas - Math
Formulas - Object
Formulas - Comparison
Formulas - Array
Persistent Menu
Trigger Automation
Webhook
Webhook Block Dashboard
Webhook block (External API REST)
How to TEST your Http Request (Webhook block)
Landbot System Fields
Set a Field block
Any of the above Output
Global Keywords 🌍
Keyword Jump
Lead Scoring block
Jump To block
AB Test
Conditions block
Conditions block II (with Dates, Usage and Agents variables)
Close Chat block
How to ask a question based on a variable not being set (empty URL params)
Business Hours block
Custom Goals
Note block
Share & Embed
Redirect Users
How to open a new URL in another tab (window)
How to redirect visitors to a URL (web only)
Conditional Welcome (Non-embedded bots)
How to add a Click-to-Call/Email/WhatsApp button
Redirect User Based on Language Input (DeepL)
Generate a URL that has variables from user answers
Popup on Exit Intent
Share
Customized Embed Actions
How to redirect user to another url in your site with Livechat open to continue conversation
How to Detect Visitors Browser
Customize and embed your WhatsApp Widget
Modifying Embed Size
Conditional Welcome: Direct the Flow of Your Bot Based on External Parameters
Detect if bot was opened
Customized Behavior in Mobile Browsers
Load script and display bot on click button
Launch Bot On Exit Intent
Display Bot During Business Hours Only (Livechat & Popup)
Open / Close a Web bot (embedded)
Launching a bot depending on browser language
How to pass WordPress logged in user data to Landbot
Set the flow depending on the url path (for embedded landbots)
How to launch a Landbot by clicking a button
Open LiveChat bot as soon as page loads
Detect if a visitor is on Mobile/Tablet or Desktop
Embed
Embed the bot into your Website
Embed Landbot in an iframe
Landbot in Wix
Landbot in your web with Google Tag Manager
Landbot in Webflow
Embed in Sharetribe
Landbot in Shopify
Embedding Landbot in Carrd
Landbot in Wordpress
Landbot in Squarespace
Customizing the Proactive Message
Design section (web bots)
Verification & Security
Account Settings and Billing
Billing
Account Settings
Account Settings
Account Homepage
Dashboard
Common reasons for not receiving account activation email
Privacy and Security
Landbot's Privacy & Security Policies
Compliance with the GDPR - General Data Protection Regulation
Landbot's Abuse Policy - How to Report an Abusive Use of Landbot
Delete Customer Data in Flow
Teammates
Agent Status and Log out
Roles & Permissions for Teammates
Manage Landbot Teammates - Add and Customize Agents
Notifications section
Startup Discounts
NGOs and Educational Organizations Discount
Human Takeover & Inbox
Integrations with Landbot
Native Integrations
Airtable
Airtable integration block
Get data filtered from Airtable with a Brick- Shop example
20 different ways to GET and filter data from Airtable
How to add/update different field types in Airtable (POST, PATCH & PUT)
How to Create, Update, Retrieve and Delete records in Airtable (POST, PATCH, GET & DELETE)
Get more than 100 items from Airtable
Insert Multiple Records to Airtable with a Loop
How to Get an Airtable Token
Advanced filters formulas Airtable block
Airtable usecase: Create an event registration bot with limited availability
Update Multiple Records in Airtable Using a Loop
Reservation bot with Airtable
Calendly
Dialogflow
Dialogflow & Landbot course
Dialogflow & Landbot intro: What is NLP, Dialogflow and what can you do with it?
Dialogflow & Landbot lesson 1: Create your first agent and intent in Dialogflow
Dialogflow & Landbot lesson 2: Get the JSON Key
Dialogflow & Landbot lesson 3: Setting up of Dialogflow in Landbot
Dialogflow & Landbot lesson 4: Training phrases and responses for a FAQ
Dialogflow & Landbot lesson 5: Entities and Landbot variables
Dialogflow & Landbot lesson 6: Redirect user depending on Dialogflow response parameters (intent, entities and more)
Learn more about Dialogflow - Courses and communities
Integrations > Dialogflow Block
How to extract parameters from Dialogflow response with Formulas
Dialogflow Integration Dashboard
Dialogflow in Unsupported Languages (& Multilingual)
Dialogflow - How to get JSON Key
Google Sheets
Google Sheets (Insert, Update and Get data)
How to use Google Sheets to create a simple verification system for returning visitors
How to Upload Document & store link
How to give unique Coupon Codes (with Google Spreadsheets)
Google Sheets Integration Dashboard
How to insert a new row with data and formulas in Google Sheets
How to use Google Spreadsheet as a Content Management System for your bot
Hubspot
MailChimp
Salesforce
Segment
SendGrid
Slack
Stripe
Zapier
How to Configure the Landbot and Zapier Integration Using the Zapier Block
Zapier Integration Dashboard
How to insert a row to Google Spreadsheet by Zapier
How to generate a document with PDFMonkey by Zapier
Send WhatsApp Message Templates from Zapier
How to send an email with Gmail by Zapier
Get Opt-ins (Contacts) from Facebook Leads using Zapier
How to extract data from an external source with Zapier and use it in Landbot
Zapier trigger
How to complete a digital signature flow by Zapier
Make a survey with Landbot and display the results in a Notion table using Zapier
Zendesk
Non-Native Integrations
ActiveCampaign
Google Calendar
Google Fonts
Google Maps
Embed Google Maps
Google Maps API Key for Address block
Extract Data With Google Maps Geocoding API
Calculate Distances With Google Maps API
Google Meet
IFTTT
Integrately
Intercom
Make
Connecting MySQL with Make.com (formerly Integromat)
How to upload a file to Google Drive using Make.com (formerly Integromat)
Send WhatsApp Message Template from Make
Make Integration With Trigger Automation Block
How to send an email through Sendinblue by Make.com (formerly Integromat)
Get Opt-ins (Contacts) from Facebook Leads using Make
How to extract data from an external source with Make.com and use it in Landbot
OCR
Pabbly
Paragon
Pipedream
PDF Monkey
Store Locator Widgets
Xano
Zendesk
WhatsApp Channel
Getting started!
WhatsApp Testing
Build a WhatsApp Bot - Best Practices and User guide
Build a WhatsApp Bot - Best Practices for Developers
Types of Content and Media you can use in WhatsApp 🖼
1. WhatsApp Article Directory
2. WhatsApp Integration FAQ's
Adding & Managing your WhatsApp Channel
Facebook Business Verification - Best Practices 🇬🇧
WhatsApp Number Deletion (WA Channel management)
Adding a WhatsApp number to your account
WhatsApp’s Messaging Policy: New Accepted Industry verticals
Meta processes guide: FBM verification, Official Business Account (OBA) requests, Appeals
Additional Number integration: Limitations and Requirements (Number integration)
Existing WhatsApp Number Migration
Key Insights for Migrating to WhatsApp Business API Cloud
How to's, Compatibility & Workarounds
WhatsApp bots - Feature Compatibility Guide
WhatsApp - How to direct a user through a different bot flow on their second visit
WhatsApp - Get user out of error message loop
Getting Subscribers: Opt-in, Contacts
How to get Opt-ins (Contacts) for your WhatsApp 🚀
WhatsApp Quality - Best Practices
Opt-In block for WhatsApp 🚀
Opt-in Check Block
WhatsApp Channel Settings
Parent Bot/Linked Bot - Add a main bot to your WhatsApp number
WhatsApp Channel Panel (Settings)
Growth Tools for WhatsApp
Duplicating bots
Messaging and contacting your users
WhatsApp Campaigns 💌
WhatsApp's Message Templates
Audience block
WhatsApp Marketing Playbook: Best Practices for Leadgen
Audiences
WhatsApp for Devs
How to calculate the number of days between two selected dates (WhatsApp)
Creating a Loop in WhatsApp
Recognise the users input when sending a Message Template with buttons
Trigger Event if User Abandons Chat
Calculate Distances in WhatsApp
Send Automated Message Templates based on Dates
Trigger Event if User Abandons Chat Using AWS
reply from Slack: How to create an integration to allow agents reply WhatsApp users from Slack (with Node JS)
Set Up a Delay Timer in Bot
Notify Teammates of Chat via WhatsApp
Native blocks for WhatsApp
Other Channels - Messenger and APIChat
Facebook Messenger
The Facebook Messenger Ultimate Guide
Types of content you can use in Messenger bots 🖼
How to Preview a Messenger bot
Facebook Ad connected to Messenger Bot
API Chat (for Developers)
AI in Landbot
Non-native AI Features
Create a JSON format response from OpenAI in WhatsApp
Connect OpenAI Assistant with Landbot
AI in WhatsApp
How to build a FAQ chatbot with GPT-3
GPT-4 in Landbot
OpenAI
Prompt Engineering for GPT-3
Build a Customer Service Bot with ChatGPT and Extract Information
Google Gemini in Landbot
Native AI Features
Metrics and Data Management
Metrics Section
How to export the data from your bots
Export data: How to open a CSV file
Bot's Analyze Section
For Developers & Designers
JavaScript and CSS
CSS and Design Customizations
Design Customizations
Advanced (Custom CSS & Custom JS)
Components CSS Library Index
Background Class CSS
Identify Blocks CSS
Buttons Class CSS
Header Class CSS
Media Class CSS
Message Bubble Class CSS
Miscellaneous Classes CSS
CSS Customization Examples: "Back to School" Theme
Get started guide for CSS Design in Landbot
CSS Customization Examples: Call To Action: WhatsApp
CSS Examples: Lead Gen
CSS Customization Examples: "Translucid"
CSS Customization Examples: "Minimalist" Theme
Dynamic Data CSS
Form Block CSS
CSS for Typewriter Effect
CSS Customization Examples: Carrd Embed Beginner
Dynamically Change a Bot's Background
Proactive Message Customizations with Javascript and CSS
Landbot v3 - Web CSS - RTL
Multi Questions CSS
CSS Customization Examples: Video Bubble
Dynamically Change Any Style
CSS Customization Examples: CV Template
Change Landbot custom CSS dynamically from parent page onload
Widget/Bubble Customizations with Javascript and CSS
JavaScript
How to change Avatar dynamically (Landbot v3)
Javascript in WhatsApp
Javascript in Landbot v3
Different ways to format numbers with JS
How to display an HTML Table and a List in Landbot v3 web
Trigger a Global Keyword with JS (web v3)
Create Dynamic Shopping Cart with JS and CSS
Add a Chart (with Chart JS library) in your Landbot
Different ways to format numbers with JS (WhatsApp)
Pop up modal to embed third party elements
Landbot API
Send WhatsApp Messages with Landbot API
How to "send" a user to a specific point in the flow with Javascript and with the API
APIs
Get Opt-ins (Contacts) using Landbot API
MessageHooks - Landbot Webhooks
Resume flow based on external process with Landbot API (Request, Set, Go)
Tracking
Google Analytics - Track Events (Not embedded)
Google Analytics - Track Events (Embedded)
Meta Pixel - Track Events (only Embedded)
How Track Google Analytics Events in Landbot with Google Tag Manager (GTM)
Google Adwords - How to track Google Adwords in Landbot
Set a timer to get the time spent during the flow
Workarounds and How To's
Workflows
How to build an event registration Landbot (to be used in one screen by many attendees) (web only)
How to let user select a time of booking (with a minimum 45 minutes notice)
Send Files Hosted in Landbot to Your Google Drive with Make
Two-Step Email Verification
Fixing Web Bot Loading Issues for iOS Devices in Meta Campaigns with Disclaimers
Progress Bar Workaround
How to set up questions with a countdown
Creating a Simple Cart in WhatsApp
More Topics
Table of Contents
- To trigger code inside the bot in specific steps of the flow: Code (Javascript) block
- To Set the Value of a Javascript variable from of a Landbot Variable
- To Set the Value of a Landbot variable with Javascript
- To communicate between a Code block and Bot Body (Design > Custom Code section)
- To add a script snippet in the embedding page (3rd party JS library)
- To communicate between Landbot and the Embedding Page (window scope)
- To communicate between the Embedding Page to Landbot
- To trigger actions in Embedded bots from the container page
- Landbot 3 SDK
- All Categories
- For Developers & Designers
- JavaScript and CSS
- JavaScript
- Javascript in Landbot v3
Javascript in Landbot v3
Updated
by Pau Sanchez
- To trigger code inside the bot in specific steps of the flow: Code (Javascript) block
- To Set the Value of a Javascript variable from of a Landbot Variable
- To Set the Value of a Landbot variable with Javascript
- To communicate between a Code block and Bot Body (Design > Custom Code section)
- To add a script snippet in the embedding page (3rd party JS library)
- To communicate between Landbot and the Embedding Page (window scope)
- To communicate between the Embedding Page to Landbot
- To trigger actions in Embedded bots from the container page
- Landbot 3 SDK
When testing Javascript be sure to use the 'Share URL' and not 'Preview'
Web Landbots offer the possibility to use Javascript in a large number of ways to make the most of Landbot and work together with your flow and your site. Here is a list of possible things you can do:
To trigger code inside the bot in specific steps of the flow: Code (Javascript) block
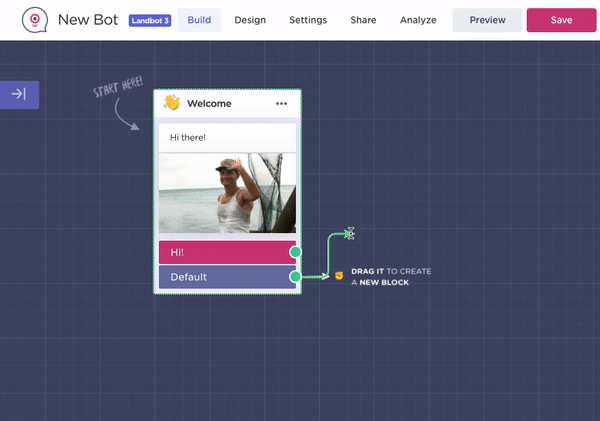
Sometimes we want to trigger some Javascript functions during the flow, here is where we can do it.
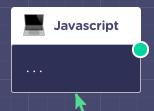
The code in these blocks will be active only when the visitor passes through this point. So functions in the block don't have a global scope.
This code will be embedded inside <script> </script> tags, so there is no need to add them, as you can see below. In this example, when the visitor passes through this block, a "hello world" will be printed in the console
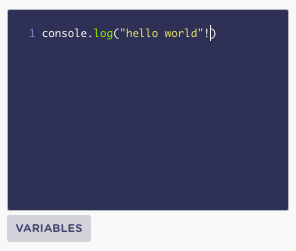
To Set the Value of a Javascript variable from of a Landbot Variable
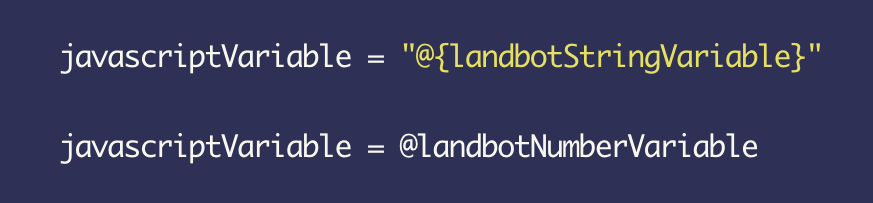
Let's say you want to print on the console the variable welcome
- First, we are going to set the value of a Javascript variable called 'welcomeinput' to the value of the Landbot variable @welcome
- Now we add the code to show in the console
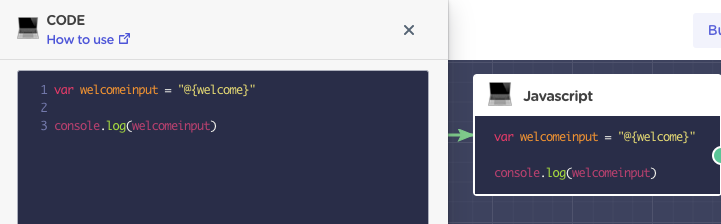
var welcomeinput = "@{welcome}";
console.log(welcomeinput);
Now we can print it:
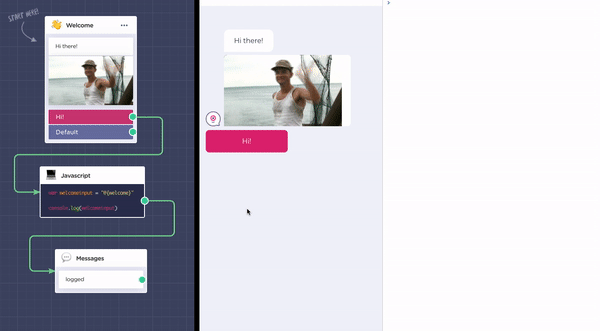
If you are working strings that contain line breaks or objects that might contain boolean values (true/false) use template literals to set Javascript variables. Example:
var object = `@{array}`;
console.log(object);
To Set the Value of a Landbot variable with Javascript
In the example below, we are going to give the Landbot variable called @var, the value of 3000
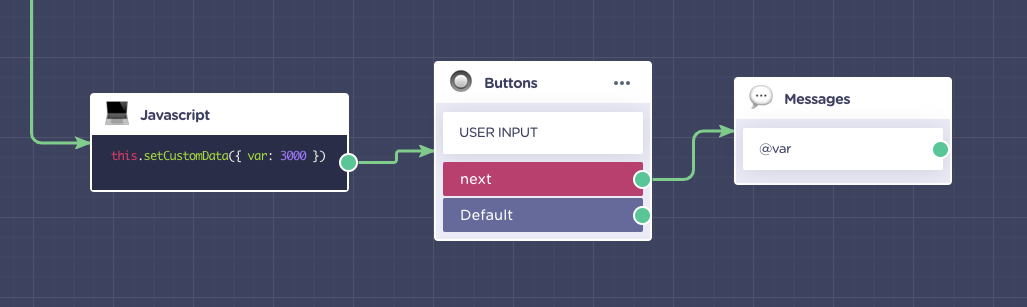
- We use the code block to set the value of a variable (in this example the Landbot variable is called "@var") :
this.setCustomData({ var: 3000 });
- We need to add an input for the user, to pass the complete the process before the value is available in Landbot scope (NOT OPTIONAL)
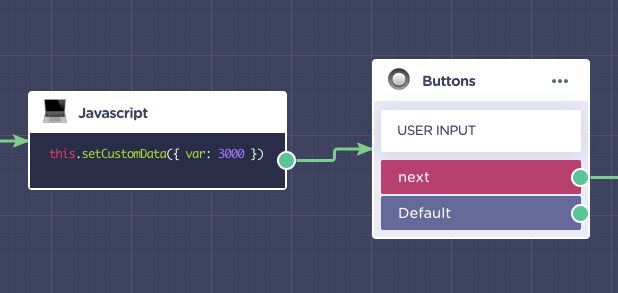
- And finally, we set up a block, where we will display the new Landbot value
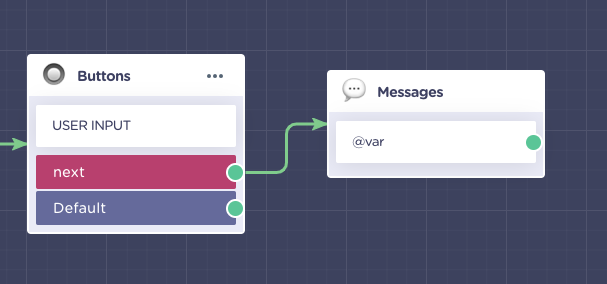
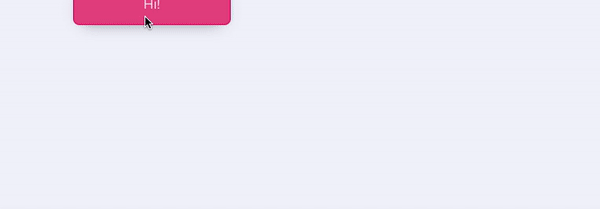
Generating the value of a Landbot variable could be as simple as in the example below where we make sure numbers have 2 decimals, and transform the numeric Landbot variable @value :
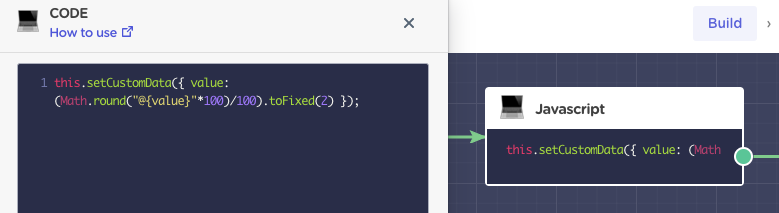
this.setCustomData({ value: (Math.round("@{value}"*100)/100).toFixed(2) });
Example: Set a variable right on the loading time of the bot
In this example, we set a value in a Landbot variable called @randomnumber. To do that we will use the section Add JS (Design > Custom Code)
<script>
var landbotScope = this;
this.onLoad(function(){
const randomNumber = Math.floor((Math.random() * 9999) + 1);
landbotScope.setCustomData({randomnumber:randomNumber})
})
</script>
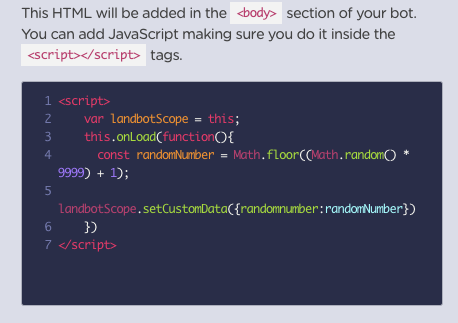
This variable won't display in any of the variables' lists, unless you create it manually
Example: Encode user input
To communicate between a Code block and Bot Body (Design > Custom Code section)
In the Design > Custom Code > Add JS section, you are able to add code, that:
- Is loaded as soon as the bot is loaded
- Can be triggered by Code blocks independently
- It is not compatible with Jump to
Example: Pass value to body scope from code block and log it
Here is an example of how you can communicate between the bot (code block) with a variable in the Design/Custom Code section:
- Code block:

this.window.setVariable("@{welcome}")
And in the Customs Code section:

<script>
var landbotScope = this;
this.onLoad(function() {
landbotScope.window.variableName = null;
landbotScope.window.setVariable = function(data) {
landbotScope.window.variableName = data;
console.log(landbotScope.window.variableName);
}
});
</script>
Other examples:
To add a script snippet in the embedding page (3rd party JS library)
Maybe we are interested to load a style or a script in our embedding page, as soon as the bot loads, and trigger it whenever we want with a code block.
Here is how you can add such script tag:
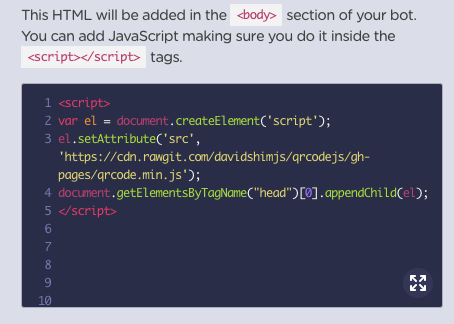
<script>
var el = document.createElement('script');
el.setAttribute('src', 'https://cdn.rawgit.com/davidshimjs/qrcodejs/gh-pages/qrcode.min.js');
document.getElementsByTagName("head")[0].appendChild(el);
</script>
We will find this after in the Window scope, of the embedding page.
Example: Generate QR code based on user input
- As displayed above we add the following code in the Custom Code section:
This will allow us to use this bot, embedded or not.<script>
var el = document.createElement('script');
el.setAttribute('src', 'https://cdn.rawgit.com/davidshimjs/qrcodejs/gh-pages/qrcode.min.js');
document.getElementsByTagName("head")[0].appendChild(el);
</script>
<script>
var landbotScope = this;
this.generateQr = function(data){
setTimeout(function(){
var elementtag = landbotScope.document.getElementById('qrcode')
this.printQR = new QRCode(elementtag, data)
},1000)}
</script> - Then in the builder we create a Question Text, where we can gather the URL that we are going to use to generate the QR code:
- After the Question Text we will add a Code block that will trigger the QR, with some delay enough so the following block is available
- After that, we just need to use a Set Variable block which will contain the QR code and a Buttons block to display the QR:
Here is how it will work:
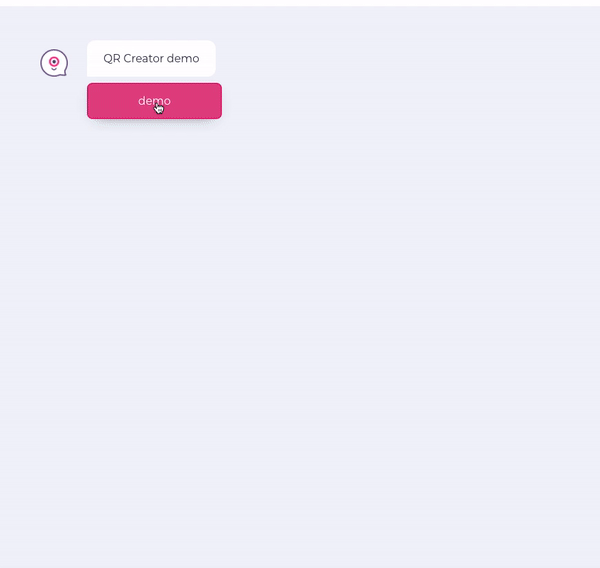
Open livechat on "exit intent" (add script to parent page)
In this example we trigger the bot to open when the user hovers outside the window, desktop only, and only once.
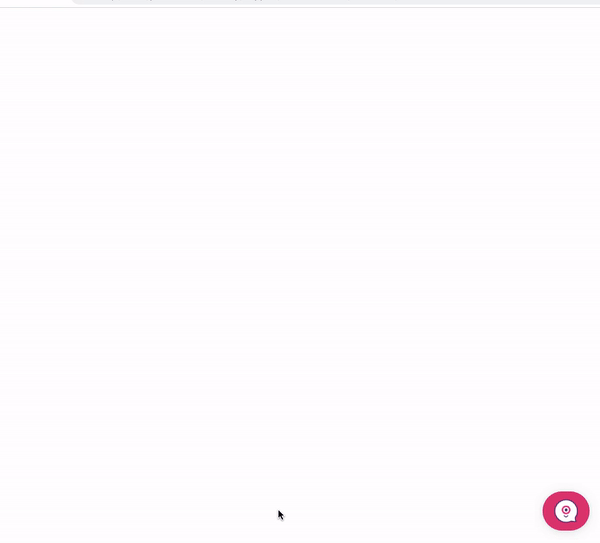
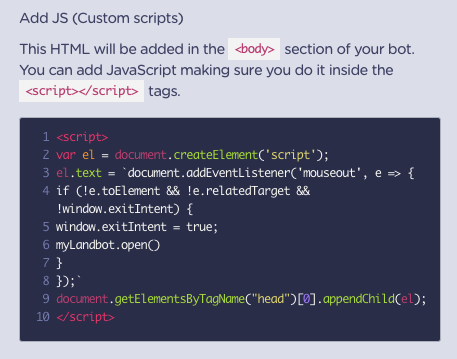
Here is the code:
<script>
var el = document.createElement('script');
el.text = `document.addEventListener('mouseout', e => {
if (!e.toElement && !e.relatedTarget && !window.exitIntent) {
window.exitIntent = true;
myLandbot.open()
}
});`
document.getElementsByTagName("head")[0].appendChild(el);
</script>
Another example
Add a Chart (with Chart JS library) in your Landbot
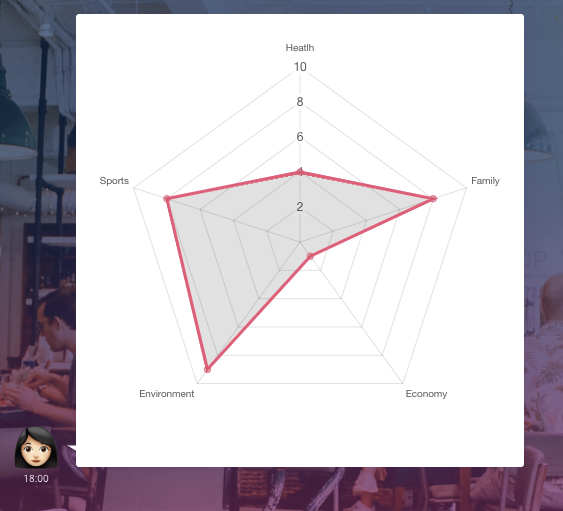
To communicate between Landbot and the Embedding Page (window scope)
This method consists of using the Window scope to call variables and functions in the parent site in this simple way, using the Code block. This is the best way for example to track events with systems like Google Tag Manager
Example: Trigger a function located in the parent site where the bot is embedded
Let's say that you have a function, that changes the color of the background of your site, depending on the user interaction with your bot. And to do that you have a function like this in the parent site (window scope):
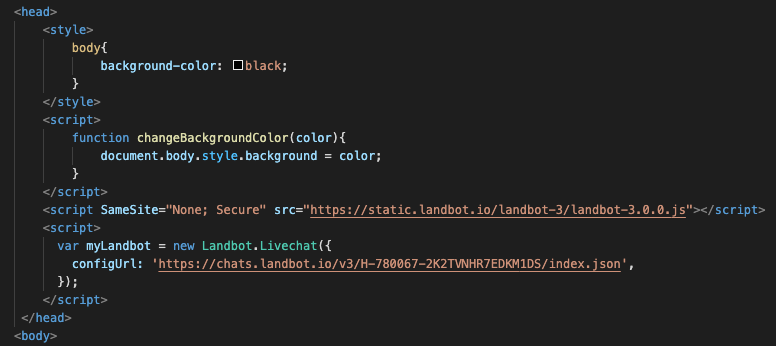
function changeBackgroundColor(color){
document.body.style.background = color;
}
Then, to trigger this event, and change the color from Landbot, you will add this in a code Block:
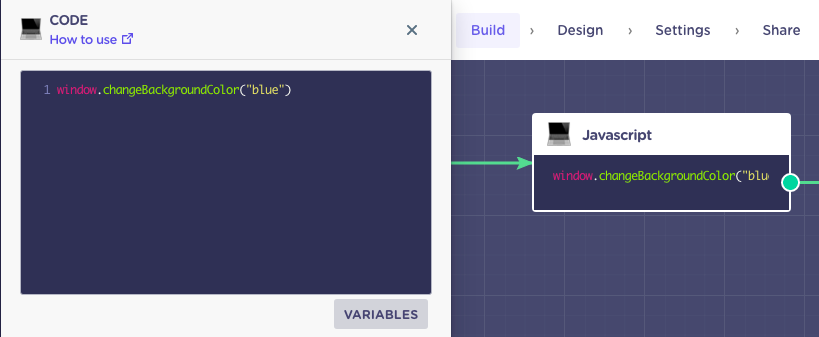
window.changeBackgroundColor("blue")
Even, you can use Landbot variables as a parameter to be passed. So if you had in the variable @color, the value, you can pass it like this:
window.changeBackgroundColor("@{color}")
Here is how it will look like in the builder:
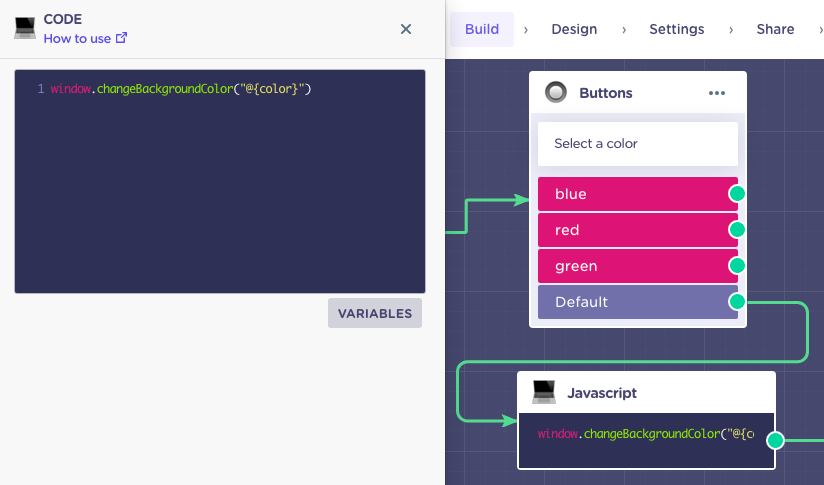
And here is how the user will see it:
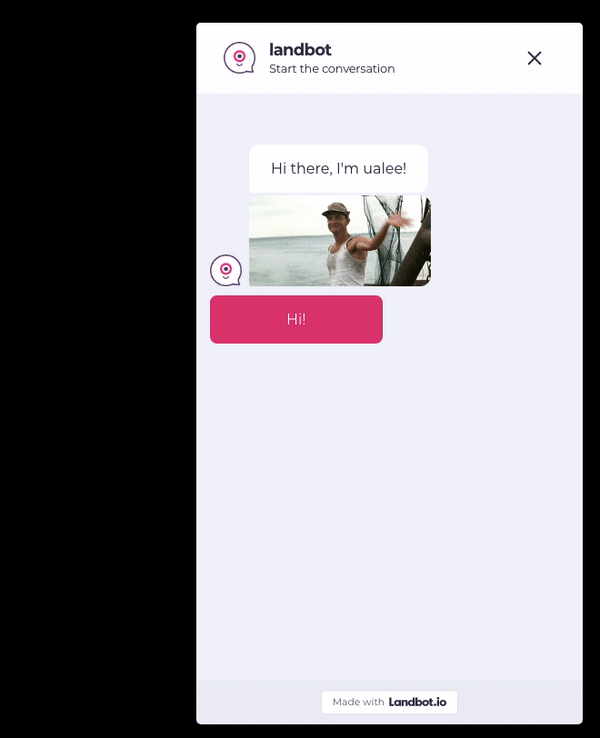
Example: Redirect user
Like in this example, where we can redirect to another URL in the same tab:
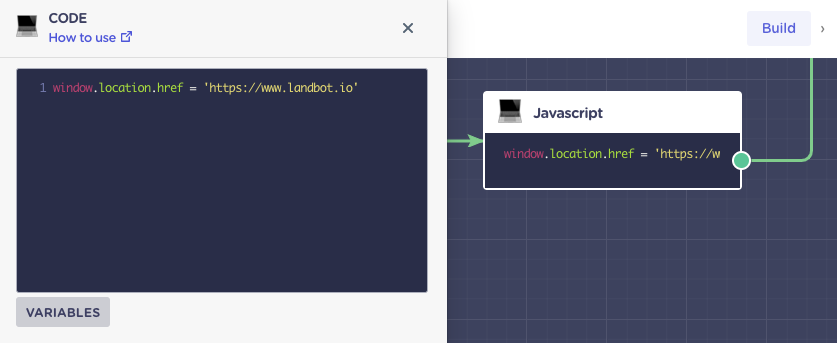
window.location.href = 'https://www.landbot.io'
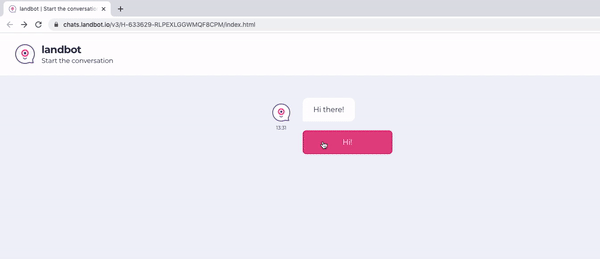
This method is also valid for not embedded Landbots (native)
To communicate between the Embedding Page to Landbot
On some occasions, we might need to pass information between Landbot and the site where the bot is embedded or another way round or even both ways. In this article, we are going to show you how to do this. Bear in mind that this is only applicable to embedded Landbots, and cannot be tested on the preview page.
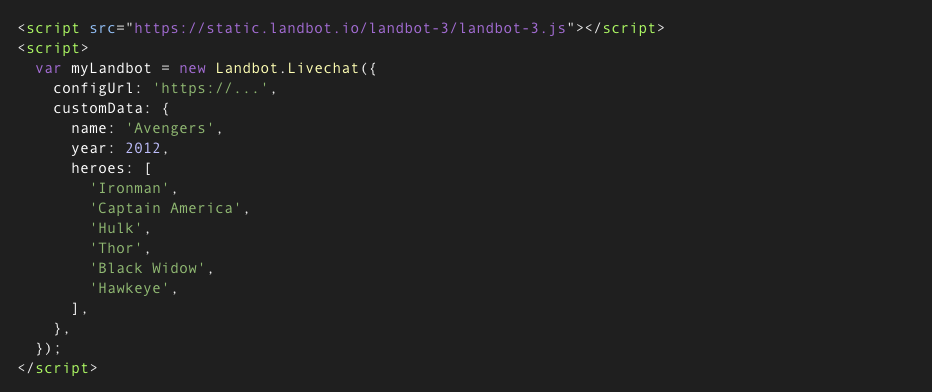
There are different methods to pass such information:
Embed Snippet Custom Data
Here we add the "customData", key to the embed snippet like in the example below, where we are giving values to the variables @name, @year, and @heroes.
<script src="https://static.landbot.io/landbot-3/landbot-3.js"></script>
<script>
var myLandbot = new Landbot.Livechat({
configUrl: 'https://...',
customData: {
name: 'Avengers',
year: 2012,
heroes: [
'Ironman',
'Captain America',
'Hulk',
'Thor',
'Black Widow',
'Hawkeye',
],
},
});
</script>
Obviously, you can also assign variables instead of static values:
<script>
var userName = 'Avengers';
var userYear = 2012;
var userHeroes = [
'Ironman',
'Captain America',
'Hulk',
'Thor',
'Black Widow',
'Hawkeye',
]
</script>
<script src="https://static.landbot.io/landbot-3/landbot-3.js"></script>
<script>
var myLandbot = new Landbot.Livechat({
configUrl: 'https://...',
customData: {
name: userName,
year: userYear,
heroes: userHeroes,
},
});
</script>
Custom Data method (setCustomData)
This a handy method in case you need to pass the information to the bot, once the bot is already loaded and you cannot use the embed snippet.
In the example below, we assign to the variable @heroes an array as a value
<script src="https://static.landbot.io/landbot-3/landbot-3.js"></script>
<script>
var myLandbot = new Landbot.Livechat({
configUrl: 'https://...'});
</script>
<script>
myLandbot.setCustomData({heroes:[
'Ironman',
'Captain America',
'Hulk',
'Thor',
'Black Widow',
'Hawkeye',
]})
</script>
API Custom Data
Another alternative that is more flexible and might be useful for very specific cases would be to pass the values via API using the CustomerFields method, for more information please check here
In case you need further information about how to handle Javascript inside Landbot, please check our dev docs
To trigger actions in Embedded bots from the container page
You can also trigger a different number of actions like in the examples below
Open LiveChat with on click of a button
Below you can find the code you need to trigger and open your landbot, embedded as a LiveChat or Pop up mode, by clicking a button.
<html>
<body>
<div id="wrapper">
<button class="openbot">Open Bot</button>
</div>
<script type ="text/javascript" src="https://static.landbot.io/landbot-3/landbot-3.0.0.js"></script>
<script>
var myLandbotpop = new Landbot.Popup({
configUrl: 'https://chats.landbot.io/v3/H-642181-Q42D49PVMDCXYYL8/index.json'
});
var buttonOpen = document.getElementsByClassName("openbot")[0];
buttonOpen.addEventListener('click', (event) => {
event.preventDefault();
myLandbotpop.open()
});
</script>
</body>
</html>
As you can see we are using the method "open()" to open the bot. For more information, about this methods, please check here
To hide the widget, you must add the code below in the Design / Advanced / Add CSS section:
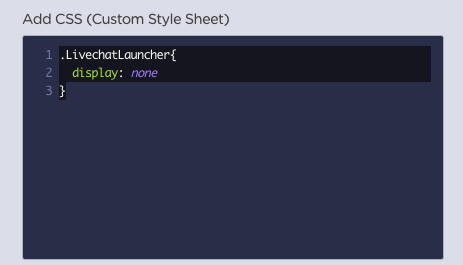
.LivechatLauncher{
display: none
}
Open LiveChat and trigger specific flow
With one click on the parent page, we will open bot and "Send" a user to a specific point in the flow with Javascript
Here is the sample set up inside in the builder:
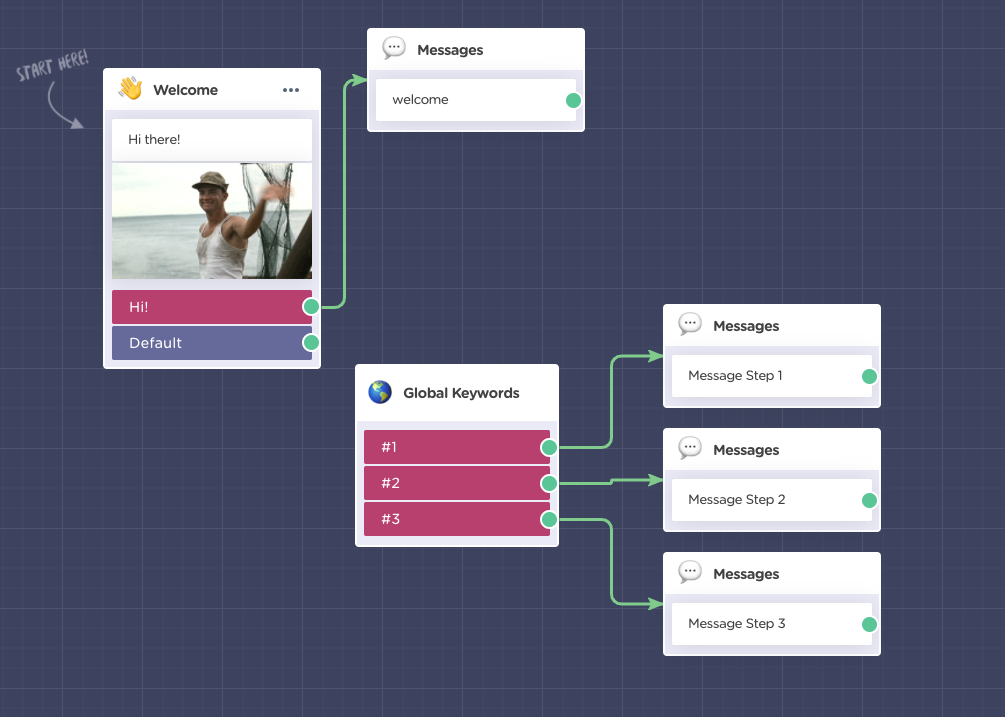
1. We will use Global Keywords, to set up the flows where we want to redirect the user.
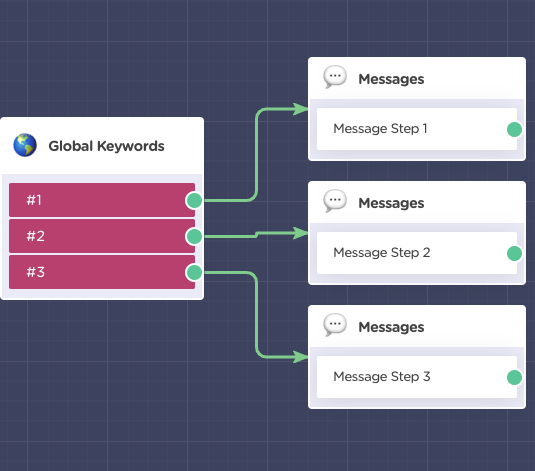
Note: Take note of the content of the Global Keyword has to match the payload key of the message to be sent, as you can see in the next example.
2. Now in the parent page, we need to set up the event listener to trigger, that will open the bot and move the user to the desired node
myLandbot.onLoad(function(){
document.getElementsByClassName("openbot")[0].addEventListener("click", function(event){
event.preventDefault()
myLandbot.open();
setTimeout(function(){
myLandbot.sendMessage({ type: 'button', message: 'Go to Step 1', payload: '#1' })
},500)
});
As you can see we are using two methods "onLoad" (to trigger any desired function once the bot is loaded) and "open" (to open the bot). For more information, about this methods, please check here
Here is an example:
See the Pen Open bot and "Redirect" user with onclick in container site Landbot 3 by Pau (@landbot_help) on CodePen.
Landbot 3 SDK
For advanced Javascript, please check our developers documentation here