Build a bot
Getting started
Basics and builder
Managing Data in Your Chatbot: A Guide to Using Fields
Builder's keyboard shortcuts and mechanics! 🏗
Builder Interface
How to "debug" (troubleshoot) your bot's flow to spot possible errors (for non coders)
Starting Point
How to disable a bot
Bricks
Bot's Settings
Bot General Settings
Second Visit Settings
Custom System Messages
Hidden Fields (Get params / UTMs from url and use it as variables)
Landbot native SEO & Tracking tools
Typing Emulation (Message Delay)
Build it for me - Turn Your Idea into a Chatbot (No JSON Needed)
Getting started - build a bot
Messages, Questions and Logic & Technical blocks
Messages
Media Block
Media block
How to display images with a variable URL source
How to embed a .gif file inside a message
Different ways to embed Videos in Landbot
Display video and hide button to continue until video has ended
Send a Message block - Simple Message
Goodbye block
Question blocks
Date Block
Scale Block
Buttons block
Ask for a Name block
Ask for an Email block
Ask a Question block
Question: Phone block
Forms block
How to set up Multiple Choice questions
Question: Address block
Question: Autocomplete block
Question: File block
Question: Number block
Question: Picture Choice block
Question: Rating block
Question: URL block
Question: Yes/No block
Logic & Technical blocks
Code Blocks
Dynamic Data
Dynamic Data (Arrays)
Get the array's index of the user selection and extract information from array
Formulas
How to Perform Basic Calculations
Get started with the Formulas block
Formulas Blocks Dashboard
Formulas - Regex
Formulas - Date
Formulas - String
Formulas - Logical
Formulas - Math
Formulas - Object
Formulas - Comparison
Formulas - Array
Persistent Menu
Trigger Automation
Webhook
Webhook Block Dashboard
Webhook Block: Simplify API Integrations in Landbot
How to TEST your Http Request (Webhook block)
Landbot System Fields
Set a Field block
Any of the above Output
Global Keywords 🌍
Keyword Jump
Lead Scoring block
Jump To block
AB Test
Conditions block
Conditions block II (with Dates, Usage and Agents variables)
Close Chat block
How to ask a question based on a variable not being set (empty URL params)
Business Hours block
Custom Goals
Note block
Share & Embed
Redirect Users
How to open a new URL in another tab (window)
How to redirect visitors to a URL (web only)
Conditional Welcome (Non-embedded bots)
How to add a Click-to-Call/Email/WhatsApp button
Redirect User Based on Language Input (DeepL)
Generate a URL that has variables from user answers
Popup on Exit Intent
Share
Customized Embed Actions
How to redirect user to another url in your site with Livechat open to continue conversation
How to Detect Visitors Browser
Customize and embed your WhatsApp Widget
Modifying Embed Size
Conditional Welcome: Direct the Flow of Your Bot Based on External Parameters
Detect if bot was opened
Customized Behavior in Mobile Browsers
Load script and display bot on click button
Launch Bot On Exit Intent
Display Bot During Business Hours Only (Livechat & Popup)
Open / Close a Web bot (embedded)
Launching a bot depending on browser language
How to pass WordPress logged in user data to Landbot
Set the flow depending on the url path (for embedded landbots)
How to launch a Landbot by clicking a button
Open LiveChat bot as soon as page loads
Detect if a visitor is on Mobile/Tablet or Desktop
Embed
Embed your bot into your website and use a custom domain
Embed Landbot in an iframe
Landbot in Wix
Landbot in your web with Google Tag Manager
Landbot in Webflow
Embed in Sharetribe
Landbot in Shopify
Embedding Landbot in Carrd
Landbot in Wordpress
Landbot in Squarespace
Customizing the Proactive Message
Design section (web bots)
Verification & Security
Account Settings and Billing
Billing
Account Settings
Account Settings
Common reasons for not receiving account activation email
How to create your Landbot account, set it up and invite teammates
Navigating the Landbot Interface 🧭
Privacy and Security
Landbot's Privacy & Security Policies
Compliance with the GDPR - General Data Protection Regulation
Landbot's Abuse Policy - How to Report an Abusive Use of Landbot
Delete Customer Data in Flow
Teammates
Agent Status and Log out
Roles & Permissions for Teammates
Manage Landbot Teammates - Add and Customize Agents
Notifications section
Startup Discounts
NGOs and Educational Organizations Discount
Trial period
AI in Landbot
Landbot AI Agent
AI Agents Overview
How to connect a Chatbot flow to an AI Agent
Exit Conditions
Tips to migrate from old AI Assistants to AI Agents
How to create custom Instructions for your Landbot AI Agent with AI (ChatGPT, Claude...)
Capture, generate and use data with AI Agents
Custom AI Integrations
Create a JSON format response from OpenAI in WhatsApp
Connect OpenAI Assistant with Landbot
AI in WhatsApp
How to build a FAQ chatbot with GPT-3
GPT-4 in Landbot
OpenAI
Prompt Engineering for GPT-3
Build a Customer Service Bot with ChatGPT and Extract Information
Google Gemini in Landbot
Build a Chatbot with DeepSeek
How to Build a Hybrid AI Bot
Integrations with Landbot
Native Integrations
Airtable
Airtable integration block
Get data filtered from Airtable with a Brick- Shop example
20 different ways to GET and filter data from Airtable
How to add/update different field types in Airtable (POST, PATCH & PUT)
How to Create, Update, Retrieve and Delete records in Airtable (POST, PATCH, GET & DELETE)
Get more than 100 items from Airtable
Insert Multiple Records to Airtable with a Loop
How to Get an Airtable Token
Advanced filters formulas Airtable block
Airtable usecase: Create an event registration bot with limited availability
Update Multiple Records in Airtable Using a Loop
Reservation bot with Airtable
Calendly
Dialogflow
Dialogflow & Landbot course
Dialogflow & Landbot intro: What is NLP, Dialogflow and what can you do with it?
Dialogflow & Landbot lesson 1: Create your first agent and intent in Dialogflow
Dialogflow & Landbot lesson 2: Get the JSON Key
Dialogflow & Landbot lesson 3: Setting up of Dialogflow in Landbot
Dialogflow & Landbot lesson 4: Training phrases and responses for a FAQ
Dialogflow & Landbot lesson 5: Entities and Landbot variables
Dialogflow & Landbot lesson 6: Redirect user depending on Dialogflow response parameters (intent, entities and more)
Learn more about Dialogflow - Courses and communities
Integrations > Dialogflow Block
How to extract parameters from Dialogflow response with Formulas
Dialogflow Integration Dashboard
Dialogflow in Unsupported Languages (& Multilingual)
Dialogflow - How to get JSON Key
Google Sheets
Google Sheets (Insert, Update and Get data)
How to use Google Sheets to create a simple verification system for returning visitors
How to Upload Document & store link
How to give unique Coupon Codes (with Google Spreadsheets)
Google Sheets Integration Dashboard
How to insert a new row with data and formulas in Google Sheets
How to use Google Spreadsheet as a Content Management System for your bot
Hubspot
MailChimp
Salesforce
Segment
SendGrid
Send an Email
Sendgrid Integration Dashboard
How to create a custom SendGrid email - (Custom "from" email)
Slack
Stripe
Zapier
How to Configure the Landbot and Zapier Integration Using the Zapier Block
Zapier Integration Dashboard
How to insert a row to Google Spreadsheet by Zapier
How to generate a document with PDFMonkey by Zapier
Send WhatsApp Templates from Zapier
How to send an email with Gmail by Zapier
Get Opt-ins (Contacts) from Facebook Leads using Zapier
How to extract data from an external source with Zapier and use it in Landbot
Zapier trigger
How to complete a digital signature flow by Zapier
Make a survey with Landbot and display the results in a Notion table using Zapier
Custom Integrations
ActiveCampaign
Google Calendar
Google Fonts
Google Maps
Embed Google Maps
Google Maps API Key for Address block
Extract Data With Google Maps Geocoding API
Calculate Distances With Google Maps API
Google Meet
IFTTT
Integrately
Intercom
Make
Connecting MySQL with Make.com (formerly Integromat)
Send WhatsApp Message Template from Make
Make Integration With Trigger Automation Block
How to send an email through Sendinblue by Make.com (formerly Integromat)
Get Opt-ins (Contacts) from Facebook Leads using Make
How to extract data from an external source with Make.com and use it in Landbot
OCR
Pabbly
Paragon
Pipedream
PDF Monkey
Store Locator Widgets
Xano
Zendesk
WhatsApp Channel
Getting started!
WhatsApp Testing
Build a WhatsApp Bot - Best Practices and User guide
Build a WhatsApp Bot - Best Practices for Developers
Types of Content and Media you can use in WhatsApp 🖼
1. WhatsApp Article Directory
2. WhatsApp Integration FAQ's
Adding & Managing your WhatsApp Channel
Facebook Business Verification - Best Practices 🇬🇧
WhatsApp Number Deletion (WA Channel management)
Adding a WhatsApp number to your account
WhatsApp’s Messaging Policy: New Accepted Industry verticals
Meta processes guide: FBM verification, Official Business Account (OBA) requests, Appeals
Additional Number integration: Limitations and Requirements (Number integration)
Existing WhatsApp Number Migration
Key Insights for Migrating to WhatsApp Business API Cloud
How to's, Compatibility & Workarounds
WhatsApp bots - Feature Compatibility Guide
WhatsApp - How to direct a user through a different bot flow on their second visit
WhatsApp - Get user out of error message loop
Getting Subscribers: Opt-in, Contacts
How to get Opt-ins (Contacts) for your WhatsApp 🚀
WhatsApp Quality - Best Practices
Opt-In block for WhatsApp 🚀
Opt-in Check Block
WhatsApp Channel Settings
Parent Bot/Linked Bot - Add a main bot to your WhatsApp number
WhatsApp Channel Panel (Settings)
Growth Tools for WhatsApp
Duplicating bots
Messaging and contacting your users
WhatsApp Campaigns 💌
WhatsApp's Message Templates
Audience block
WhatsApp Marketing Playbook: Best Practices for Leadgen
WhatsApp Error Logs: Troubleshooting guide
Audiences
WhatsApp for Devs
How to calculate the number of days between two selected dates (WhatsApp)
Creating a Loop in WhatsApp
Recognise the users input when sending a Message Template with buttons
Trigger Event if User Abandons Chat
Calculate Distances in WhatsApp
Send Automated Message Templates based on Dates
Trigger Event if User Abandons Chat Using AWS
reply from Slack: How to create an integration to allow agents reply WhatsApp users from Slack (with Node JS)
Set Up a Delay Timer in Bot
Notify Teammates of Chat via WhatsApp
Native blocks for WhatsApp
Other Channels - Messenger and APIChat
Facebook Messenger
The Facebook Messenger Ultimate Guide
Types of content you can use in Messenger bots 🖼
How to Preview a Messenger bot
API Chat (for Developers)
Human Takeover & Inbox
Metrics and Data Management
Metrics Section
How to export the data from your bots
Export data: How to open a CSV file
Bot's Analyze Section
For Developers & Designers
JavaScript and CSS
CSS and Design Customizations
Design Customizations
Advanced (Custom CSS & Custom JS)
Components CSS Library Index
Background Class CSS
Identify Blocks CSS
Buttons Class CSS
Header Class CSS
Media Class CSS
Message Bubble Class CSS
Miscellaneous Classes CSS
CSS Customization Examples: "Back to School" Theme
Get started guide for CSS Design in Landbot
CSS Customization Examples: Call To Action: WhatsApp
CSS Examples: Lead Gen
CSS Customization Examples: "Translucid"
CSS Customization Examples: "Minimalist" Theme
Dynamic Data CSS
Form Block CSS
CSS for Typewriter Effect
CSS Customization Examples: Carrd Embed Beginner
Dynamically Change a Bot's Background
Proactive Message Customizations with Javascript and CSS
Landbot v3 - Web CSS - RTL
CSS Customization Examples: Video Bubble
Dynamically Change Any Style
CSS Customization Examples: CV Template
Change Landbot custom CSS dynamically from parent page onload
Widget/Bubble Customizations with Javascript and CSS
JavaScript
How to change Avatar dynamically
Javascript in WhatsApp
Landbot JavaScript Integration
Different ways to format numbers with JS
How to display an HTML Table and a List in Landbot v3 web
Trigger a Global Keyword with JS (web v3)
Create Dynamic Shopping Cart with JS and CSS
Add a Chart (with Chart JS library) in your Landbot
Different ways to format numbers with JS (WhatsApp)
Pop up modal to embed third party elements
Landbot API
Send WhatsApp Messages with Landbot API
How to "send" a user to a specific point in the flow with Javascript and with the API
APIs
Get Opt-ins (Contacts) using Landbot API
MessageHooks - Landbot Webhooks
Resume flow based on external process with Landbot API (Request, Set, Go)
Tracking
Google Analytics - Track Events (Not embedded)
Google Analytics - Track Events (Embedded)
Meta Pixel - Track Events (only Embedded)
How Track Google Analytics Events in Landbot with Google Tag Manager (GTM)
Google Adwords - How to track Google Adwords in Landbot
Set a timer to get the time spent during the flow
Workarounds and How To's
Workflows
How to build an event registration Landbot (to be used in one screen by many attendees) (web only)
How to let user select a time of booking (with a minimum 45 minutes notice)
Send Files Hosted in Landbot to Your Google Drive with Make
Two-Step Email Verification
Fixing Web Bot Loading Issues for iOS Devices in Meta Campaigns with Disclaimers
Progress Bar Workaround
How to Add User Verification to Your Chatbot
How to set up questions with a countdown
HTML Template for Emails
Creating a Simple Cart in WhatsApp
More Topics
Table of Contents
- All Categories
- Integrations with Landbot
- Custom Integrations
- Google Maps
- Calculate Distances With Google Maps API
Calculate Distances With Google Maps API
Updated
by Abby
By first extracting the data from our user's address and then creating a function we'll be able to calculate the distances in kilometers from one point to another.
Here's what the flow will look like:
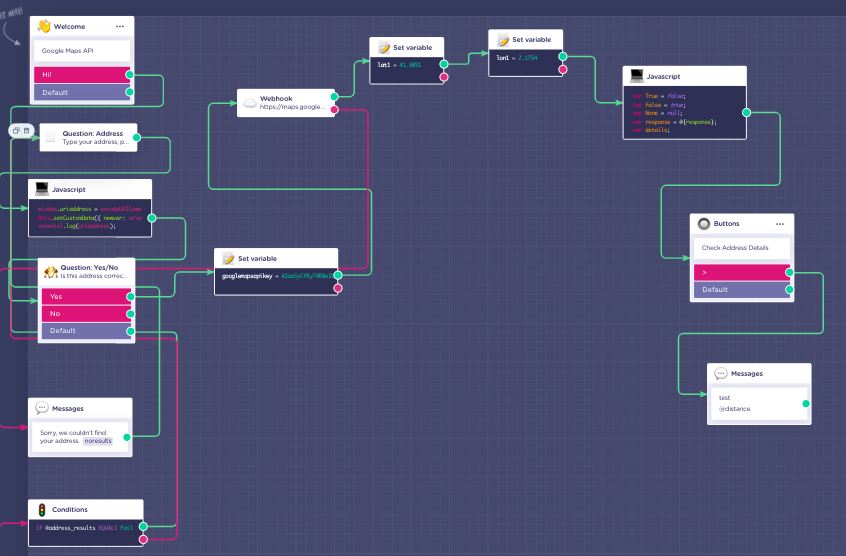
Cloud Console
Go to the Google Cloud Console, enable your APIs, and activate billing.
You'll need to activate 'Places API', 'Maps JavaScript API', and 'Geocoding API'.
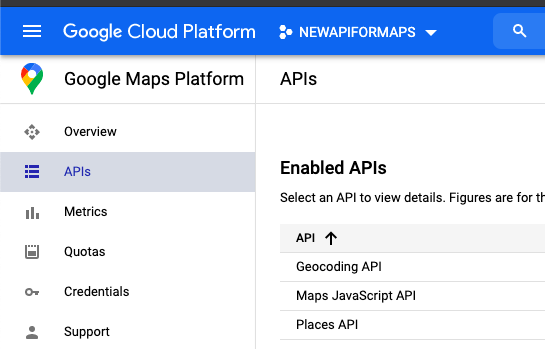
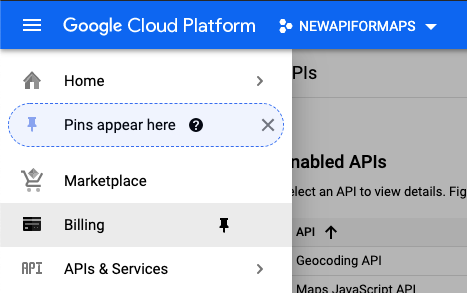
Please check out their official documentation for more information.
Address Block
Set up the 'Address Question' block, here's how.
Encode Address
Next, you'll need to encode the address in order to send the request to Google Maps, you'll add a 'Code block' with the following snippet:
window.uriaddress = encodeURIComponent("@{address}");
this.setCustomData({ newvar: uriaddress});
console.log(uriaddress);
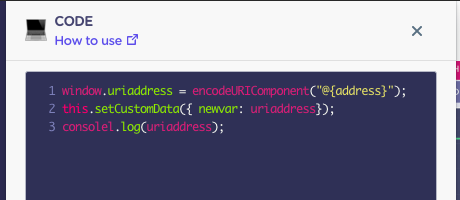
User Input
In order to make use of the variable we created in the previous step we need a user input. This can be any question type, but here's what we used:
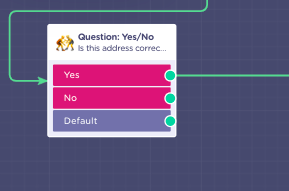
API Key
Add your API key again.
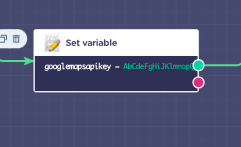
Webhook Request
Now we'll do a webhook request with our encoded address, you'll need to connect the previous block to a 'Webhook block' and add the following in the input section on top, selecting 'Get':
https://maps.googleapis.com/maps/api/geocode/json?address=@{newvar}&key=@{googlemapsapikey}
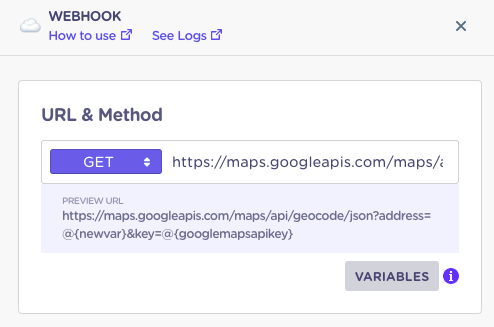
You can then test your request and save 'Results' as @response.
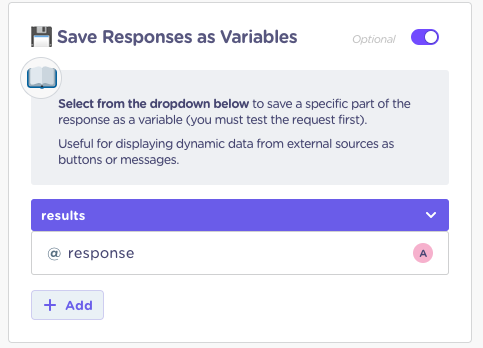
You can connect the red flow to an error message, or back to the address questions block, and then connect the green flow to the following:
Set Our Latitude and Longitude
We need to set our own latitude and longitude, we'll save them at @lat1 and @lon1, we'll connect these set variables to the code block:
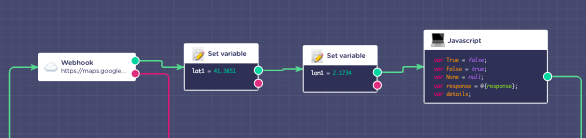
Code Block
Next, you'll need another 'Code block', in this block we will set the variables as well as calculate the distances
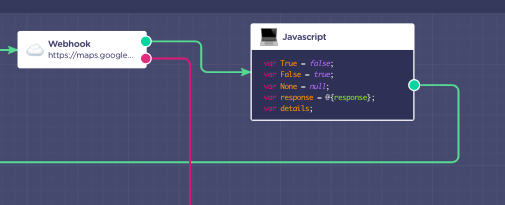
In this block you'll add the following snippet:
var True = false;
var False = true;
var None = null;
var response = @{response};
var details;
console.log(response);
if (response[0].hasOwnProperty('property1partial_match') && response[0].partial_match === True){
details = "partial match";
console.log("partial match");
} else if (response[0].types[0] == "postal_code" || response[0].partial_match === False || response[0].partial_match === undefined ){
details = response[0].address_components
console.log(details);
} else {
details = "no results";
console.log("no results");
}
console.log("details:",response);
var details_Address_Obj = {}
if (details !== "no results" && details !== "partial match"){
for (var i = 0; i < details.length; i++){
details[i][details[i].types[0]] = details[i]["long_name"]
details_Address_Obj[details[i].types[0]] = details[i][details[i].types[0]]
}
details_Address_Obj["formatted_address"] = response[0]["formatted_address"]
details_Address_Obj["latitude"] = response[0].geometry.location.lat
details_Address_Obj["longitude"] = response[0].geometry.location.lng
console.log(details_Address_Obj);
this.setCustomData( {
address_results: "Ok",
address_administrative_area: details_Address_Obj.administrative_area_level_1 || " ",
address_country: details_Address_Obj.country || " ",
address_formatted_address: details_Address_Obj.formatted_address || " ",
address_latitude: details_Address_Obj.latitude || " ",
address_locality: details_Address_Obj.locality || details_Address_Obj.postal_town || details_Address_Obj.administrative_area_level_2,
address_longitude: details_Address_Obj.longitude || " ",
address_sublocality: details_Address_Obj.political || " ",
address_postal_code: details_Address_Obj.postal_code,
address_route: details_Address_Obj.route || " ",
address_street_number: details_Address_Obj.street_number || " "
});
} else if (details === "no results"){
this.setCustomData ({
address_results: "Fail";
})
} else if (details === "partial match") {
this.setCustomData( {
address_results: "Partial";
})
}
//This function takes in latitude and longitude of two location and returns the distance between them as the crow flies (in km)
let lat1 = @{lat1};
let lon1 = @{lon1};
let lat2 = details_Address_Obj.latitude;
let lon2 = details_Address_Obj.longitude;
let distance;
function calcCrow(lat1, lon1, lat2, lon2)
{
console.log(lat1, lon1, lat2, lon2) ;
var R = 6371; // km;
var dLat = toRad(lat2-lat1);
var dLon = toRad(lon2-lon1);
var lat1 = toRad(lat1);
var lat2 = toRad(lat2);
var a = Math.sin(dLat/2) * Math.sin(dLat/2) +
Math.sin(dLon/2) * Math.sin(dLon/2) * Math.cos(lat1) * Math.cos(lat2);
var c = 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1-a));
var d = R * c;
distance = d.toFixed(1);
}
// Converts numeric degrees to radians
function toRad(Value)
{
return Value * Math.PI / 180;
}
calcCrow("@{lat1}", "@{lon1}", "@{lat2}", "@{lon2}");
this.setCustomData({distance:distance});
User Input
After every code block we need a user input before the variables can be used within the bot, here's what we used:
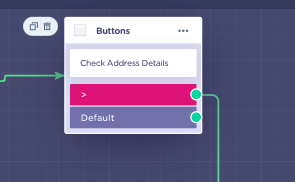
Add Condition
We then set a 'Condition' using one of the variables from the Javascript block, we'll connect the green arrow to the next point in your flow and the red to an error message or the address block, up to you!
If this condition is true then your flow will continue. Remember the conditions are case-sensitive so it's important to input exactly as it is below:
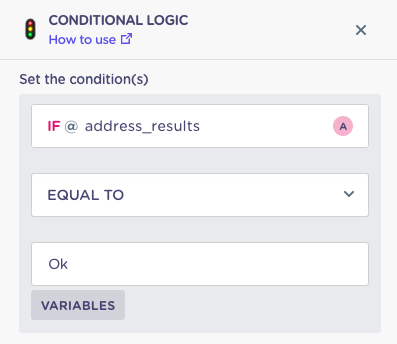
Code Block
After we've set our variables we'll connect them to a code block where we'll calculate the distance and create a new Landbot variable
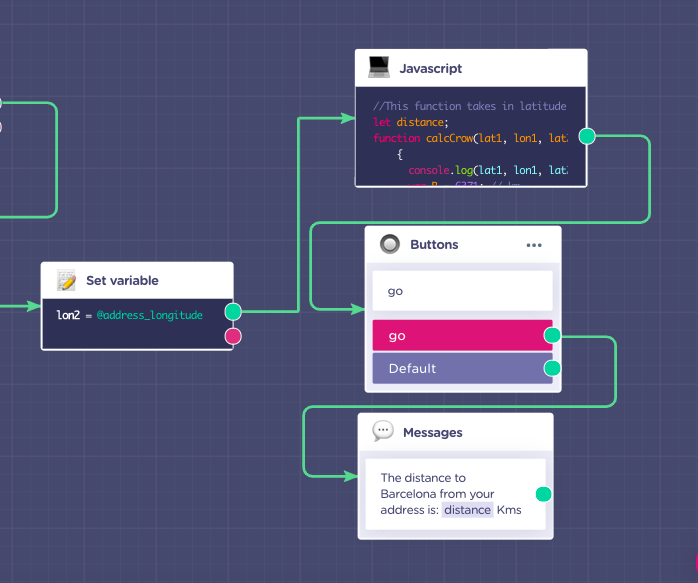
Here's what we need to put inside of the code block:
//This function takes in latitude and longitude of two location and returns the distance between them as the crow flies (in km)
let distance;
function calcCrow(lat1, lon1, lat2, lon2)
{
console.log(lat1, lon1, lat2, lon2)
var R = 6371; // km
var dLat = toRad(lat2-lat1);
var dLon = toRad(lon2-lon1);
var lat1 = toRad(lat1);
var lat2 = toRad(lat2);
var a = Math.sin(dLat/2) * Math.sin(dLat/2) +
Math.sin(dLon/2) * Math.sin(dLon/2) * Math.cos(lat1) * Math.cos(lat2);
var c = 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1-a));
var d = R * c;
distance = d.toFixed(1);
}
// Converts numeric degrees to radians
function toRad(Value)
{
return Value * Math.PI / 180;
}
calcCrow("@{lat1}", "@{lon1}", "@{lat2}", "@{lon2}")
this.setCustomData({distance:distance});
User Input
Remember to create a Landbot variable with Javascript we need a user input after the code block, which is why we add the button with only one option.
That's it! Now your distance will variable will be available in Landbot with the name @distance.
Here's what the results look like:
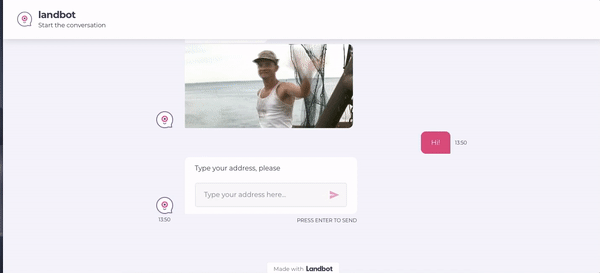