Build a bot
Getting started
Basics and builder
Managing Data in Your Chatbot: A Guide to Using Fields
Builder's keyboard shortcuts and mechanics! 🏗
Builder Interface
How to "debug" (troubleshoot) your bot's flow to spot possible errors (for non coders)
Starting Point
How to disable a bot
Bricks
Bot's Settings
Bot General Settings
Second Visit Settings
Custom System Messages
Hidden Fields (Get params / UTMs from url and use it as variables)
Landbot native SEO & Tracking tools
Typing Emulation (Message Delay)
Build it for me!
Getting started - build a bot
Messages, Questions and Logic & Technical blocks
Messages
Media Block
Media block
How to display images with a variable URL source
How to embed a .gif file inside a message
Different ways to embed Videos in Landbot
Display video and hide button to continue until video has ended
Send a Message block - Simple Message
Goodbye block
Question blocks
Date Block
Email Block
Form Block
Scale Block
Buttons block
How to set up Multiple Choice questions
Multi-Question block
Question: Address block
Question: Autocomplete block
Question: File block
Ask for a name
Question: Number block
Question: Phone block
Question: Picture Choice block
Question: Rating block
Ask a Question Block
Question: URL block
Question: Yes/No block
Logic & Technical blocks
Code Blocks
Dynamic Data
Dynamic Data (Arrays)
Get the array's index of the user selection and extract information from array
Formulas
How to Perform Basic Calculations
Get started with the Formulas block
Formulas Blocks Dashboard
Formulas - Regex
Formulas - Date
Formulas - String
Formulas - Logical
Formulas - Math
Formulas - Object
Formulas - Comparison
Formulas - Array
Persistent Menu
Trigger Automation
Webhook
Webhook Block Dashboard
Webhook Block: Simplify API Integrations in Landbot
How to TEST your Http Request (Webhook block)
Landbot System Fields
Set a Field block
Any of the above Output
Global Keywords 🌍
Keyword Jump
Lead Scoring block
Jump To block
AB Test
Conditions block
Conditions block II (with Dates, Usage and Agents variables)
Close Chat block
How to ask a question based on a variable not being set (empty URL params)
Business Hours block
Custom Goals
Note block
Share & Embed
Redirect Users
How to open a new URL in another tab (window)
How to redirect visitors to a URL (web only)
Conditional Welcome (Non-embedded bots)
How to add a Click-to-Call/Email/WhatsApp button
Redirect User Based on Language Input (DeepL)
Generate a URL that has variables from user answers
Popup on Exit Intent
Share
Customized Embed Actions
How to redirect user to another url in your site with Livechat open to continue conversation
How to Detect Visitors Browser
Customize and embed your WhatsApp Widget
Modifying Embed Size
Conditional Welcome: Direct the Flow of Your Bot Based on External Parameters
Detect if bot was opened
Customized Behavior in Mobile Browsers
Load script and display bot on click button
Launch Bot On Exit Intent
Display Bot During Business Hours Only (Livechat & Popup)
Open / Close a Web bot (embedded)
Launching a bot depending on browser language
How to pass WordPress logged in user data to Landbot
Set the flow depending on the url path (for embedded landbots)
How to launch a Landbot by clicking a button
Open LiveChat bot as soon as page loads
Detect if a visitor is on Mobile/Tablet or Desktop
Embed
Embed the bot into your Website
Embed Landbot in an iframe
Landbot in Wix
Landbot in your web with Google Tag Manager
Landbot in Webflow
Embed in Sharetribe
Landbot in Shopify
Embedding Landbot in Carrd
Landbot in Wordpress
Landbot in Squarespace
Customizing the Proactive Message
Design section (web bots)
Verification & Security
Account Settings and Billing
Billing
Account Settings
Account Settings
Account Homepage
Dashboard
Common reasons for not receiving account activation email
Privacy and Security
Landbot's Privacy & Security Policies
Compliance with the GDPR - General Data Protection Regulation
Landbot's Abuse Policy - How to Report an Abusive Use of Landbot
Delete Customer Data in Flow
Teammates
Agent Status and Log out
Roles & Permissions for Teammates
Manage Landbot Teammates - Add and Customize Agents
Notifications section
Startup Discounts
NGOs and Educational Organizations Discount
Human Takeover & Inbox
Integrations with Landbot
Native Integrations
Airtable
Airtable integration block
Get data filtered from Airtable with a Brick- Shop example
20 different ways to GET and filter data from Airtable
How to add/update different field types in Airtable (POST, PATCH & PUT)
How to Create, Update, Retrieve and Delete records in Airtable (POST, PATCH, GET & DELETE)
Get more than 100 items from Airtable
Insert Multiple Records to Airtable with a Loop
How to Get an Airtable Token
Advanced filters formulas Airtable block
Airtable usecase: Create an event registration bot with limited availability
Update Multiple Records in Airtable Using a Loop
Reservation bot with Airtable
Calendly
Dialogflow
Dialogflow & Landbot course
Dialogflow & Landbot intro: What is NLP, Dialogflow and what can you do with it?
Dialogflow & Landbot lesson 1: Create your first agent and intent in Dialogflow
Dialogflow & Landbot lesson 2: Get the JSON Key
Dialogflow & Landbot lesson 3: Setting up of Dialogflow in Landbot
Dialogflow & Landbot lesson 4: Training phrases and responses for a FAQ
Dialogflow & Landbot lesson 5: Entities and Landbot variables
Dialogflow & Landbot lesson 6: Redirect user depending on Dialogflow response parameters (intent, entities and more)
Learn more about Dialogflow - Courses and communities
Integrations > Dialogflow Block
How to extract parameters from Dialogflow response with Formulas
Dialogflow Integration Dashboard
Dialogflow in Unsupported Languages (& Multilingual)
Dialogflow - How to get JSON Key
Google Sheets
Google Sheets (Insert, Update and Get data)
How to use Google Sheets to create a simple verification system for returning visitors
How to Upload Document & store link
How to give unique Coupon Codes (with Google Spreadsheets)
Google Sheets Integration Dashboard
How to insert a new row with data and formulas in Google Sheets
How to use Google Spreadsheet as a Content Management System for your bot
Hubspot
MailChimp
Salesforce
Segment
SendGrid
Slack
Stripe
Zapier
How to Configure the Landbot and Zapier Integration Using the Zapier Block
Zapier Integration Dashboard
How to insert a row to Google Spreadsheet by Zapier
How to generate a document with PDFMonkey by Zapier
Send WhatsApp Message Templates from Zapier
How to send an email with Gmail by Zapier
Get Opt-ins (Contacts) from Facebook Leads using Zapier
How to extract data from an external source with Zapier and use it in Landbot
Zapier trigger
How to complete a digital signature flow by Zapier
Make a survey with Landbot and display the results in a Notion table using Zapier
Zendesk
Non-Native Integrations
ActiveCampaign
Google Calendar
Google Fonts
Google Maps
Embed Google Maps
Google Maps API Key for Address block
Extract Data With Google Maps Geocoding API
Calculate Distances With Google Maps API
Google Meet
IFTTT
Integrately
Intercom
Make
Connecting MySQL with Make.com (formerly Integromat)
How to upload a file to Google Drive using Make.com (formerly Integromat)
Send WhatsApp Message Template from Make
Make Integration With Trigger Automation Block
How to send an email through Sendinblue by Make.com (formerly Integromat)
Get Opt-ins (Contacts) from Facebook Leads using Make
How to extract data from an external source with Make.com and use it in Landbot
OCR
Pabbly
Paragon
Pipedream
PDF Monkey
Store Locator Widgets
Xano
Zendesk
WhatsApp Channel
Getting started!
WhatsApp Testing
Build a WhatsApp Bot - Best Practices and User guide
Build a WhatsApp Bot - Best Practices for Developers
Types of Content and Media you can use in WhatsApp 🖼
1. WhatsApp Article Directory
2. WhatsApp Integration FAQ's
Adding & Managing your WhatsApp Channel
Facebook Business Verification - Best Practices 🇬🇧
WhatsApp Number Deletion (WA Channel management)
Adding a WhatsApp number to your account
WhatsApp’s Messaging Policy: New Accepted Industry verticals
Meta processes guide: FBM verification, Official Business Account (OBA) requests, Appeals
Additional Number integration: Limitations and Requirements (Number integration)
Existing WhatsApp Number Migration
Key Insights for Migrating to WhatsApp Business API Cloud
How to's, Compatibility & Workarounds
WhatsApp bots - Feature Compatibility Guide
WhatsApp - How to direct a user through a different bot flow on their second visit
WhatsApp - Get user out of error message loop
Getting Subscribers: Opt-in, Contacts
How to get Opt-ins (Contacts) for your WhatsApp 🚀
WhatsApp Quality - Best Practices
Opt-In block for WhatsApp 🚀
Opt-in Check Block
WhatsApp Channel Settings
Parent Bot/Linked Bot - Add a main bot to your WhatsApp number
WhatsApp Channel Panel (Settings)
Growth Tools for WhatsApp
Duplicating bots
Messaging and contacting your users
WhatsApp Campaigns 💌
WhatsApp's Message Templates
Audience block
WhatsApp Marketing Playbook: Best Practices for Leadgen
WhatsApp Error Logs: Troubleshooting guide
Audiences
WhatsApp for Devs
How to calculate the number of days between two selected dates (WhatsApp)
Creating a Loop in WhatsApp
Recognise the users input when sending a Message Template with buttons
Trigger Event if User Abandons Chat
Calculate Distances in WhatsApp
Send Automated Message Templates based on Dates
Trigger Event if User Abandons Chat Using AWS
reply from Slack: How to create an integration to allow agents reply WhatsApp users from Slack (with Node JS)
Set Up a Delay Timer in Bot
Notify Teammates of Chat via WhatsApp
Native blocks for WhatsApp
Other Channels - Messenger and APIChat
Facebook Messenger
The Facebook Messenger Ultimate Guide
Types of content you can use in Messenger bots 🖼
How to Preview a Messenger bot
Facebook Ad connected to Messenger Bot
API Chat (for Developers)
AI in Landbot
Custom AI Integrations
Create a JSON format response from OpenAI in WhatsApp
Connect OpenAI Assistant with Landbot
AI in WhatsApp
How to build a FAQ chatbot with GPT-3
GPT-4 in Landbot
OpenAI
Prompt Engineering for GPT-3
Build a Customer Service Bot with ChatGPT and Extract Information
Google Gemini in Landbot
Build a Chatbot with DeepSeek
Native AI Features
Metrics and Data Management
Metrics Section
How to export the data from your bots
Export data: How to open a CSV file
Bot's Analyze Section
For Developers & Designers
JavaScript and CSS
CSS and Design Customizations
Design Customizations
Advanced (Custom CSS & Custom JS)
Components CSS Library Index
Background Class CSS
Identify Blocks CSS
Buttons Class CSS
Header Class CSS
Media Class CSS
Message Bubble Class CSS
Miscellaneous Classes CSS
CSS Customization Examples: "Back to School" Theme
Get started guide for CSS Design in Landbot
CSS Customization Examples: Call To Action: WhatsApp
CSS Examples: Lead Gen
CSS Customization Examples: "Translucid"
CSS Customization Examples: "Minimalist" Theme
Dynamic Data CSS
Form Block CSS
CSS for Typewriter Effect
CSS Customization Examples: Carrd Embed Beginner
Dynamically Change a Bot's Background
Proactive Message Customizations with Javascript and CSS
Landbot v3 - Web CSS - RTL
Multi Questions CSS
CSS Customization Examples: Video Bubble
Dynamically Change Any Style
CSS Customization Examples: CV Template
Change Landbot custom CSS dynamically from parent page onload
Widget/Bubble Customizations with Javascript and CSS
JavaScript
How to change Avatar dynamically (Landbot v3)
Javascript in WhatsApp
Landbot v3 JavaScript Integration
Different ways to format numbers with JS
How to display an HTML Table and a List in Landbot v3 web
Trigger a Global Keyword with JS (web v3)
Create Dynamic Shopping Cart with JS and CSS
Add a Chart (with Chart JS library) in your Landbot
Different ways to format numbers with JS (WhatsApp)
Pop up modal to embed third party elements
Landbot API
Send WhatsApp Messages with Landbot API
How to "send" a user to a specific point in the flow with Javascript and with the API
APIs
Get Opt-ins (Contacts) using Landbot API
MessageHooks - Landbot Webhooks
Resume flow based on external process with Landbot API (Request, Set, Go)
Tracking
Google Analytics - Track Events (Not embedded)
Google Analytics - Track Events (Embedded)
Meta Pixel - Track Events (only Embedded)
How Track Google Analytics Events in Landbot with Google Tag Manager (GTM)
Google Adwords - How to track Google Adwords in Landbot
Set a timer to get the time spent during the flow
Workarounds and How To's
Workflows
How to build an event registration Landbot (to be used in one screen by many attendees) (web only)
How to let user select a time of booking (with a minimum 45 minutes notice)
Send Files Hosted in Landbot to Your Google Drive with Make
Two-Step Email Verification
Fixing Web Bot Loading Issues for iOS Devices in Meta Campaigns with Disclaimers
Progress Bar Workaround
How to set up questions with a countdown
Creating a Simple Cart in WhatsApp
More Topics
Table of Contents
- All Categories
- WhatsApp Channel
- WhatsApp for Devs
- reply from Slack: How to create an integration to allow agents reply WhatsApp users from Slack (with Node JS)
reply from Slack: How to create an integration to allow agents reply WhatsApp users from Slack (with Node JS)
Updated
by Pau Sanchez
Bots are great at automating conversations, but sometimes, users need human intervention. Landbot offers a Chat section, where agents can reply from, even though, in some cases a company prefer to deal directly from messaging platforms like Slack. In this article we explain how to set up an integration for WhatsApp bot users, to be replied from Slack thanks to Landbot API and MessageHooks.
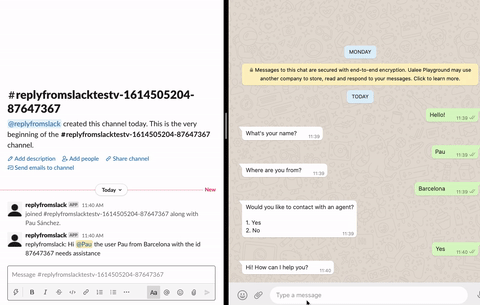
replyfromslack description
We are going to describe in this article the steps needed to set up the integration in Landbot and in Slack, and the code from the repository you will need
We will work based on:
Landbot API to send messages to the end user: Link
Landbot MessageHooks to get requests on every event going on in the specific channel where the bot runs: Link
Slack API to send messages to the Slack agent: Link
Slack App Event Subscription to get request on every event going on in Slack
Functionalities and logic of replyfromslack
- The automated part of the conversation from the user with the bot won't be sent to the other platform (Slack)
- Once the user requires Human Intervention, by going through a specific flow, will trigger the creation of a conversation in the other platform (Channel in Slack)
- Part of the bot has a flow designed to stop user of more inputs, trigger the start of the bot, with the Slack variable loop
- Once the first message is sent from the agent in Slack, the Agent API will takeover, and no more automated messages will be displayed.
- Once both conditions are met (Slack Channel created, first agent interaction and message being sent to the user, therefore Agent API takeover) the app as a middleservice will take care of:
1. Reading messages/events from Slack, parse them, filter those that are meant to be sent to Landbot and send them
2. Reading messages/events from Landbot, parse them, filter those that are meant to be sent to Slack and send them
- Messages can be in different formats from both platforms, and can be sent in different ways depending on the platform
- Once the conversation has ended, the Agent, with a specific keyword ("CLOSECHAT"), will be able to :
1. Delete from their account in Landbot a session variable
2. Send the user to a specific step in the flow of the bot, so once the user restarts interacting it will be with the bot
3. Delete the Slack channel to avoid clutter.
Set up in Slack
Now is time to create and app in Slack, that will be installed in the workspace, make sure you have admin rights to do so.
Create APP
Go to https://api.slack.com/apps
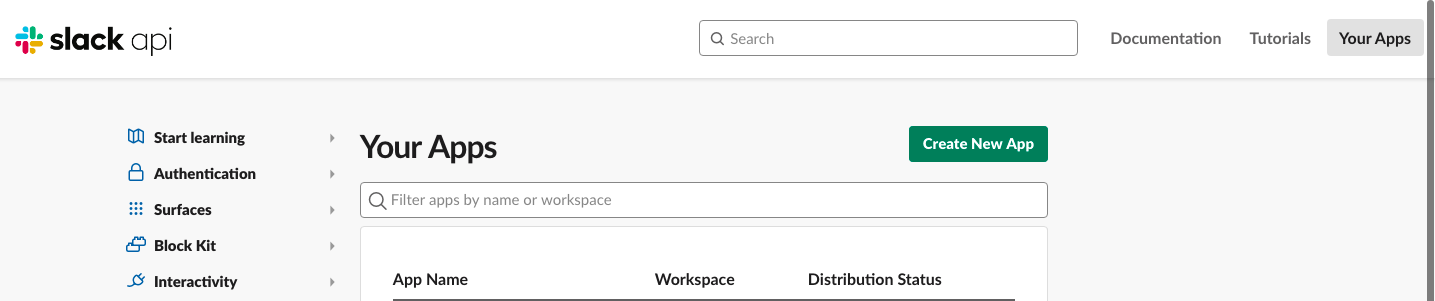
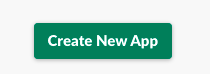
Press Create New App and this screen will appear:
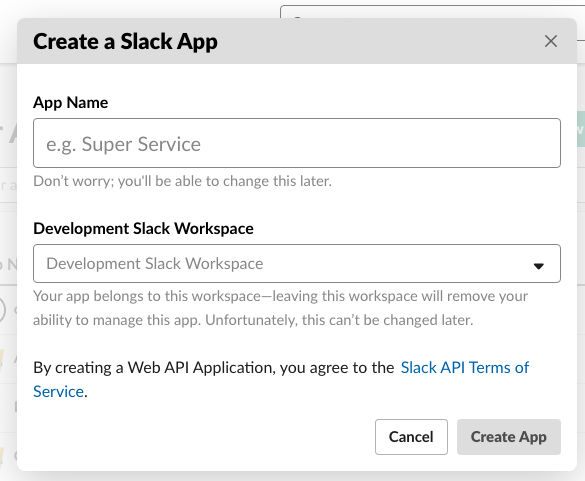
Fill the details and select a Slack Workspace, and press Create App
Once your App has been create select Basic Information:
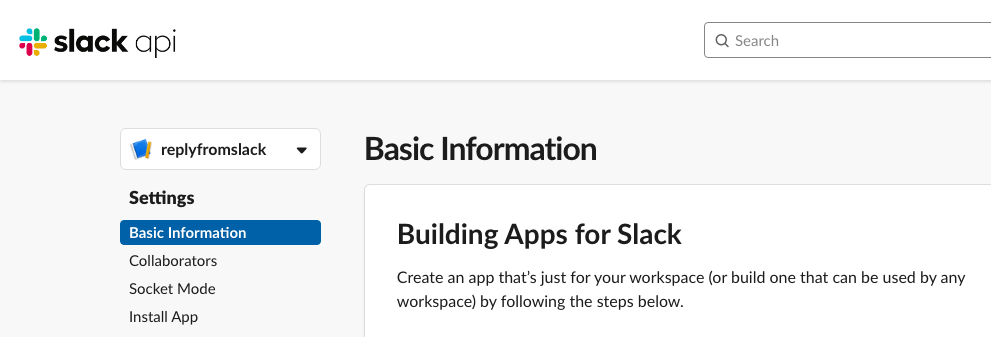
Set the scopes
Select from the side panel OAuth & Permissions:
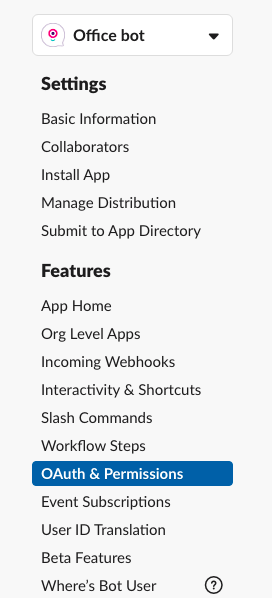
Scroll down to Scopes:
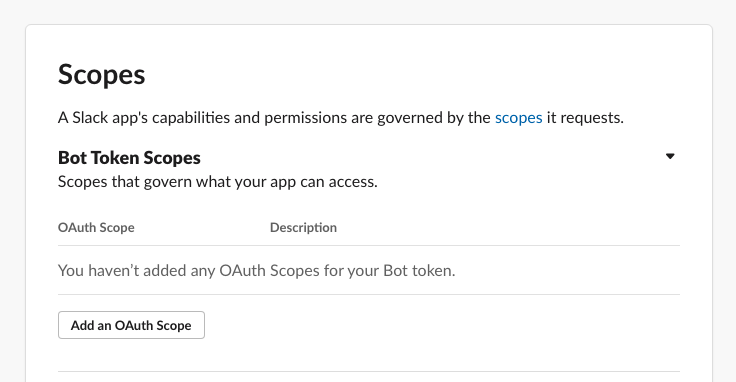
Under "Bot Token Scopes" press "Add an OAuth Scope":
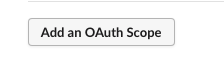
For the Slack App to reply from, we will need the following scopes to be added:
- channels:manage
- channels:read
- chat:write
- chat:write.customize
- files:read
- files:write
- groups:read
- groups:write
- im:history
- im:read
- im:write
- mpim:read
- mpim:write
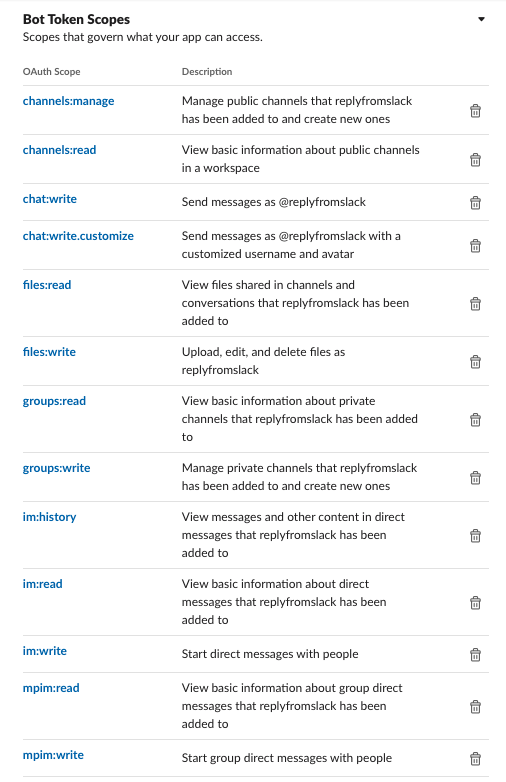
Scroll up again and select "Install to Workspace":
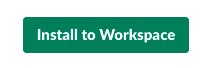
Grant permission to access your workspace:
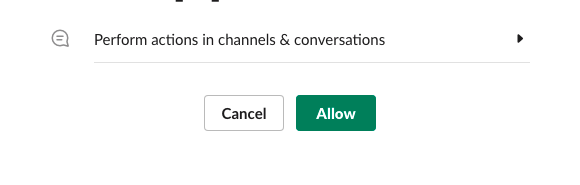
Once installed and allowed, you will have an Auth Token, that we later will use in the config.json file of the app:
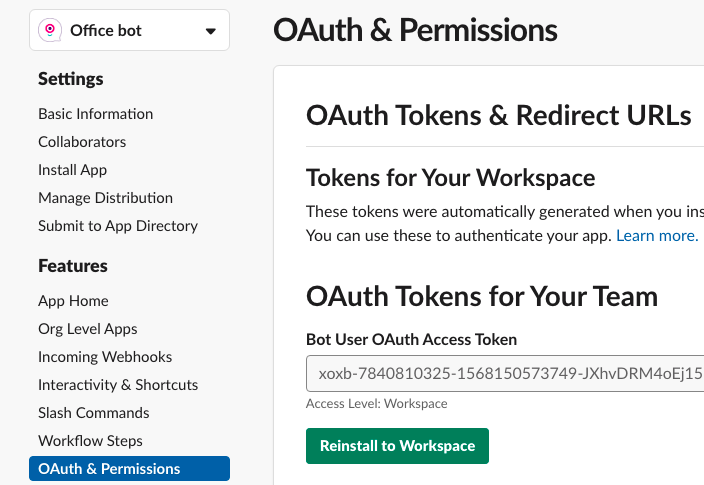
Set up in Landbot
Builder bot logic
First we need to prepare the bot logic and flow to be able to handle the user interaction with the agent. Here is a sample of a basic flow:
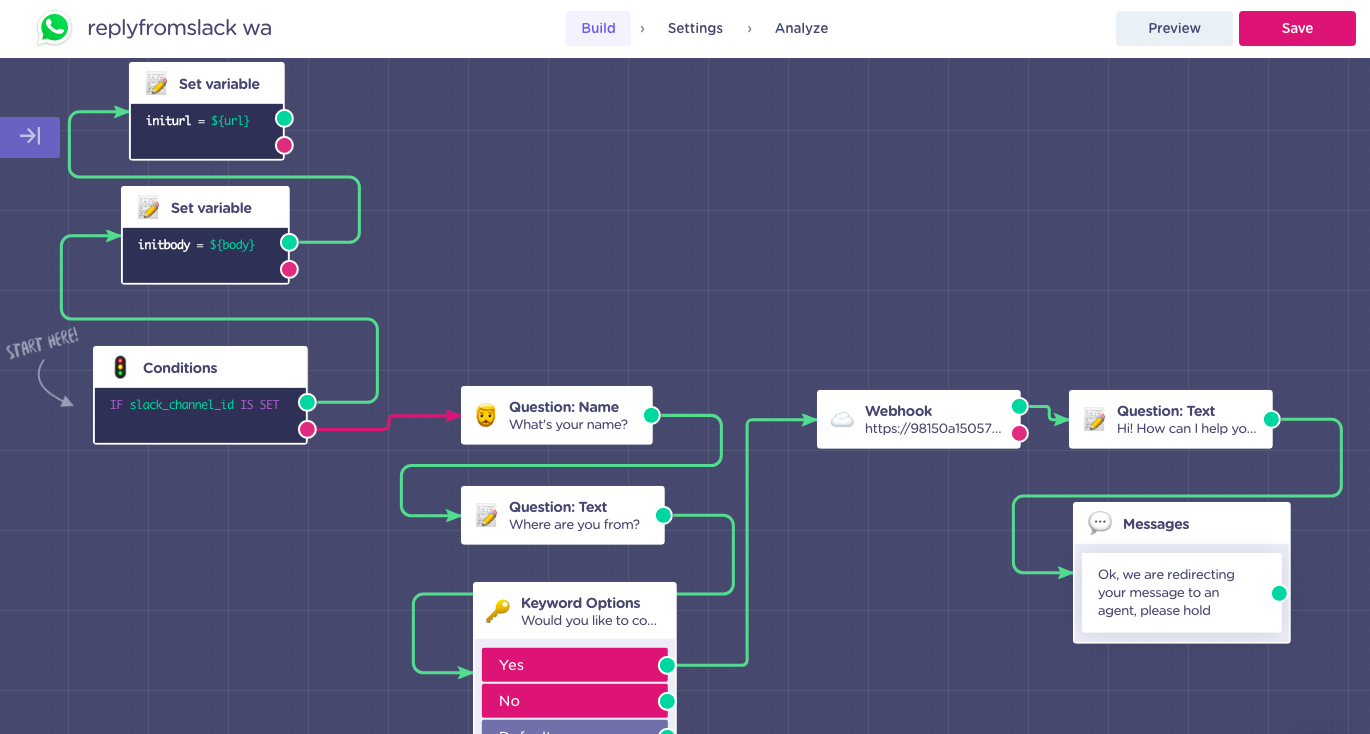
- The start of the bot will be a Conditional block. This will check if the user has already started a conversation with an agent in Slack. If so, it will stop the bot restarting if the agent didn't takeover yet with the first message
The condition IS SET, will check if the variable (in this case @slack_channel_id) has any value. It will only have a value once it goes through the Webhook block, that is right before the first input for the agent
- As a way to capture the input from the user, between the channel creation in Slack and the first input from the user, we will add 2 Set Variable blocks that will store user inputs that come in ${body} for texts and ${url} for any other media hosted in servers
- Now, is the time for the automated part of the conversation, in this example we ask the name (required if we want to display the name in the Slack channel) and other data, that we also will send to the Slack channel.
- Also, as a sample, we added a Keyword Option, to let the user decide if they want to be redirected to an agent
- Now, that we have the confirmation from the user to be redirected to an agent, we need to set up a Webhook block, that will trigger our app to create a channel in Slack and passing some data about the user
As you can see in the example, we are using ngrock to tunel the request to our local server. Notice that we use the path /landbot_trigger
Also, in the body of the webhook we will send some information:
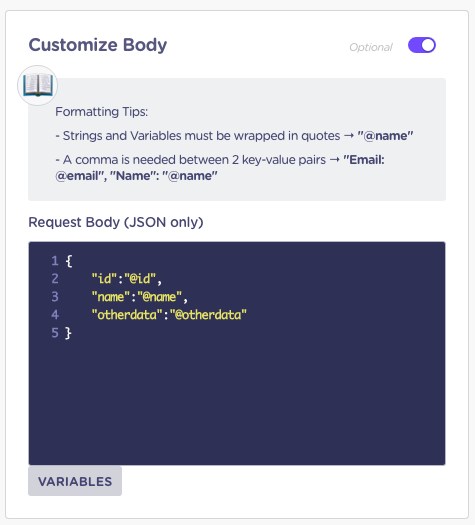
Among the data in the JSON there are:
@id *required to keep the session between the user and the agent
@name *recommended to be able to display the name in the Slack Channel
@otherdata *recommended, in case you want to display some information to the agent in Slack
And finally, we need to Save the Response:
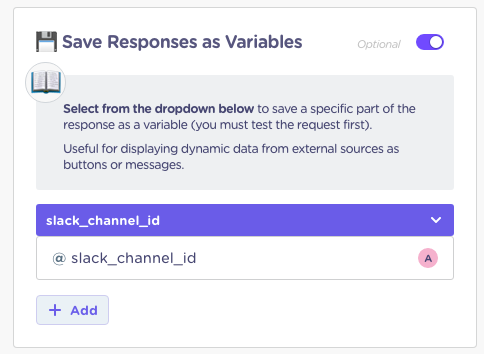
This variable comes from our app, and is the variable that will keep the control if the user is starting a conversation with the Agent.
- Finally, we will add a Question block to allow the user to make the first input that will be sent to Slack and a Send Message block, to display the user, that must wait
Messagehooks
Messagehooks are in Landbot, what "webhooks" are in other platforms. A simple way to subscribe to all the events going on in a specific Channel where the bot runs. This is the main way we will get the inputs from the user. If you want to know more about MessageHooks, please check this article
In case of WhatsApp (Messenger and APIChatbot too) we need to link the bot to a Channel. We will do it from the Channels section:
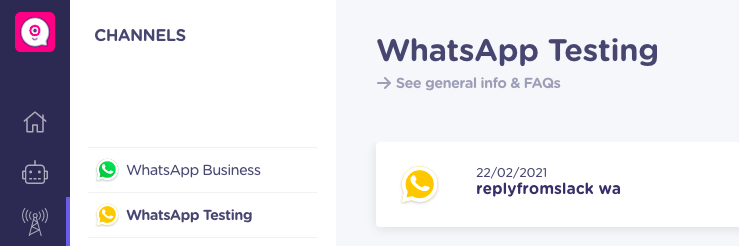
If this step is already done, we will create a MessageHook:
- First we need to go to Integrations:
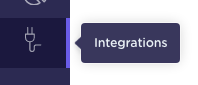
- Select Developer Tools
- Select MessageHooks
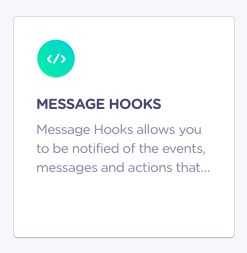
- And again MessageHooks
- Press "Add new message hook"
Here is where we will do 2 things:
- Link the MessageHook to the Channel where the bot is linked
- Add the Hook URL, where all the request will be done
Check that according to our app, we expect to get the requests in the path /landbot_hook
Code Repository
Clone the Github Repository containing the project files "here" and install the npm packages
https://github.com/pausanchez/replyfromslack
App configurations (config.json)
Inside the folder, there is a config.json file, where we will set up the main configurations and data for the app. Here is a description and information of each one:
- landbot_token
example: "123212" *string
This is your Landbot API Token, you will find it in your Landbot Account section (https://app.landbot.io/gui/settings/account)
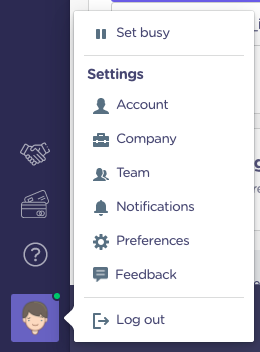
- landbot_bot_id_redirection
example: "849366" *string
That is the bot id, where you will redirect the user back once the agent finishes. The id will be the number in the URL of the bot builder
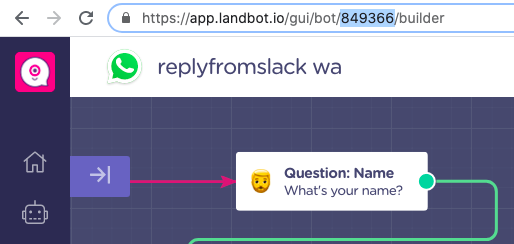
- landbot_node_id_redirection
example: "bot_start" *string
This is the block(node id) where you want to redirect the user once it finished the conversation with the agent. To get the id, press right click on the block in the builder, and press "copy reference":
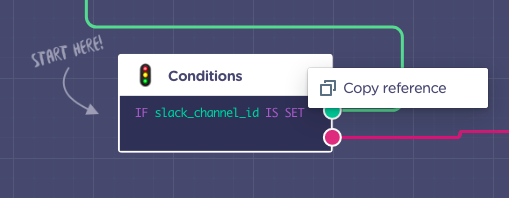
- landbot_control_variable
example: "slack_channel_id" *string
This is the variable we will use for 2 reasons: 1 make sure that we keep the session of the user in Landbot with the right Slack Channel.
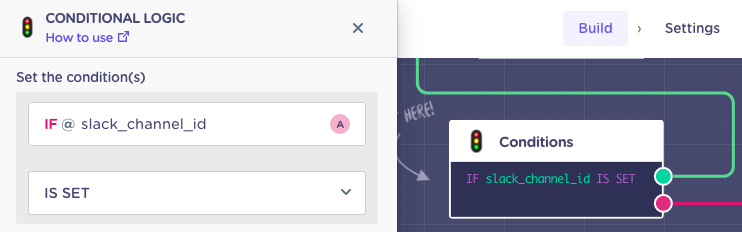
At the same time, we will use that variable to make sure the user only triggers the bot once we delete the variable
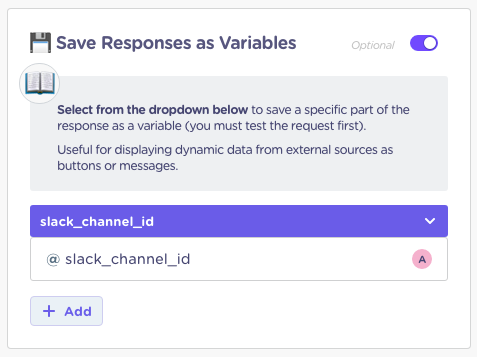
- slack_app_token
example: "xoxb-78403..." *string
That is the slack app Bot User OAuth Token
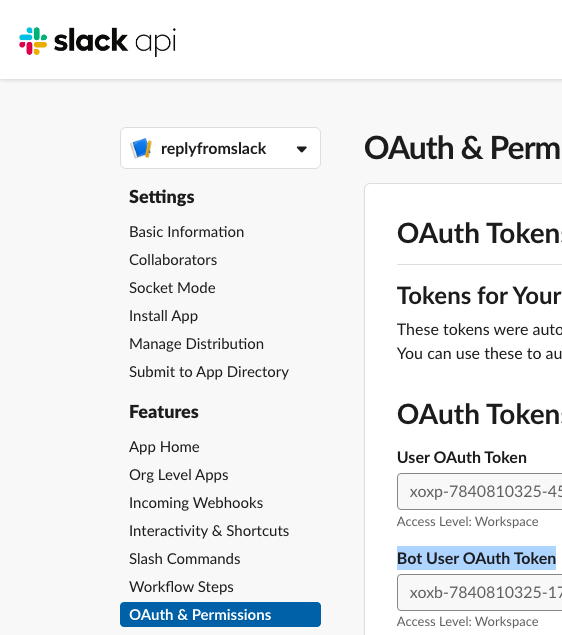
- slack_agent_ids
example: "UD9V5TXXX" *string
That is the id of the Slack users that you want to invite to the channel, so they are notified once a channel has been created.
To get the id of a user in Slack go to the profile of the user and press MORE and select "Copy member ID"
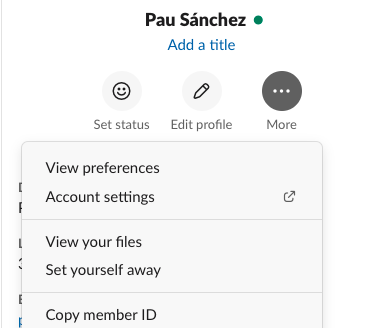
- slack_close_chat_keyword
example: "CLOSECHAT" *string
That is the keyword, that once the agent from Slack sends, it will:
1. Delete the control variable
2. Redirect the user to the desired block we set up
3. Delete the conversation in Slack to avoid clutter
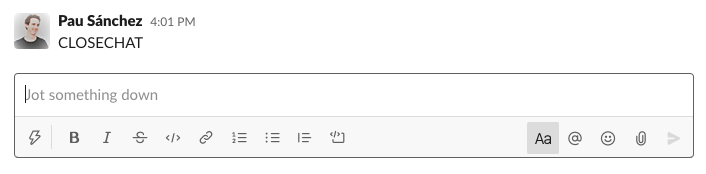
- slack_agent_to_mention
example: "<@UD9V5TXXX>" *string
That is the agent you want to mention in the first sentence
- slack_app_channel_name
example: "replyfromslacktestv" *string
That us the name at the beginning of the Slack channels
- slack_base_channel_name
example: "replyfromslack" *string
That is the name of the Slack app
- slack_icon_pic_src
example: "https://files.com/landbot-icon.png" *string
That is the url of the source of the image you want to use as icon for the user in Slack
- baseURL
example: "https://66699b169dbb.ngrok.io" *string
The url generated by ngrock, used to display the temporary files
Initialize the App
- Trigger ngrock and copy the https url
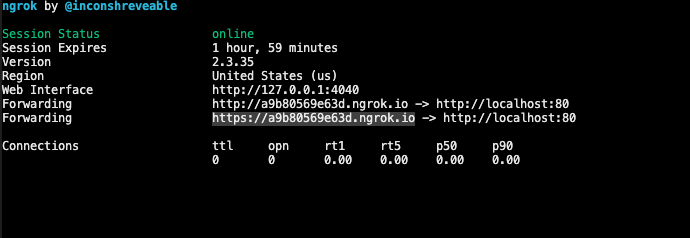
- add the Base url in the config.json file for the temp files:
- Initialise the app

- Go to the https://api.slack.com/apps and select Event Subscriptions
- Add the ngrock url and the path slack_hook:
Once is verified, press SAVE CHANGES
- Go to Landbot MessageHooks and add the endpoint needed with the path /landbot_hook:
- Go to the bot builder, and change the URL with the /landbot_trigger path:
- If is the first time, we will need to finish the set up of the webhook, to save the response. To so we will make a test, with some dummy values, according to the set up in the Body:
And press the Test the request, if everything is set up correctly you will see a 200 status and the response:
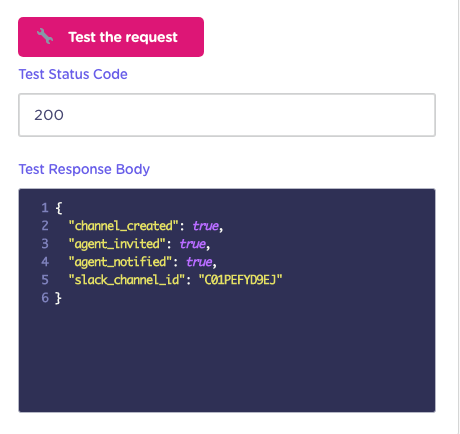
Now, scroll down to save the value of "slack_channel_id" in a Landbot variable:
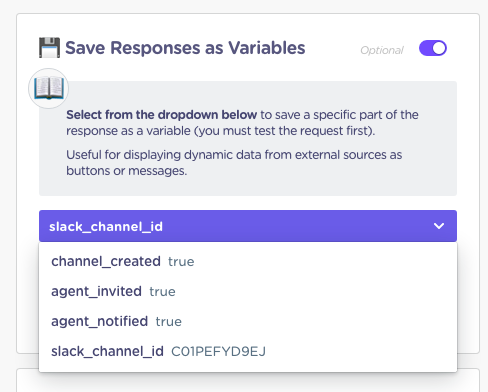
So it will be like this:
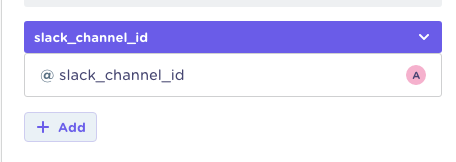
Make sure to save the webhook changes, and the bot changes!
- Also, in Slack it will be generated the first channel:
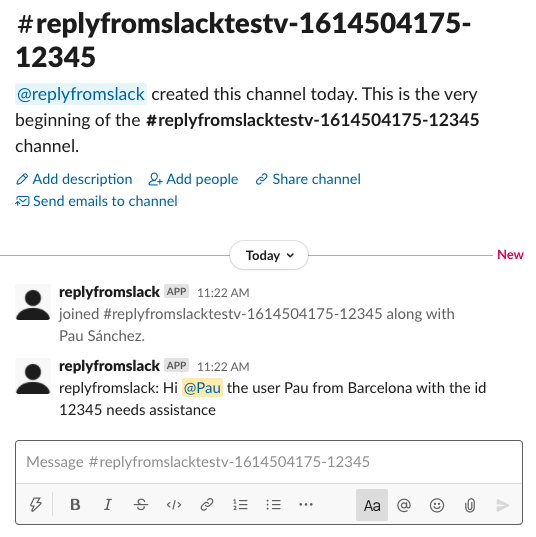
If you want to delete this channel generated during the test of the webhook, you might need to use POSTMAN to make such request:
curl --location --request POST 'https://slack.com/api/conversations.archive?token=<SLACK APP API TOKEN>&channel=<SLACK CHANNEL ID>' \
The Slack Channel Id, can be captured easily from the channel link, just press right click on the channel, and you will be able to capture the url. The numbers at the end are the id of the channel
- That's it, now you are ready to go!
Notes
Media files and documents in the code of the repository are hosted in the local server, open for the developer to set up it's own custom solution and logic.